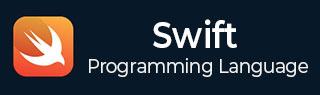
- Swift Tutorial
- Swift - Home
- Swift - Overview
- Swift - Environment
- Swift - Basic Syntax
- Swift - Variables
- Swift - Constants
- Swift - Literals
- Swift - Comments
- Swift Operators
- Swift - Operators
- Swift - Arithmetic Operators
- Swift - Comparison Operators
- Swift - Logical Operators
- Swift - Assignment Operators
- Swift - Bitwise Operators
- Swift - Misc Operators
- Swift Advanced Operators
- Swift - Operator Overloading
- Swift - Arithmetic Overflow Operators
- Swift - Identity Operators
- Swift - Range Operators
- Swift Data Types
- Swift - Data Types
- Swift - Integers
- Swift - Floating-Point Numbers
- Swift - Double
- Swift - Boolean
- Swift - Strings
- Swift - Characters
- Swift - Type Aliases
- Swift - Optionals
- Swift - Tuples
- Swift - Assertions and Precondition
- Swift Control Flow
- Swift - Decision Making
- Swift - if statement
- Swift - if...else if...else Statement
- Swift - if-else Statement
- Swift - nested if statements
- Swift - switch statement
- Swift - Loops
- Swift - for in loop
- Swift - While loop
- Swift - repeat...while loop
- Swift - continue statement
- Swift - break statement
- Swift - fall through statement
- Swift Collections
- Swift - Arrays
- Swift - Sets
- Swift - Dictionaries
- Swift Functions
- Swift - Functions
- Swift - Nested Functions
- Swift - Function Overloading
- Swift - Recursion
- Swift - Higher-Order Functions
- Swift Closures
- Swift - Closures
- Swift-Escaping and Non-escaping closure
- Swift - Auto Closures
- Swift OOps
- Swift - Enumerations
- Swift - Structures
- Swift - Classes
- Swift - Properties
- Swift - Methods
- Swift - Subscripts
- Swift - Inheritance
- Swift-Overriding
- Swift - Initialization
- Swift - Deinitialization
- Swift Advanced
- Swift - ARC Overview
- Swift - Optional Chaining
- Swift - Error handling
- Swift - Concurrency
- Swift - Type Casting
- Swift - Nested Types
- Swift - Extensions
- Swift - Protocols
- Swift - Generics
- Swift - Access Control
- Swift - Function vs Method
- Swift - SwiftyJSON
- Swift - Singleton class
- Swift Random Numbers
- Swift Opaque and Boxed Type
- Swift Useful Resources
- Swift - Compile Online
- Swift - Quick Guide
- Swift - Useful Resources
- Swift - Discussion
Swift - Identity Operators
Identity operators are used to check whether the given constants or variables refer to the same instance or not. These operators are generally used with objects or classes because they are reference types. These operators are different from equality operators.
Equality operators check if the two given values are equal to not whereas the identity operators check if the two given variables refer to the same reference or not. Swift supports two types of identity operators −
Operator | Name | Example |
---|---|---|
+=== | Identical to | Num1 === Num2 |
!== | Not Identical to | Num1 !== Num2 |
Identical to Operator in Swift
Identical to "===" operator is used to check whether the given two constants or variables refer to the same instance of the class or not. If yes, then this operator will return true.
Otherwise, this operator will return false. This operator compares the reference not the content of the objects.
Syntax
Following is the syntax of the Identical to operator −
myVariable1 === myVariable2
Example
Swift program to check if the given variables refer to the same object or not.
import Foundation // Class class TutorialsPoint { var empName: String init(empName: String) { self.empName = empName } } // Creating object of TutorialsPoint class let object1 = TutorialsPoint(empName: "Monika") let object2 = object1 // Checking if both variables refer to the same object or not // Using identical to (===) operator if object1 === object2 { print("YES! Both object1 and object2 are identical") } else { print("NO! Both object1 and object2 are not identical") }
Output
YES! Both object1 and object2 are identical
Not Identical to Operator in Swift
The Not Identical to "!==" operator is used to check whether the given variables do not refer to the same operator or not. If yes, then this operator will return true. Otherwise, it will return false.
Syntax
Following is the syntax of Not Identical to Operator −
myVariable1 !== myVariable2
Example
Swift program to check if two variables do not refer to the same object or not using not identical to "!==" operator.
import Foundation // Class class Author1 { var bookName: String init(bookName: String) { self.bookName = bookName } } class Author2 { var bookName: String init(bookName: String) { self.bookName = bookName } } // Creating objects let obj1 = Author1(bookName: "Tales of Mumbai") let obj2 = Author2(bookName: "Right to find") // Using not identical to (!==) operator if obj1 !== obj2 { print("They are identically not equal") } else { print("They are identically equal") }
Output
They are identically not equal