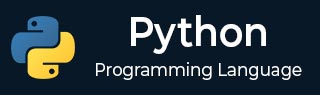
- Python Basics
- Python - Home
- Python - Overview
- Python - History
- Python - Features
- Python vs C++
- Python - Hello World Program
- Python - Application Areas
- Python - Interpreter
- Python - Environment Setup
- Python - Virtual Environment
- Python - Basic Syntax
- Python - Variables
- Python - Data Types
- Python - Type Casting
- Python - Unicode System
- Python - Literals
- Python - Operators
- Python - Arithmetic Operators
- Python - Comparison Operators
- Python - Assignment Operators
- Python - Logical Operators
- Python - Bitwise Operators
- Python - Membership Operators
- Python - Identity Operators
- Python - Operator Precedence
- Python - Comments
- Python - User Input
- Python - Numbers
- Python - Booleans
- Python Control Statements
- Python - Control Flow
- Python - Decision Making
- Python - If Statement
- Python - If else
- Python - Nested If
- Python - Match-Case Statement
- Python - Loops
- Python - for Loops
- Python - for-else Loops
- Python - While Loops
- Python - break Statement
- Python - continue Statement
- Python - pass Statement
- Python - Nested Loops
- Python Functions & Modules
- Python - Functions
- Python - Default Arguments
- Python - Keyword Arguments
- Python - Keyword-Only Arguments
- Python - Positional Arguments
- Python - Positional-Only Arguments
- Python - Arbitrary Arguments
- Python - Variables Scope
- Python - Function Annotations
- Python - Modules
- Python - Built in Functions
- Python Strings
- Python - Strings
- Python - Slicing Strings
- Python - Modify Strings
- Python - String Concatenation
- Python - String Formatting
- Python - Escape Characters
- Python - String Methods
- Python - String Exercises
- Python Lists
- Python - Lists
- Python - Access List Items
- Python - Change List Items
- Python - Add List Items
- Python - Remove List Items
- Python - Loop Lists
- Python - List Comprehension
- Python - Sort Lists
- Python - Copy Lists
- Python - Join Lists
- Python - List Methods
- Python - List Exercises
- Python Tuples
- Python - Tuples
- Python - Access Tuple Items
- Python - Update Tuples
- Python - Unpack Tuples
- Python - Loop Tuples
- Python - Join Tuples
- Python - Tuple Methods
- Python - Tuple Exercises
- Python Sets
- Python - Sets
- Python - Access Set Items
- Python - Add Set Items
- Python - Remove Set Items
- Python - Loop Sets
- Python - Join Sets
- Python - Copy Sets
- Python - Set Operators
- Python - Set Methods
- Python - Set Exercises
- Python Dictionaries
- Python - Dictionaries
- Python - Access Dictionary Items
- Python - Change Dictionary Items
- Python - Add Dictionary Items
- Python - Remove Dictionary Items
- Python - Dictionary View Objects
- Python - Loop Dictionaries
- Python - Copy Dictionaries
- Python - Nested Dictionaries
- Python - Dictionary Methods
- Python - Dictionary Exercises
- Python Arrays
- Python - Arrays
- Python - Access Array Items
- Python - Add Array Items
- Python - Remove Array Items
- Python - Loop Arrays
- Python - Copy Arrays
- Python - Reverse Arrays
- Python - Sort Arrays
- Python - Join Arrays
- Python - Array Methods
- Python - Array Exercises
- Python File Handling
- Python - File Handling
- Python - Write to File
- Python - Read Files
- Python - Renaming and Deleting Files
- Python - Directories
- Python - File Methods
- Python - OS File/Directory Methods
- Python - OS Path Methods
- Object Oriented Programming
- Python - OOPs Concepts
- Python - Classes & Objects
- Python - Class Attributes
- Python - Class Methods
- Python - Static Methods
- Python - Constructors
- Python - Access Modifiers
- Python - Inheritance
- Python - Polymorphism
- Python - Method Overriding
- Python - Method Overloading
- Python - Dynamic Binding
- Python - Dynamic Typing
- Python - Abstraction
- Python - Encapsulation
- Python - Interfaces
- Python - Packages
- Python - Inner Classes
- Python - Anonymous Class and Objects
- Python - Singleton Class
- Python - Wrapper Classes
- Python - Enums
- Python - Reflection
- Python Errors & Exceptions
- Python - Syntax Errors
- Python - Exceptions
- Python - try-except Block
- Python - try-finally Block
- Python - Raising Exceptions
- Python - Exception Chaining
- Python - Nested try Block
- Python - User-defined Exception
- Python - Logging
- Python - Assertions
- Python - Built-in Exceptions
- Python Multithreading
- Python - Multithreading
- Python - Thread Life Cycle
- Python - Creating a Thread
- Python - Starting a Thread
- Python - Joining Threads
- Python - Naming Thread
- Python - Thread Scheduling
- Python - Thread Pools
- Python - Main Thread
- Python - Thread Priority
- Python - Daemon Threads
- Python - Synchronizing Threads
- Python Synchronization
- Python - Inter-thread Communication
- Python - Thread Deadlock
- Python - Interrupting a Thread
- Python Networking
- Python - Networking
- Python - Socket Programming
- Python - URL Processing
- Python - Generics
- Python Libraries
- NumPy Tutorial
- Pandas Tutorial
- SciPy Tutorial
- Matplotlib Tutorial
- Django Tutorial
- OpenCV Tutorial
- Python Miscellenous
- Python - Date & Time
- Python - Maths
- Python - Iterators
- Python - Generators
- Python - Closures
- Python - Decorators
- Python - Recursion
- Python - Reg Expressions
- Python - PIP
- Python - Database Access
- Python - Weak References
- Python - Serialization
- Python - Templating
- Python - Output Formatting
- Python - Performance Measurement
- Python - Data Compression
- Python - CGI Programming
- Python - XML Processing
- Python - GUI Programming
- Python - Command-Line Arguments
- Python - Docstrings
- Python - JSON
- Python - Sending Email
- Python - Further Extensions
- Python - Tools/Utilities
- Python - GUIs
- Python Useful Resources
- Python Compiler
- NumPy Compiler
- Matplotlib Compiler
- SciPy Compiler
- Python - Questions & Answers
- Python - Online Quiz
- Python - Programming Examples
- Python - Quick Guide
- Python - Useful Resources
- Python - Discussion
Python - Remove List Items
Removing List Items
Removing list items in Python implies deleting elements from an existing list. Lists are ordered collections of items, and sometimes you need to remove certain elements from them based on specific criteria or indices. When we remove list items, we are reducing the size of the list or eliminating specific elements.
We can remove list items in Python using various methods such as remove(), pop() and clear(). Additionally, we can use the del statement to remove items at a specific index. Let us explore through all these methods in this tutorial.
Remove List Item Using remove() Method
The remove() method in Python is used to remove the first occurrence of a specified item from a list.
We can remove list items using the remove() method by specifying the value we want to remove within the parentheses, like my_list.remove(value), which deletes the first occurrence of value from my_list.
Example
In the following example, we are deleting the element "Physics" from the list "list1" using the remove() method −
list1 = ["Rohan", "Physics", 21, 69.75] print ("Original list: ", list1) list1.remove("Physics") print ("List after removing: ", list1)
It will produce the following output −
Original list: ['Rohan', 'Physics', 21, 69.75] List after removing: ['Rohan', 21, 69.75]
Remove List Item Using pop() Method
The pop() method in Python is used to removes and returns the last element from a list if no index is specified, or removes and returns the element at a specified index, altering the original list.
We can remove list items using the pop() method by calling it without any arguments my_list.pop(), which removes and returns the last item from my_list, or by providing the index of the item we want to remove my_list.pop(index), which removes and returns the item at that index.
Example
The following example shows how you can use the pop() method to remove list items −
list2 = [25.50, True, -55, 1+2j] print ("Original list: ", list2) list2.pop(2) print ("List after popping: ", list2)
We get the output as shown below −
Original list: [25.5, True, -55, (1+2j)] List after popping: [25.5, True, (1+2j)]
Remove List Item Using clear() Method
The clear() method in Python is used to remove all elements from a list, leaving it empty.
We can remove all list items using the clear() method by calling it on the list object like my_list.clear(), which empties my_list, leaving it with no elements.
Example
In this example, we are using the clear() method to remove all elements from the list "my_list" −
my_list = [1, 2, 3, 4, 5] # Clearing the list my_list.clear() print("Cleared list:", my_list)
Output of the above code is as follows −
Cleared list: []
Remove List Item Using del Keyword
The del keyword in Python is used to delete element either at a specific index or a slice of indices from memory.
We can remove list items using the del keyword by specifying the index or slice of the items we want to delete, like del my_list[index] to delete a single item or del my_list[start:stop] to delete a range of items.
Example
In the below example, we are using the "del" keyword to delete an element at the index "2" from the list "list1" −
list1 = ["a", "b", "c", "d"] print ("Original list: ", list1) del list1[2] print ("List after deleting: ", list1)
The result produced is as follows −
Original list: ['a', 'b', 'c', 'd'] List after deleting: ['a', 'b', 'd']
Example
In here, we are deleting a series of consecutive items from a list with the slicing operator −
list2 = [25.50, True, -55, 1+2j] print ("List before deleting: ", list2) del list2[0:2] print ("List after deleting: ", list2)
It will produce the following output −
List before deleting: [25.5, True, -55, (1+2j)] List after deleting: [-55, (1+2j)]