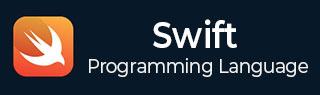
- Swift Tutorial
- Swift - Home
- Swift - Overview
- Swift - Environment
- Swift - Basic Syntax
- Swift - Variables
- Swift - Constants
- Swift - Literals
- Swift - Comments
- Swift Operators
- Swift - Operators
- Swift - Arithmetic Operators
- Swift - Comparison Operators
- Swift - Logical Operators
- Swift - Assignment Operators
- Swift - Bitwise Operators
- Swift - Misc Operators
- Swift Advanced Operators
- Swift - Operator Overloading
- Swift - Arithmetic Overflow Operators
- Swift - Identity Operators
- Swift - Range Operators
- Swift Data Types
- Swift - Data Types
- Swift - Integers
- Swift - Floating-Point Numbers
- Swift - Double
- Swift - Boolean
- Swift - Strings
- Swift - Characters
- Swift - Type Aliases
- Swift - Optionals
- Swift - Tuples
- Swift - Assertions and Precondition
- Swift Control Flow
- Swift - Decision Making
- Swift - if statement
- Swift - if...else if...else Statement
- Swift - if-else Statement
- Swift - nested if statements
- Swift - switch statement
- Swift - Loops
- Swift - for in loop
- Swift - While loop
- Swift - repeat...while loop
- Swift - continue statement
- Swift - break statement
- Swift - fall through statement
- Swift Collections
- Swift - Arrays
- Swift - Sets
- Swift - Dictionaries
- Swift Functions
- Swift - Functions
- Swift - Nested Functions
- Swift - Function Overloading
- Swift - Recursion
- Swift - Higher-Order Functions
- Swift Closures
- Swift - Closures
- Swift-Escaping and Non-escaping closure
- Swift - Auto Closures
- Swift OOps
- Swift - Enumerations
- Swift - Structures
- Swift - Classes
- Swift - Properties
- Swift - Methods
- Swift - Subscripts
- Swift - Inheritance
- Swift-Overriding
- Swift - Initialization
- Swift - Deinitialization
- Swift Advanced
- Swift - ARC Overview
- Swift - Optional Chaining
- Swift - Error handling
- Swift - Concurrency
- Swift - Type Casting
- Swift - Nested Types
- Swift - Extensions
- Swift - Protocols
- Swift - Generics
- Swift - Access Control
- Swift - Function vs Method
- Swift - SwiftyJSON
- Swift - Singleton class
- Swift Random Numbers
- Swift Opaque and Boxed Type
- Swift Useful Resources
- Swift - Compile Online
- Swift - Quick Guide
- Swift - Useful Resources
- Swift - Discussion
Swift - Operator Overloading
Operator Overloading in Swift
Operator overloading is a powerful technique in Swift programming. Operator overloading allows us to change the working of the existing operators like +, -, /, *, %, etc. with the customized code.
It makes code more expressive and readable. To overload an operator, we have to define the behaviour of that operator using the "static func" keyword.
Syntax
Following is the syntax for operator overloading −
static func operatorName() { // body }
Example 1
Swift program to overload + operator to calculate the sum of two complex numbers.
import Foundation struct ComplexNumber { var real: Double var imag: Double // Overloading + operator to add two complex numbers static func+(left: ComplexNumber, right: ComplexNumber) -> ComplexNumber { return ComplexNumber(real: left.real + right.real, imag: left.imag + right.imag) } } let complexNumber1 = ComplexNumber(real: 2.1, imag: 2.0) let complexNumber2 = ComplexNumber(real: 6.1, imag: 9.0) // Calling + operator to add two complex numbers let sumOfComplexNumbers = complexNumber1 + complexNumber2 print(sumOfComplexNumbers)
Output
ComplexNumber(real: 8.2, imag: 11.0)
Example 2
Swift program to overload custom prefix operator.
import Foundation struct Car { var price: Double // Overloading the custom prefix operator "++" to increase the price of car static prefix func ++ (carPrice: inout Car) { carPrice.price += 500000.0 } } var currentPrice = Car(price: 2500000.0) // Calling the custom ++ operator to increase the car price ++currentPrice print("Updated car price is", currentPrice.price)
Output
Updated car price is 2500000.0
Limitation of Operator Overloading in Swift
The following are the limitations of operator overloading −
- In Swift, you can overload limited operators like arithmetic and customized operators.
- While overloading operators, you are not allowed to change the precedence or associativity of the operators.
- Swift does not support short-circuiting behaviour for overloading logical operators.
- Do not overuse operator overloading because it makes your code difficult to read and understand.
Difference Between Operator Function and Normal Functions
The following are the major differences between the operator functions and normal functions −
Operator Function | Normal Function |
---|---|
They are define using "static func" keyword and a custom operator symbol. | They are defined using "func" keyword and the function name. |
They are used to customize the behaviours of operators. | They are used to complete general purpose tasks. |
They are called implicitly when using operator with custom types. | They are called explicitly with the help of function name. |
They can be defined in index, prefix or postfix form. | They can only defined in infix form. |
Advertisements