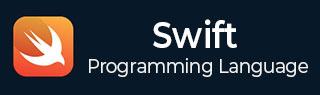
- Swift Tutorial
- Swift - Home
- Swift - Overview
- Swift - Environment
- Swift - Basic Syntax
- Swift - Variables
- Swift - Constants
- Swift - Literals
- Swift - Comments
- Swift Operators
- Swift - Operators
- Swift - Arithmetic Operators
- Swift - Comparison Operators
- Swift - Logical Operators
- Swift - Assignment Operators
- Swift - Bitwise Operators
- Swift - Misc Operators
- Swift Advanced Operators
- Swift - Operator Overloading
- Swift - Arithmetic Overflow Operators
- Swift - Identity Operators
- Swift - Range Operators
- Swift Data Types
- Swift - Data Types
- Swift - Integers
- Swift - Floating-Point Numbers
- Swift - Double
- Swift - Boolean
- Swift - Strings
- Swift - Characters
- Swift - Type Aliases
- Swift - Optionals
- Swift - Tuples
- Swift - Assertions and Precondition
- Swift Control Flow
- Swift - Decision Making
- Swift - if statement
- Swift - if...else if...else Statement
- Swift - if-else Statement
- Swift - nested if statements
- Swift - switch statement
- Swift - Loops
- Swift - for in loop
- Swift - While loop
- Swift - repeat...while loop
- Swift - continue statement
- Swift - break statement
- Swift - fall through statement
- Swift Collections
- Swift - Arrays
- Swift - Sets
- Swift - Dictionaries
- Swift Functions
- Swift - Functions
- Swift - Nested Functions
- Swift - Function Overloading
- Swift - Recursion
- Swift - Higher-Order Functions
- Swift Closures
- Swift - Closures
- Swift-Escaping and Non-escaping closure
- Swift - Auto Closures
- Swift OOps
- Swift - Enumerations
- Swift - Structures
- Swift - Classes
- Swift - Properties
- Swift - Methods
- Swift - Subscripts
- Swift - Inheritance
- Swift-Overriding
- Swift - Initialization
- Swift - Deinitialization
- Swift Advanced
- Swift - ARC Overview
- Swift - Optional Chaining
- Swift - Error handling
- Swift - Concurrency
- Swift - Type Casting
- Swift - Nested Types
- Swift - Extensions
- Swift - Protocols
- Swift - Generics
- Swift - Access Control
- Swift - Function vs Method
- Swift - SwiftyJSON
- Swift - Singleton class
- Swift Random Numbers
- Swift Opaque and Boxed Type
- Swift Useful Resources
- Swift - Compile Online
- Swift - Quick Guide
- Swift - Useful Resources
- Swift - Discussion
Swift - Type Aliases
Swift provides a special feature named Type Aliases. Type aliases are used to define another name or aliases for the pre-defined types. It only gives a new name to the existing type and does not create a new type. For example, "Int" can also be defined as "myInt".
After defining type alias, we can use that alias anywhere in the program. A single program can contain more than one alias. A type alias is commonly used to improve code readability, code quality and abstracting complex types. We can define type aliases with the help of the type alias keyword.
Syntax
Following is the syntax of the type alias –
typealiase newName = existingType
We can define type aliases for the following data types −
Primitive Data Types
User Defined Data Types
Complex Data Types
Type Aliases for Primitive Data Types in Swift
Primitive data types are the pre-defined data types provided by Swift such as Int, Float, Double, Character and String. With the help of a type alias, we can provide a new name to the in-built data types which can be used throughout the program without any error. For example, "typealias myString = String" now using myString we can create a string type variable.
Syntax
Following is the syntax of the type alias for primitive data types −
typealias newName = PreDefinedDataType
Here the PreDefinedDataType can be Int, Float, Double, String and Character.
Example
Swift program to create a type alias for in-built data types.
import Foundation // Creating type typealias for String typealias myString = String // Creating type typealias for Float typealias myNum = Float // Creating type typealias for Int typealias Num = Int // Declaring integer type variable using Num var number : Num = 10 // Declaring string type variable using myString var newString : myString = "Tutorialspoint" // Declaring float type variable using myNum var value : myNum = 23.456 print("Number:", number) print("type:", type(of: number)) print("\nString:", newString) print("type:", type(of: newString)) print("\nFloat:", value) print("type:", type(of: value))
Output
Number: 10 type: Int String: Tutorialspoint type: String Float: 23.456 type: Float
Type Aliases for User- Defined Data Types in Swift
Using type aliases, we can also provide an alternate name to the user-defined data types. For example, "typealias mySet = Set<String>" now using mySet we can create a set of string type.
Syntax
Following is the syntax of the type alias for user-defined data types −
typealias newName = dataType
Here the datatype can be Array, Set, dictionary, etc.
Example
Swift program to create a type alias for user-defined data types.
import Foundation // Creating type typealias for Set typealias mySet = Set<Int> // Creating type typealias for Array typealias myNum = Array<Int> // Creating type typealias for Array typealias Num = Array<String> // Declaring set of integer type using mySet var newSet : mySet = [32, 3, 1, 2] // Declaring array of integer type using myNum var newArray : myNum = [32, 2, 1, 1, 3] // Declaring array of string type using Num var newArr : Num = ["Swift", "C++", "C#"] print("Set:", newSet) print("Array:", newArray) print("Array:", newArr)
Output
Set: [32, 3, 1, 2] Array: [32, 2, 1, 1, 3] Array: ["Swift", "C++", "C#"]
Type Aliases for Complex Data Types in Swift
Complex data are the special type of data types which contain more than one pre-defined data type. So using types aliases we can also create aliases of complex data types. For example, (String, String) -> string as "MyString".
Syntax
Following is the syntax of type aliases for complex data types −
typealias newName = CdataType
Here the CdataType can be any complex data type like (String)->(String).
Example
Swift program to create a type alias for complex data types.
import Foundation // Creating type typealias for function type typealias Value = (String, String) -> String func addStr(s1: String, s2: String) -> String{ return s1 + s2 } // Assigning addStr function to Value var newFunc : Value = addStr // Calling function var result = newFunc("Hello", "World") print(result)
Output
HelloWorld