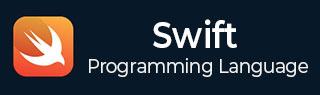
- Swift Tutorial
- Swift - Home
- Swift - Overview
- Swift - Environment
- Swift - Basic Syntax
- Swift - Variables
- Swift - Constants
- Swift - Literals
- Swift - Comments
- Swift Operators
- Swift - Operators
- Swift - Arithmetic Operators
- Swift - Comparison Operators
- Swift - Logical Operators
- Swift - Assignment Operators
- Swift - Bitwise Operators
- Swift - Misc Operators
- Swift Advanced Operators
- Swift - Operator Overloading
- Swift - Arithmetic Overflow Operators
- Swift - Identity Operators
- Swift - Range Operators
- Swift Data Types
- Swift - Data Types
- Swift - Integers
- Swift - Floating-Point Numbers
- Swift - Double
- Swift - Boolean
- Swift - Strings
- Swift - Characters
- Swift - Type Aliases
- Swift - Optionals
- Swift - Tuples
- Swift - Assertions and Precondition
- Swift Control Flow
- Swift - Decision Making
- Swift - if statement
- Swift - if...else if...else Statement
- Swift - if-else Statement
- Swift - nested if statements
- Swift - switch statement
- Swift - Loops
- Swift - for in loop
- Swift - While loop
- Swift - repeat...while loop
- Swift - continue statement
- Swift - break statement
- Swift - fall through statement
- Swift Collections
- Swift - Arrays
- Swift - Sets
- Swift - Dictionaries
- Swift Functions
- Swift - Functions
- Swift - Nested Functions
- Swift - Function Overloading
- Swift - Recursion
- Swift - Higher-Order Functions
- Swift Closures
- Swift - Closures
- Swift-Escaping and Non-escaping closure
- Swift - Auto Closures
- Swift OOps
- Swift - Enumerations
- Swift - Structures
- Swift - Classes
- Swift - Properties
- Swift - Methods
- Swift - Subscripts
- Swift - Inheritance
- Swift-Overriding
- Swift - Initialization
- Swift - Deinitialization
- Swift Advanced
- Swift - ARC Overview
- Swift - Optional Chaining
- Swift - Error handling
- Swift - Concurrency
- Swift - Type Casting
- Swift - Nested Types
- Swift - Extensions
- Swift - Protocols
- Swift - Generics
- Swift - Access Control
- Swift - Function vs Method
- Swift - SwiftyJSON
- Swift - Singleton class
- Swift Random Numbers
- Swift Opaque and Boxed Type
- Swift Useful Resources
- Swift - Compile Online
- Swift - Quick Guide
- Swift - Useful Resources
- Swift - Discussion
Swift - Comparison Operators
Comparison Operator in Swift
Comparison operators are the most frequently used operators in Swift. They are used to compare two values or expressions and return a boolean value accordingly. They are commonly used with loops and conditional statements. Swift supports the following comparison operators −
Operator | Name | Example |
---|---|---|
== | Equal to | 56 == 56 = true |
!= | Not Equal to | 56 != 78 = true |
> | Greater than | 56 > 32 = true |
< | Less than | 44 < 67 = true |
>= | Greater than or Equal to | 77 >= 33 = true |
<= | Less than or Equal to | 21 <= 56 = true |
Equal to Operator in Swift
The equal to operator is used to check if both the given values are equal or not. If they are equal, then it will return true. Otherwise, it will return false.
Syntax
Following is the syntax of the Equal to "==" operator −
Value1 == Value2
Example
Swift program to check if the input password is equal to the stored password using the equal to "==" operator.
import Foundation let password = "XP123" if (password == "XP123"){ print("Welcome!! Entered password is correct") } else { print("Error!!! Please enter correct Password") }
Output
Welcome!! Entered password is correct
Not Equal to Operator in Swift
The not equal to operator is used to check if both the given values are not equal. If they are not equal, then it will return true. Otherwise, it will return false.
Syntax
Following is the syntax of the not equal to operator −
value1 != value2
Example
Swift program to check if the input string is not equal to the stored string using the not equal to(!=) operator.
import Foundation let str = "Mohina" if (str != "Noni"){ print("Both the strings are not equal") } else { print("Both the strings are equal") }
Output
Both the strings are not equal
Greater than Operator in Swift
The greater than operator is used to check if the left-hand side value is greater than the right-hand side value. If they are, then it will return true. Otherwise, it will return false.
Syntax
Following is the syntax of the Greater than operator −
value1 > value2
Example
Swift program to add only those numbers that are greater than 50 using greater than ">" operator.
import Foundation let arr = [3, 55, 2, 44, 66] var sum = 0 // Iterate through each element of the array for x in arr{ // Find the sum of only those elements that // are greater than 50 if (x > 50){ sum += x } } print("Sum = \(sum)")
Output
Sum = 121
Less than Operator in Swift
The less than operator is used to check if the left-hand side value is less than the right-hand side value. If they are, then it will return true. Otherwise, it will return false.
Syntax
Following is the syntax of the Less than operator −
value1 < value2
Example
Swift program to add only those numbers that are less than 55 using less than(<) operator.
import Foundation let arr = [1, 55, 2, 90, 12] var sum = 0 // Iterate through each element of the array for x in arr{ // Find the sum of only those elements that // are less than 55 if (x < 55){ sum += x } } print("Sum = \(sum)")
Output
Sum = 15
Greater than or Equal to Operator in Swift
The greater than or equal to operator is used to check if the left-hand side value is greater than or equal to the right-hand side value. If they are, then it will return true. Otherwise, it will return false.
Syntax
Following is the syntax of the Greater than or Equal to operator −
value1 >= value2
Example
Swift program to check valid age for voting using greater than or equal to(>=) operator.
import Foundation let age = 18 if (age >= 18){ print("You are eligible for voting") } else { print("You are not eligible for voting") }
Output
You are eligible for voting
Less than or Equal to Operator in Swift
The less than or equal to operator is used to check if the left-hand side value is less than or equal to the right-hand side value. If they are, then it will return true.
Syntax
Following is the syntax of the Less than or Equal to operator −
value1 <= value2
Example
Swift program to compare two numbers using less than or equal to operator.
import Foundation let num = 18 if (num <= 20){ print("Given num is less than 20") } else { print("Given num is greater than 20") }
Output
Given num is less than 20