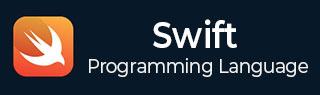
- Swift Tutorial
- Swift - Home
- Swift - Overview
- Swift - Environment
- Swift - Basic Syntax
- Swift - Variables
- Swift - Constants
- Swift - Literals
- Swift - Comments
- Swift Operators
- Swift - Operators
- Swift - Arithmetic Operators
- Swift - Comparison Operators
- Swift - Logical Operators
- Swift - Assignment Operators
- Swift - Bitwise Operators
- Swift - Misc Operators
- Swift Advanced Operators
- Swift - Operator Overloading
- Swift - Arithmetic Overflow Operators
- Swift - Identity Operators
- Swift - Range Operators
- Swift Data Types
- Swift - Data Types
- Swift - Integers
- Swift - Floating-Point Numbers
- Swift - Double
- Swift - Boolean
- Swift - Strings
- Swift - Characters
- Swift - Type Aliases
- Swift - Optionals
- Swift - Tuples
- Swift - Assertions and Precondition
- Swift Control Flow
- Swift - Decision Making
- Swift - if statement
- Swift - if...else if...else Statement
- Swift - if-else Statement
- Swift - nested if statements
- Swift - switch statement
- Swift - Loops
- Swift - for in loop
- Swift - While loop
- Swift - repeat...while loop
- Swift - continue statement
- Swift - break statement
- Swift - fall through statement
- Swift Collections
- Swift - Arrays
- Swift - Sets
- Swift - Dictionaries
- Swift Functions
- Swift - Functions
- Swift - Nested Functions
- Swift - Function Overloading
- Swift - Recursion
- Swift - Higher-Order Functions
- Swift Closures
- Swift - Closures
- Swift-Escaping and Non-escaping closure
- Swift - Auto Closures
- Swift OOps
- Swift - Enumerations
- Swift - Structures
- Swift - Classes
- Swift - Properties
- Swift - Methods
- Swift - Subscripts
- Swift - Inheritance
- Swift-Overriding
- Swift - Initialization
- Swift - Deinitialization
- Swift Advanced
- Swift - ARC Overview
- Swift - Optional Chaining
- Swift - Error handling
- Swift - Concurrency
- Swift - Type Casting
- Swift - Nested Types
- Swift - Extensions
- Swift - Protocols
- Swift - Generics
- Swift - Access Control
- Swift - Function vs Method
- Swift - SwiftyJSON
- Swift - Singleton class
- Swift Random Numbers
- Swift Opaque and Boxed Type
- Swift Useful Resources
- Swift - Compile Online
- Swift - Quick Guide
- Swift - Useful Resources
- Swift - Discussion
Swift - Continue Statement
The continue statement is designed to be used inside the loop to skip the code inside the loop block and process the next iteration. With the help of the continue statement, we can bypass the remaining code present inside the loop for a certain iteration according to the given condition.
For a for loop, the continue statement causes the conditional test and increments the portions of the loop to execute. For while and do...while loops, the continue statement causes the program control to pass to the conditional tests.
Syntax
Following is the syntax of the continue statement −
continue
Flow Diagram
The following flow diagram will show how the continue statement works −
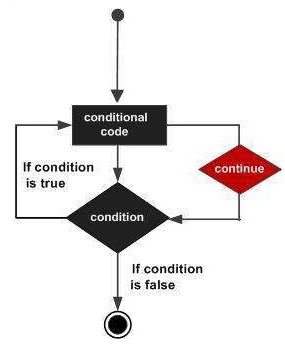
Example
Swift program to demonstrate the use of continue statement.
import Foundation let nums = [30, 2, 14, 7, 19, 11, 13, 10] // Loop to print even numbers for n in nums { if n % 2 != 0 { // Skip the code for odd numbers continue } // It will execute only for even numbers print("Even Number: \(n)") }
Output
It will produce the following output −
Even Number: 30 Even Number: 2 Even Number: 14 Even Number: 10
Example
Swift program to skip -4 from the given array using continue statement.
import Foundation let arr = [11, 12, 23, -4, 88, 92, 34, 2] for x in arr { if x == -4 { // When x = -4, skip the rest of the loop continue } print("Value: \(x)") }
Output
It will produce the following output −
Value: 11 Value: 12 Value: 23 Value: 88 Value: 92 Value: 34 Value: 2
Example
Swift program to skip city names whose length is more than 5 characters using continue statement.
import Foundation let city = ["Delhi", "Mumbai", "Jaipur", "Pune", "Goa"] for x in city { if x.count > 5 { // Skip those cities whose word count is more than 5 characters continue } print("City names: \(x)") }
Output
City names: Delhi City names: Pune City names: Goa