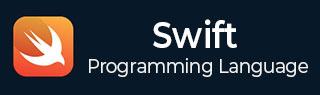
- Swift Tutorial
- Swift - Home
- Swift - Overview
- Swift - Environment
- Swift - Basic Syntax
- Swift - Variables
- Swift - Constants
- Swift - Literals
- Swift - Comments
- Swift Operators
- Swift - Operators
- Swift - Arithmetic Operators
- Swift - Comparison Operators
- Swift - Logical Operators
- Swift - Assignment Operators
- Swift - Bitwise Operators
- Swift - Misc Operators
- Swift Advanced Operators
- Swift - Operator Overloading
- Swift - Arithmetic Overflow Operators
- Swift - Identity Operators
- Swift - Range Operators
- Swift Data Types
- Swift - Data Types
- Swift - Integers
- Swift - Floating-Point Numbers
- Swift - Double
- Swift - Boolean
- Swift - Strings
- Swift - Characters
- Swift - Type Aliases
- Swift - Optionals
- Swift - Tuples
- Swift - Assertions and Precondition
- Swift Control Flow
- Swift - Decision Making
- Swift - if statement
- Swift - if...else if...else Statement
- Swift - if-else Statement
- Swift - nested if statements
- Swift - switch statement
- Swift - Loops
- Swift - for in loop
- Swift - While loop
- Swift - repeat...while loop
- Swift - continue statement
- Swift - break statement
- Swift - fall through statement
- Swift Collections
- Swift - Arrays
- Swift - Sets
- Swift - Dictionaries
- Swift Functions
- Swift - Functions
- Swift - Nested Functions
- Swift - Function Overloading
- Swift - Recursion
- Swift - Higher-Order Functions
- Swift Closures
- Swift - Closures
- Swift-Escaping and Non-escaping closure
- Swift - Auto Closures
- Swift OOps
- Swift - Enumerations
- Swift - Structures
- Swift - Classes
- Swift - Properties
- Swift - Methods
- Swift - Subscripts
- Swift - Inheritance
- Swift-Overriding
- Swift - Initialization
- Swift - Deinitialization
- Swift Advanced
- Swift - ARC Overview
- Swift - Optional Chaining
- Swift - Error handling
- Swift - Concurrency
- Swift - Type Casting
- Swift - Nested Types
- Swift - Extensions
- Swift - Protocols
- Swift - Generics
- Swift - Access Control
- Swift - Function vs Method
- Swift - SwiftyJSON
- Swift - Singleton class
- Swift Random Numbers
- Swift Opaque and Boxed Type
- Swift Useful Resources
- Swift - Compile Online
- Swift - Quick Guide
- Swift - Useful Resources
- Swift - Discussion
Swift - If Statement
An if statement consists of a Boolean expression followed by a block of statements. When the boolean expression is true, then only the block of statements will execute. Otherwise, the controls move to the next statement present just after the if statement block. They are also known as branch statements because they allow the program to take different paths according to the given condition.
For example, a teacher says to its students that “they are only allowed to write with a black pencil”. So here the conditional statement is “black pencil”. Hence If the “black pencil = true”, then only students are allowed to write.
Syntax
Following is the syntax of the if statement −
if boolean_expression{ /* statement(s) will execute if the boolean expression is true */ }
If the Boolean expression evaluates to true, then the block of code inside the if statement will be executed. If the Boolean expression evaluates to false, then the first set of code after the end of the if statement (after the closing curly brace) will be executed.
Flow Diagram
The following flow diagram will show how if-statement works −

Example
Swift program to check if the given number is less than 20 or not using the if statement.
import Foundation var varA:Int = 10; /* Check the boolean condition using the if statement */ if varA < 20 { /* If the condition is true then print the following */ print("varA is less than 20"); } print("Value of variable varA is \(varA)");
Output
It will produce the following output −
varA is less than 20 The value of variable varA is 10
Example
Swift program to find the age for voting using if statement.
import Foundation var age : Int = 19; /* Checking the age for voting */ if age >= 18 { /* If the condition is true */ print("Eligible for voting"); } print("A Candidate whose age is 18+ is eligible for voting ")
Output
It will produce the following output −
Eligible for voting A candidate whose age is 18+ is eligible for voting
Example
Swift program to check the entered username is equal to the stored username using if statement.
import Foundation let username = "input231" let inputUsername = "input231" // Checking for equality if username == inputUsername{ print("Login successful") } var result = 32 + 23 print(result)
Output
It will produce the following output −
Login successful 55