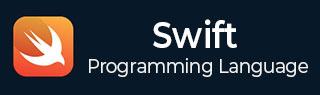
- Swift Tutorial
- Swift - Home
- Swift - Overview
- Swift - Environment
- Swift - Basic Syntax
- Swift - Variables
- Swift - Constants
- Swift - Literals
- Swift - Comments
- Swift Operators
- Swift - Operators
- Swift - Arithmetic Operators
- Swift - Comparison Operators
- Swift - Logical Operators
- Swift - Assignment Operators
- Swift - Bitwise Operators
- Swift - Misc Operators
- Swift Advanced Operators
- Swift - Operator Overloading
- Swift - Arithmetic Overflow Operators
- Swift - Identity Operators
- Swift - Range Operators
- Swift Data Types
- Swift - Data Types
- Swift - Integers
- Swift - Floating-Point Numbers
- Swift - Double
- Swift - Boolean
- Swift - Strings
- Swift - Characters
- Swift - Type Aliases
- Swift - Optionals
- Swift - Tuples
- Swift - Assertions and Precondition
- Swift Control Flow
- Swift - Decision Making
- Swift - if statement
- Swift - if...else if...else Statement
- Swift - if-else Statement
- Swift - nested if statements
- Swift - switch statement
- Swift - Loops
- Swift - for in loop
- Swift - While loop
- Swift - repeat...while loop
- Swift - continue statement
- Swift - break statement
- Swift - fall through statement
- Swift Collections
- Swift - Arrays
- Swift - Sets
- Swift - Dictionaries
- Swift Functions
- Swift - Functions
- Swift - Nested Functions
- Swift - Function Overloading
- Swift - Recursion
- Swift - Higher-Order Functions
- Swift Closures
- Swift - Closures
- Swift-Escaping and Non-escaping closure
- Swift - Auto Closures
- Swift OOps
- Swift - Enumerations
- Swift - Structures
- Swift - Classes
- Swift - Properties
- Swift - Methods
- Swift - Subscripts
- Swift - Inheritance
- Swift-Overriding
- Swift - Initialization
- Swift - Deinitialization
- Swift Advanced
- Swift - ARC Overview
- Swift - Optional Chaining
- Swift - Error handling
- Swift - Concurrency
- Swift - Type Casting
- Swift - Nested Types
- Swift - Extensions
- Swift - Protocols
- Swift - Generics
- Swift - Access Control
- Swift - Function vs Method
- Swift - SwiftyJSON
- Swift - Singleton class
- Swift Random Numbers
- Swift Opaque and Boxed Type
- Swift Useful Resources
- Swift - Compile Online
- Swift - Quick Guide
- Swift - Useful Resources
- Swift - Discussion
Swift - Random Numbers
Random Numbers are the sequence of numbers that are generated by the system without any pre-defined pattern or in an unpredictable manner. They are commonly used in chance events, produce unpredictable outcomes, etc. Random numbers are widely used in cryptography, gaming, statistical analysis, generating unique id/sessions for users, etc.
In Swift, we can find random numbers using the following methods −
- random(in:) function
- random(in: using:) function
- randomElement() function
Using random(in:) Function
We can find random numbers with the help of the random(in:) function. This function returns a random number from the given range. Using this function, we can generate random numbers of Int, Double, Float and Bool types.
Syntax
Following is the syntax of the random(in:) function −
static func random(in: inputRange)
Here this function takes only one parameter which is the inputRange. The inputRange represents a range in which a random() function creates a random number.
Return Value
This function returns a random number from the given range.
Example
Swift program to generate random numbers using random(in:) function.
import Foundation // Generating a random number of Int type var randomNumber1 = Int.random(in: 10...23) print("Random Number:", randomNumber1) // Generating a random number of Float type var randomNumber2 = Float.random(in: 10.2...23.2) print("Random Number:", randomNumber2) // Generating a random number of Double type var randomNumber3 = Double.random(in: 15.2..<25.2) print("Random Number:", randomNumber3) // Generating a random number of Bool type var randomNumber4 = Bool.random() print("Random Number:", randomNumber4)
Output
It will produce the following output −
Random Number: 20 Random Number: 14.4035845 Random Number: 17.450544998301993 Random Number: true
Using random(in:using:) Function
To generate random numbers Swift supports a pre-defined function named random(in:using:) function. This function generates a random number within the given range with the help of a given generator. It also generates random numbers of Int, Double, Float and Bool types.
Syntax
Following is the syntax of the random(in:using:) function −
static func random(in: inputRange, using: generator)
This function takes two parameters −
inputRange − It represents a range. Its value must not be empty.
generator − It represents the generator using which random(in:using:) function will generate a random number.
Return Value
This function returns a random number within the specified range.
Example
Swift program to generate random numbers using random(in:using:) function.
import Foundation // Specifying a generator var myGenerator = SystemRandomNumberGenerator() // Generating a random number of Int type var randomNumber1 = Int.random(in: 30...43, using: &myGenerator) print("Random Number:", randomNumber1) // Generating a random number of Float type var randomNumber2 = Float.random(in: 12.2...33.2, using: &myGenerator) print("Random Number:", randomNumber2) // Generating a random number of Double type var randomNumber3 = Double.random(in: 35.2..<45.2, using: &myGenerator) print("Random Number:", randomNumber3) // Generating a random number of Bool type var randomNumber4 = Bool.random(using: &myGenerator) print("Random Number:", randomNumber4)
Output
It will produce the following output −
Random Number: 34 Random Number: 20.267605 Random Number: 42.47363754282583 Random Number: false
Using randomElement() Function
When we are working with collections such as an array and dictionary, we can use the randomElement() function. This function returns a random element from the given collection. The value returned by this function is an optional type so we must unwrap it using (!).
Syntax
Following is the syntax of the randomElement() function −
static func randomElement()
Return Value
This function returns a random number from the specified collection. If the collection is empty, then it will return nil.
Example
Swift program to generate a random element from the given collection.
import Foundation let myVeggies = ["Lemon", "Cabbage", "Green Chilly", "Potato"] // Getting random element let randomVeggie = myVeggies.randomElement()! print("Random Element: \(randomVeggie)")
Output
It will produce the following output −
Random Element: Lemon