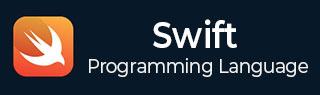
- Swift Tutorial
- Swift - Home
- Swift - Overview
- Swift - Environment
- Swift - Basic Syntax
- Swift - Variables
- Swift - Constants
- Swift - Literals
- Swift - Comments
- Swift Operators
- Swift - Operators
- Swift - Arithmetic Operators
- Swift - Comparison Operators
- Swift - Logical Operators
- Swift - Assignment Operators
- Swift - Bitwise Operators
- Swift - Misc Operators
- Swift Advanced Operators
- Swift - Operator Overloading
- Swift - Arithmetic Overflow Operators
- Swift - Identity Operators
- Swift - Range Operators
- Swift Data Types
- Swift - Data Types
- Swift - Integers
- Swift - Floating-Point Numbers
- Swift - Double
- Swift - Boolean
- Swift - Strings
- Swift - Characters
- Swift - Type Aliases
- Swift - Optionals
- Swift - Tuples
- Swift - Assertions and Precondition
- Swift Control Flow
- Swift - Decision Making
- Swift - if statement
- Swift - if...else if...else Statement
- Swift - if-else Statement
- Swift - nested if statements
- Swift - switch statement
- Swift - Loops
- Swift - for in loop
- Swift - While loop
- Swift - repeat...while loop
- Swift - continue statement
- Swift - break statement
- Swift - fall through statement
- Swift Collections
- Swift - Arrays
- Swift - Sets
- Swift - Dictionaries
- Swift Functions
- Swift - Functions
- Swift - Nested Functions
- Swift - Function Overloading
- Swift - Recursion
- Swift - Higher-Order Functions
- Swift Closures
- Swift - Closures
- Swift-Escaping and Non-escaping closure
- Swift - Auto Closures
- Swift OOps
- Swift - Enumerations
- Swift - Structures
- Swift - Classes
- Swift - Properties
- Swift - Methods
- Swift - Subscripts
- Swift - Inheritance
- Swift-Overriding
- Swift - Initialization
- Swift - Deinitialization
- Swift Advanced
- Swift - ARC Overview
- Swift - Optional Chaining
- Swift - Error handling
- Swift - Concurrency
- Swift - Type Casting
- Swift - Nested Types
- Swift - Extensions
- Swift - Protocols
- Swift - Generics
- Swift - Access Control
- Swift - Function vs Method
- Swift - SwiftyJSON
- Swift - Singleton class
- Swift Random Numbers
- Swift Opaque and Boxed Type
- Swift Useful Resources
- Swift - Compile Online
- Swift - Quick Guide
- Swift - Useful Resources
- Swift - Discussion
Swift - Methods
Methods are the functions of a particular type, such as class, structure or enumeration. They define the behavior and functionality for instances of a type. They are generally accessed by the instances. Swift also supports type methods that are associated with the type itself.
Instance Methods in Swift
In Swift, instance methods are the functions that belong to the specific instance of a class, structure or enumeration. They are called on an instance of the type and can access and modify instance properties or other instance methods, or also add functionality related to the instance's need.
The syntax of the instance method is the same as the function. Instance method can also have, parameters, return type, parameter names, and argument labels. It can be written inside the {} curly braces. It has implicit access to methods and properties of the type instance. An instance method is called only using the instance of that particular type, we are not allowed to call an instance method without any instance.
Syntax
Following is the syntax of the instance method-
func methodname(Parameters) -> returntype { Statement1 Statement2 --- Statement N return parameters }
Instance methods are accessed with '.' dot syntax.
instanceName.methodName()
Example
// Defining a class class Calculations { // Properties let a: Int let b: Int let res: Int // Initializer init(a: Int, b: Int) { self.a = a self.b = b res = a + b } // Instance method func tot(c: Int) -> Int { return res - c } // Instance method func result() { print("Result is: \(tot(c: 20))") print("Result is: \(tot(c: 50))") } } // Creating and initializing the instance of the class let pri = Calculations(a: 600, b: 300) // Accessing the instance method pri.result()
Output
It will produce the following output −
Result is: 880 Result is: 850
Mutating Instance Method
In Swift, structures and enumerations are value types, which means we cannot alter the properties inside the instance method. So to modify the properties inside a method we need to specify that method as a mutating method using the mutating keyword and the changes made by the method in its properties will written back to the original structure when the method ends.
We can only mutate methods of structure and enumeration but not class because a class is a reference type so their properties can be modified without using mutating keyword. Also, we cannot call a mutating method on a constant of structure type.
Syntax
Following is the syntax of the mutating instance method −
mutating func methodname(Parameters) -> returntype { Statement }
Example
Swift program to demonstrate the mutating method in structure.
// Defining a structure struct CalculateSum { // Properties var num1 = 1 var num2 = 1 // Instance method func sum() -> Int { return num1 + num2 } // Mutating instance method mutating func increment(res: Int) { num1 *= res num2 *= res print("New Number 1 is ", num1) print("New Number 2 is ", num2) } } // Creating and initializing the instance of structure var obj = CalculateSum(num1: 10, num2: 12) // Calling mutating method obj.increment(res: 10) // Calling instance method print("Sum is ", obj.sum())
Output
It will produce the following output −
New Number 1 is 100 New Number 2 is 120 Sum is 220
Assigning Instance to self within a Mutating Method
In mutating methods, we are allowed to assign a new instance to the implicit self property. It will replace the existing instance with the new instance. While assigning a new instance to the self always be careful because it changes the state of the instance and any reference related to the original instance will not reflect the changes.
Example
Swift program to demonstrate how to assign an instance to self within a mutating method.
// Defining a structure struct Student { var age: Int var name: String // Mutating method that assigns a new instance to self mutating func newValue() { self = Student(age: 23, name: "Jyoti") } } // Creating an instance of the Student structure var obj = Student(age: 22, name: "Poonam") // Calling the mutating method obj.newValue() // Displaying the updated values print("New age: \(obj.age), New name: \(obj.name)")
Output
It will produce the following output −
New age: 23, New name: Jyoti
Method Argument label and Parameter name in Swift
Just like a function, a method can also have labels and names for its arguments and parameters. These labels and names provide a clear and descriptive context for the argument and parameter. The parameter name is used in the declaration of the method whereas the argument label is used when the method is called. By default, the argument label and parameter name are the same, but multiple parameters can have the same or unique argument label. The names of the parameters should be unique so that the compiler can easily distinguish them. We can also ignore the argument label by placing the underscore(_) before the name of the parameter.
Syntax
Following is the syntax for the argument label and parameter name −
// Parameter name With argument label func methodname(argumentLabel parameterName: Type, parameterName: Type) -> returntype { Statement return value } methodname(name1:value, name2: value) // Parameter name without argument label func methodname(_name1: Type, _name2: Type) -> returntype { Statement return value } methodname(value1, value2)
Example
Swift program to demonstrate how to specify the parameter name and argument label in the method.
// Defining class class CalculateSum { // Method with parameter name and argument label func addingTwoNumbers(num1 x: Int, num2 y: Int) -> Int { return x + y } // Method without argument label func addingThreeNumbers(_ x: Int, _ y: Int, num1 z: Int) -> Int { return x + y + z } } // Creating an instance of CalculateSum class let obj = CalculateSum() // Calling the method with parameter name and argument label let result1 = obj.addingTwoNumbers(num1: 10, num2: 20) // Calling the method without argument label let result2 = obj.addingThreeNumbers(20, 30, num1: 40) print("Sum of two numbers:", result1) print("Sum of three numbers:", result2)
Output
It will produce the following output −
Sum of two numbers: 30 Sum of three numbers: 90
The self property in Methods
The self keyword is used to distinguish between the property name and the method’s parameters. The self keyword is generally used with a property inside the instance methods, mutating methods, or initializer so that the compiler can easily discriminate which one is a parameter and a property if their names are the same. Or the self keyword is used to refer to the current instance for its defined methods. We can use the self keyword in structure, classes, and enumerations.
Syntax
Following is the syntax of the self keyword −
self.PropertyName
Example
Swift program to demonstrate how to use self keyword.
// Defining class class Student { var name: String // Initializer init(name: String) { self.name = name } // Instance method using self func greet(name: String) { self.name = name print("Hello, my name is \(self.name).") } } // Creating an instance of the Student class var obj = Student(name: "Mona") // Calling the greet method obj.greet(name: "Mohina")
Output
It will produce the following output −
Hello, my name is Mohina.
Type Methods
Swift supports a special type of methods that are known as Type methods. Type methods are called on the type itself, not by the instance of the type. These methods are represented by a static keyword in Structure and Enumeration whereas a class keyword in Classes. In classes, the type method allows a subclass to override the superclass’s implementation of the type method. The type method is accessed by using dot(.) notation.
In the type method, the property will refer to the type itself, not the instance of the type, which means we can distinguish between type property and type method parameters. A type method is allowed to call another type method within the other method’s name without using any prefix type name. Similarly in structure and enumeration, a type method can access the type property by using the name of the property without any prefix type.
Syntax
Following is the syntax of the type method in structure and enumeration −
static func methodname(Parameters) -> returntype { Statement }
Following is the syntax of the type method in class −
class ClassName{ class func methodname(Parameters) -> returntype { Statement } }
Following is the syntax of the accessing type method −
type.typeMethodName()
Example
Swift program to demonstrate how to create and access type methods in structure and class.
// Defining a structure struct AreaOfRectangle { // Type method using static keyword static func area(_ length: Int, _ breadth: Int) -> Int { return length * breadth } } // Defining a class class Area { // Type method using class keyword class func areaOfTriangle(base: Double, height: Double) -> Double { return (1 * base * height)/2 } } // Accessing type method of AreaOfRectangle structure let result1 = AreaOfRectangle.area(12, 10) print("Area of rectangle is ", result1) // Accessing type method of Area class let result2 = Area.areaOfTriangle(base: 5, height: 15) print("Area of triangle is ", result2)
Output
It will produce the following output −
Area of rectangle is 120 Area of triangle is 37.5
Example
Swift program to demonstrate how a type method calls another type method and type property.
// Defining a structure struct Student { // Type Property static let name = "Mohina" static let totalMarks = 300 // Type method to add marks of three major subjects static func add(_ Maths: Int, _ Chemistry: Int, _ Physics: Int) -> Int { return Maths + Physics + Chemistry } // Type method to find the percentage of three subjects marks by calling another type method static func percentage() -> Double { // Calling another type of method let finalMarks = Double(add(70, 80, 90)) // Accessing type property let percentage = ((finalMarks / Double(totalMarks)) * 100) return percentage } } // Accessing a type property print("Name of the student: \(Student.name)") // Accessing a type method print("Percentage: ", Student.percentage())
Output
It will produce the following output −
Name of the student: Mohina Percentage: 80.0