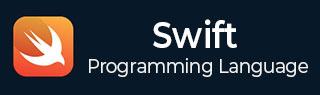
- Swift Tutorial
- Swift - Home
- Swift - Overview
- Swift - Environment
- Swift - Basic Syntax
- Swift - Variables
- Swift - Constants
- Swift - Literals
- Swift - Comments
- Swift Operators
- Swift - Operators
- Swift - Arithmetic Operators
- Swift - Comparison Operators
- Swift - Logical Operators
- Swift - Assignment Operators
- Swift - Bitwise Operators
- Swift - Misc Operators
- Swift Advanced Operators
- Swift - Operator Overloading
- Swift - Arithmetic Overflow Operators
- Swift - Identity Operators
- Swift - Range Operators
- Swift Data Types
- Swift - Data Types
- Swift - Integers
- Swift - Floating-Point Numbers
- Swift - Double
- Swift - Boolean
- Swift - Strings
- Swift - Characters
- Swift - Type Aliases
- Swift - Optionals
- Swift - Tuples
- Swift - Assertions and Precondition
- Swift Control Flow
- Swift - Decision Making
- Swift - if statement
- Swift - if...else if...else Statement
- Swift - if-else Statement
- Swift - nested if statements
- Swift - switch statement
- Swift - Loops
- Swift - for in loop
- Swift - While loop
- Swift - repeat...while loop
- Swift - continue statement
- Swift - break statement
- Swift - fall through statement
- Swift Collections
- Swift - Arrays
- Swift - Sets
- Swift - Dictionaries
- Swift Functions
- Swift - Functions
- Swift - Nested Functions
- Swift - Function Overloading
- Swift - Recursion
- Swift - Higher-Order Functions
- Swift Closures
- Swift - Closures
- Swift-Escaping and Non-escaping closure
- Swift - Auto Closures
- Swift OOps
- Swift - Enumerations
- Swift - Structures
- Swift - Classes
- Swift - Properties
- Swift - Methods
- Swift - Subscripts
- Swift - Inheritance
- Swift-Overriding
- Swift - Initialization
- Swift - Deinitialization
- Swift Advanced
- Swift - ARC Overview
- Swift - Optional Chaining
- Swift - Error handling
- Swift - Concurrency
- Swift - Type Casting
- Swift - Nested Types
- Swift - Extensions
- Swift - Protocols
- Swift - Generics
- Swift - Access Control
- Swift - Function vs Method
- Swift - SwiftyJSON
- Swift - Singleton class
- Swift Random Numbers
- Swift Opaque and Boxed Type
- Swift Useful Resources
- Swift - Compile Online
- Swift - Quick Guide
- Swift - Useful Resources
- Swift - Discussion
Swift - Break Statement
Swift Break Statement
The break statement in Swift is designed to terminate the control flow statements prematurely. Or we can say that it is used to terminate the loop at a certain condition before reaching its final conclusion. We can use break statements with –
- Loop Statements
- Switch Statements
Syntax
Following is the syntax of the break statement −
break
Flow Diagram
The following flow diagram will show how the break statement works −
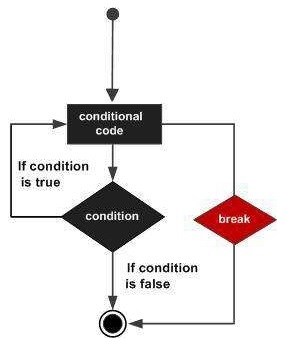
Swift break Statement with Loops
The break statement is commonly used with loops. In a loop, when the break condition is true, the loop immediately terminates its iterations and the controls will return to the statement present just after the loop. We can use break statement inside the for-in loop, while loop and in nested loops. If we are using nested loops (i.e., one loop inside another loop), then the break statement will stop the execution of the innermost loop and start executing the next line of the code after the block.
Example
Swift program to demonstrate the use of break statement in a for-in loop.
import Foundation print("Numbers:") for x in 1...5 { if x == 3 { // When x is equal to 3 the loop will terminate break } print(x) }
Output
It will produce the following output −
Numbers: 1 2
Example
Swift program to demonstrate the use of break statement in a nested loop.
import Foundation // Outer loop for x in 1...6 { // Inner loop for y in 1...5 { if y == 4 { // When y = 4 terminate the inner loops break } print("(\(x), \(y))") } }
Output
It will produce the following output −
(1, 1) (1, 2) (1, 3) (2, 1) (2, 2) (2, 3) (3, 1) (3, 2) (3, 3) (4, 1) (4, 2) (4, 3) (5, 1) (5, 2) (5, 3) (6, 1) (6, 2) (6, 3)
Example
Swift program to demonstrate the use of break statement in a while loop.
import Foundation var x = 2 while true { print("Current value of x: \(x)") if x == 8 { print("Loop ends because x is equal to 8.") break } x += 1 }
Output
It will produce the following output −
Current value of x: 2 Current value of x: 3 Current value of x: 4 Current value of x: 5 Current value of x: 6 Current value of x: 7 Current value of x: 8 Loop ends because x is equal to 8.
Swift break Statement with Switch Statement
We can also use a break statement in the switch statement to terminate the switch block after the match of a certain case and control moves to the next statement present after the switch block. All the cases present in the switch must explicitly be terminated using a break statement.
By default, Swift does not fall through to the next case, however, if you want then you can use the fall-through statement.
Example
Swift program to demonstrate the use of a break statement in a switch statement.
import Foundation let color = "Blue" switch color { case "Blue", "Black", "Brown": print("Dark colors") // Using break statement to break the switch statement break case "baby pink", "Blush", "Peach": print("Pastel Colors") default: print("Invalid Color") }
Output
It will produce the following output −
Dark colors