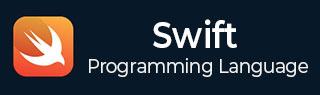
- Swift Tutorial
- Swift - Home
- Swift - Overview
- Swift - Environment
- Swift - Basic Syntax
- Swift - Variables
- Swift - Constants
- Swift - Literals
- Swift - Comments
- Swift Operators
- Swift - Operators
- Swift - Arithmetic Operators
- Swift - Comparison Operators
- Swift - Logical Operators
- Swift - Assignment Operators
- Swift - Bitwise Operators
- Swift - Misc Operators
- Swift Advanced Operators
- Swift - Operator Overloading
- Swift - Arithmetic Overflow Operators
- Swift - Identity Operators
- Swift - Range Operators
- Swift Data Types
- Swift - Data Types
- Swift - Integers
- Swift - Floating-Point Numbers
- Swift - Double
- Swift - Boolean
- Swift - Strings
- Swift - Characters
- Swift - Type Aliases
- Swift - Optionals
- Swift - Tuples
- Swift - Assertions and Precondition
- Swift Control Flow
- Swift - Decision Making
- Swift - if statement
- Swift - if...else if...else Statement
- Swift - if-else Statement
- Swift - nested if statements
- Swift - switch statement
- Swift - Loops
- Swift - for in loop
- Swift - While loop
- Swift - repeat...while loop
- Swift - continue statement
- Swift - break statement
- Swift - fall through statement
- Swift Collections
- Swift - Arrays
- Swift - Sets
- Swift - Dictionaries
- Swift Functions
- Swift - Functions
- Swift - Nested Functions
- Swift - Function Overloading
- Swift - Recursion
- Swift - Higher-Order Functions
- Swift Closures
- Swift - Closures
- Swift-Escaping and Non-escaping closure
- Swift - Auto Closures
- Swift OOps
- Swift - Enumerations
- Swift - Structures
- Swift - Classes
- Swift - Properties
- Swift - Methods
- Swift - Subscripts
- Swift - Inheritance
- Swift-Overriding
- Swift - Initialization
- Swift - Deinitialization
- Swift Advanced
- Swift - ARC Overview
- Swift - Optional Chaining
- Swift - Error handling
- Swift - Concurrency
- Swift - Type Casting
- Swift - Nested Types
- Swift - Extensions
- Swift - Protocols
- Swift - Generics
- Swift - Access Control
- Swift - Function vs Method
- Swift - SwiftyJSON
- Swift - Singleton class
- Swift Random Numbers
- Swift Opaque and Boxed Type
- Swift Useful Resources
- Swift - Compile Online
- Swift - Quick Guide
- Swift - Useful Resources
- Swift - Discussion
Swift - Characters
A character in Swift is a single character String literal such as “A", "!", “c", addressed by the data type Character. Or we can say that the character data type is designed to represent a single Unicode character.
Syntax
Following is the syntax to declare a character data type −
var char : Character = "A"
Example
Swift program to create two variables to store characters.
import Foundation let char1: Character = "A" let char2: Character = "B" print("Value of char1 \(char1)") print("Value of char2 \(char2)")
Output
Value of char1 A Value of char2 B
Example
If we try to store more than one character in a Character type variable or constant, then Swift will not allow that and give an error before compilation.
import Foundation // Following is illegal in Swift let char: Character = "AB" print("Value of char \(char)")
Output
main.swift:4:23: error: cannot convert value of type 'String' to specified type 'Character' let char: Character = "AB"
Example
Also, we are not allowed to create an empty Character variable or constant which will have an empty value. If we try to do we will get an error.
import Foundation // Creating empty Character let char1: Character = "" var char2: Character = "" print("Value of char1 \(char1)") print("Value of char2 \(char2)")
Output
main.swift:4:24: error: cannot convert value of type 'String' to specified type 'Character' let char1: Character = "" ^~ main.swift:5:24: error: cannot convert value of type 'String' to specified type 'Character' var char2: Character = "" ^~
Accessing Characters from Strings in Swift
A string represents a collection of Character values in a specified order. So we can access individual characters from the given String by iterating over that string with a for-in loop −
Example
import Foundation // Accessing Characters from Strings using for-in loop for ch in "Hello" { print(ch) }
Output
H e l l o
Concatenating String with Character in Swift
In Swift, we can concatenate String with a character using the + and += operators. Both the operators concatenate the given character at the end of the specified string.
While concatenating a string with a character we required an explicit conversion from character to string because Swift enforces strong typing. Also, we are not allowed to concatenate a character(variable) with a string.
Example
Swift program to concatenate a string with a character using the + operator.
import Foundation let str = "Swift" let char: Character = "#" // Concatenating string with character using + operator let concatenatedStr = str + String(char) print(concatenatedStr)
Output
Swift#
Example
Swift program to concatenate a string with a character using the += operator.
import Foundation var str = "Swift" let char: Character = "!" // Concatenating string with character using += operator str += String(char) print(str)
Output
Swift!