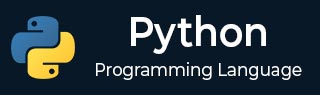
- Python Basics
- Python - Home
- Python - Overview
- Python - History
- Python - Features
- Python vs C++
- Python - Hello World Program
- Python - Application Areas
- Python - Interpreter
- Python - Environment Setup
- Python - Virtual Environment
- Python - Basic Syntax
- Python - Variables
- Python - Data Types
- Python - Type Casting
- Python - Unicode System
- Python - Literals
- Python - Operators
- Python - Arithmetic Operators
- Python - Comparison Operators
- Python - Assignment Operators
- Python - Logical Operators
- Python - Bitwise Operators
- Python - Membership Operators
- Python - Identity Operators
- Python - Operator Precedence
- Python - Comments
- Python - User Input
- Python - Numbers
- Python - Booleans
- Python Control Statements
- Python - Control Flow
- Python - Decision Making
- Python - If Statement
- Python - If else
- Python - Nested If
- Python - Match-Case Statement
- Python - Loops
- Python - for Loops
- Python - for-else Loops
- Python - While Loops
- Python - break Statement
- Python - continue Statement
- Python - pass Statement
- Python - Nested Loops
- Python Functions & Modules
- Python - Functions
- Python - Default Arguments
- Python - Keyword Arguments
- Python - Keyword-Only Arguments
- Python - Positional Arguments
- Python - Positional-Only Arguments
- Python - Arbitrary Arguments
- Python - Variables Scope
- Python - Function Annotations
- Python - Modules
- Python - Built in Functions
- Python Strings
- Python - Strings
- Python - Slicing Strings
- Python - Modify Strings
- Python - String Concatenation
- Python - String Formatting
- Python - Escape Characters
- Python - String Methods
- Python - String Exercises
- Python Lists
- Python - Lists
- Python - Access List Items
- Python - Change List Items
- Python - Add List Items
- Python - Remove List Items
- Python - Loop Lists
- Python - List Comprehension
- Python - Sort Lists
- Python - Copy Lists
- Python - Join Lists
- Python - List Methods
- Python - List Exercises
- Python Tuples
- Python - Tuples
- Python - Access Tuple Items
- Python - Update Tuples
- Python - Unpack Tuples
- Python - Loop Tuples
- Python - Join Tuples
- Python - Tuple Methods
- Python - Tuple Exercises
- Python Sets
- Python - Sets
- Python - Access Set Items
- Python - Add Set Items
- Python - Remove Set Items
- Python - Loop Sets
- Python - Join Sets
- Python - Copy Sets
- Python - Set Operators
- Python - Set Methods
- Python - Set Exercises
- Python Dictionaries
- Python - Dictionaries
- Python - Access Dictionary Items
- Python - Change Dictionary Items
- Python - Add Dictionary Items
- Python - Remove Dictionary Items
- Python - Dictionary View Objects
- Python - Loop Dictionaries
- Python - Copy Dictionaries
- Python - Nested Dictionaries
- Python - Dictionary Methods
- Python - Dictionary Exercises
- Python Arrays
- Python - Arrays
- Python - Access Array Items
- Python - Add Array Items
- Python - Remove Array Items
- Python - Loop Arrays
- Python - Copy Arrays
- Python - Reverse Arrays
- Python - Sort Arrays
- Python - Join Arrays
- Python - Array Methods
- Python - Array Exercises
- Python File Handling
- Python - File Handling
- Python - Write to File
- Python - Read Files
- Python - Renaming and Deleting Files
- Python - Directories
- Python - File Methods
- Python - OS File/Directory Methods
- Python - OS Path Methods
- Object Oriented Programming
- Python - OOPs Concepts
- Python - Classes & Objects
- Python - Class Attributes
- Python - Class Methods
- Python - Static Methods
- Python - Constructors
- Python - Access Modifiers
- Python - Inheritance
- Python - Polymorphism
- Python - Method Overriding
- Python - Method Overloading
- Python - Dynamic Binding
- Python - Dynamic Typing
- Python - Abstraction
- Python - Encapsulation
- Python - Interfaces
- Python - Packages
- Python - Inner Classes
- Python - Anonymous Class and Objects
- Python - Singleton Class
- Python - Wrapper Classes
- Python - Enums
- Python - Reflection
- Python Errors & Exceptions
- Python - Syntax Errors
- Python - Exceptions
- Python - try-except Block
- Python - try-finally Block
- Python - Raising Exceptions
- Python - Exception Chaining
- Python - Nested try Block
- Python - User-defined Exception
- Python - Logging
- Python - Assertions
- Python - Built-in Exceptions
- Python Multithreading
- Python - Multithreading
- Python - Thread Life Cycle
- Python - Creating a Thread
- Python - Starting a Thread
- Python - Joining Threads
- Python - Naming Thread
- Python - Thread Scheduling
- Python - Thread Pools
- Python - Main Thread
- Python - Thread Priority
- Python - Daemon Threads
- Python - Synchronizing Threads
- Python Synchronization
- Python - Inter-thread Communication
- Python - Thread Deadlock
- Python - Interrupting a Thread
- Python Networking
- Python - Networking
- Python - Socket Programming
- Python - URL Processing
- Python - Generics
- Python Libraries
- NumPy Tutorial
- Pandas Tutorial
- SciPy Tutorial
- Matplotlib Tutorial
- Django Tutorial
- OpenCV Tutorial
- Python Miscellenous
- Python - Date & Time
- Python - Maths
- Python - Iterators
- Python - Generators
- Python - Closures
- Python - Decorators
- Python - Recursion
- Python - Reg Expressions
- Python - PIP
- Python - Database Access
- Python - Weak References
- Python - Serialization
- Python - Templating
- Python - Output Formatting
- Python - Performance Measurement
- Python - Data Compression
- Python - CGI Programming
- Python - XML Processing
- Python - GUI Programming
- Python - Command-Line Arguments
- Python - Docstrings
- Python - JSON
- Python - Sending Email
- Python - Further Extensions
- Python - Tools/Utilities
- Python - GUIs
- Python Useful Resources
- Python Compiler
- NumPy Compiler
- Matplotlib Compiler
- SciPy Compiler
- Python - Questions & Answers
- Python - Online Quiz
- Python - Programming Examples
- Python - Quick Guide
- Python - Useful Resources
- Python - Discussion
Python - Operators
Python Operators
Python operators are special symbols (sometimes called keywords) that are used to perform certain most commonly required operations on one or more operands (value, variables, or expressions).
Types of Operators in Python
Python language supports the following types of operators −
Arithmetic Operators
Comparison (Relational) Operators
Assignment Operators
Logical Operators
Bitwise Operators
Membership Operators
Identity Operators
Let us have a look at all the operators one by one.
Python Arithmetic Operators
Arithmetic operators are used to perform basic mathematical operations such as addition, subtraction, multiplication, etc.
Assume variable a holds 10 and variable b holds 20, then
Operator | Name | Example |
---|---|---|
+ | Addition | a + b = 30 |
- | Subtraction | a – b = -10 |
* | Multiplication | a * b = 200 |
/ | Division | b / a = 2 |
% | Modulus | b % a = 0 |
** | Exponent | a**b =10**20 |
// | Floor Division | 9//2 = 4 |
Example of Python Arithmetic Operators
a = 21 b = 10 c = 0 c = a + b print ("a: {} b: {} a+b: {}".format(a,b,c)) c = a - b print ("a: {} b: {} a-b: {}".format(a,b,c) ) c = a * b print ("a: {} b: {} a*b: {}".format(a,b,c)) c = a / b print ("a: {} b: {} a/b: {}".format(a,b,c)) c = a % b print ("a: {} b: {} a%b: {}".format(a,b,c)) a = 2 b = 3 c = a**b print ("a: {} b: {} a**b: {}".format(a,b,c)) a = 10 b = 5 c = a//b print ("a: {} b: {} a//b: {}".format(a,b,c))
Output
a: 21 b: 10 a+b: 31 a: 21 b: 10 a-b: 11 a: 21 b: 10 a*b: 210 a: 21 b: 10 a/b: 2.1 a: 21 b: 10 a%b: 1 a: 2 b: 3 a**b: 8 a: 10 b: 5 a//b: 2
Python Comparison Operators
Comparison operators compare the values on either side of them and decide the relation among them. They are also called Relational operators.
Assume variable a holds 10 and variable b holds 20, then
Operator | Name | Example |
---|---|---|
== | Equal | (a == b) is not true. |
!= | Not equal | (a != b) is true. |
> | Greater than | (a > b) is not true. |
< | Less than | (a < b) is true. |
>= | Greater than or equal to | (a >= b) is not true. |
<= | Less than or equal to | (a <= b) is true. |
Example of Python Comparison Operators
a = 21 b = 10 if ( a == b ): print ("Line 1 - a is equal to b") else: print ("Line 1 - a is not equal to b") if ( a != b ): print ("Line 2 - a is not equal to b") else: print ("Line 2 - a is equal to b") if ( a < b ): print ("Line 3 - a is less than b" ) else: print ("Line 3 - a is not less than b") if ( a > b ): print ("Line 4 - a is greater than b") else: print ("Line 4 - a is not greater than b") a,b=b,a #values of a and b swapped. a becomes 10, b becomes 21 if ( a <= b ): print ("Line 5 - a is either less than or equal to b") else: print ("Line 5 - a is neither less than nor equal to b") if ( b >= a ): print ("Line 6 - b is either greater than or equal to b") else: print ("Line 6 - b is neither greater than nor equal to b")
Output
Line 1 - a is not equal to b Line 2 - a is not equal to b Line 3 - a is not less than b Line 4 - a is greater than b Line 5 - a is either less than or equal to b Line 6 - b is either greater than or equal to b
Python Assignment Operators
Assignment operators are used to assign values to variables. Following is a table which shows all Python assignment operators.
Operator | Example | Same As |
---|---|---|
= | a = 10 | a = 10 |
+= | a += 30 | a = a + 30 |
-= | a -= 15 | a = a - 15 |
*= | a *= 10 | a = a * 10 |
/= | a /= 5 | a = a / 5 |
%= | a %= 5 | a = a % 5 |
**= | a **= 4 | a = a ** 4 |
//= | a //= 5 | a = a // 5 |
&= | a &= 5 | a = a & 5 |
|= | a |= 5 | a = a | 5 |
^= | a ^= 5 | a = a ^ 5 |
>>= | a >>= 5 | a = a >> 5 |
<<= | a <<= 5 | a = a << 5 |
Example of Python Assignment Operators
a = 21 b = 10 c = 0 print ("a: {} b: {} c : {}".format(a,b,c)) c = a + b print ("a: {} c = a + b: {}".format(a,c)) c += a print ("a: {} c += a: {}".format(a,c)) c *= a print ("a: {} c *= a: {}".format(a,c)) c /= a print ("a: {} c /= a : {}".format(a,c)) c = 2 print ("a: {} b: {} c : {}".format(a,b,c)) c %= a print ("a: {} c %= a: {}".format(a,c)) c **= a print ("a: {} c **= a: {}".format(a,c)) c //= a print ("a: {} c //= a: {}".format(a,c))
Output
a: 21 b: 10 c : 0 a: 21 c = a + b: 31 a: 21 c += a: 52 a: 21 c *= a: 1092 a: 21 c /= a : 52.0 a: 21 b: 10 c : 2 a: 21 c %= a: 2 a: 21 c **= a: 2097152 a: 21 c //= a: 99864
Python Bitwise Operators
Bitwise operator works on bits and performs bit by bit operation. These operators are used to compare binary numbers.
There are following Bitwise operators supported by Python language
Operator | Name | Example |
---|---|---|
& | AND | a & b |
| | OR | a | b |
^ | XOR | a ^ b |
~ | NOT | ~a |
<< | Zero fill left shift | a << 3 |
>> | Signed right shift | a >> 3 |
Example of Python Bitwise Operators
a = 20 b = 10 print ('a=',a,':',bin(a),'b=',b,':',bin(b)) c = 0 c = a & b; print ("result of AND is ", c,':',bin(c)) c = a | b; print ("result of OR is ", c,':',bin(c)) c = a ^ b; print ("result of EXOR is ", c,':',bin(c)) c = ~a; print ("result of COMPLEMENT is ", c,':',bin(c)) c = a << 2; print ("result of LEFT SHIFT is ", c,':',bin(c)) c = a >> 2; print ("result of RIGHT SHIFT is ", c,':',bin(c))
Output
a= 20 : 0b10100 b= 10 : 0b1010 result of AND is 0 : 0b0 result of OR is 30 : 0b11110 result of EXOR is 30 : 0b11110 result of COMPLEMENT is -21 : -0b10101 result of LEFT SHIFT is 80 : 0b1010000 result of RIGHT SHIFT is 5 : 0b101
Python Logical Operators
Python logical operators are used to combile two or more conditions and check the final result. There are following logical operators supported by Python language. Assume variable a holds 10 and variable b holds 20 then
Operator | Name | Example |
---|---|---|
and | AND | a and b |
or | OR | a or b |
not | NOT | not(a) |
Example of Python Logical Operators
var = 5 print(var > 3 and var < 10) print(var > 3 or var < 4) print(not (var > 3 and var < 10))
Output
True True False
Python Membership Operators
Python's membership operators test for membership in a sequence, such as strings, lists, or tuples. There are two membership operators as explained below −
Operator | Description | Example |
---|---|---|
in | Returns True if it finds a variable in the specified sequence, false otherwise. | a in b |
not in | returns True if it does not finds a variable in the specified sequence and false otherwise. | a not in b |
Example of Python Membership Operators
a = 10 b = 20 list = [1, 2, 3, 4, 5 ] print ("a:", a, "b:", b, "list:", list) if ( a in list ): print ("a is present in the given list") else: print ("a is not present in the given list") if ( b not in list ): print ("b is not present in the given list") else: print ("b is present in the given list") c=b/a print ("c:", c, "list:", list) if ( c in list ): print ("c is available in the given list") else: print ("c is not available in the given list")
Output
a: 10 b: 20 list: [1, 2, 3, 4, 5] a is not present in the given list b is not present in the given list c: 2.0 list: [1, 2, 3, 4, 5] c is available in the given list
Python Identity Operators
Identity operators compare the memory locations of two objects. There are two Identity operators explained below −
Operator | Description | Example |
---|---|---|
is | Returns True if both variables are the same object and false otherwise. | a is b |
is not | Returns True if both variables are not the same object and false otherwise. | a is not b |
Example of Python Identity Operators
a = [1, 2, 3, 4, 5] b = [1, 2, 3, 4, 5] c = a print(a is c) print(a is b) print(a is not c) print(a is not b)
Output
True False False True
Python Operators Precedence
The following table lists all operators from highest precedence to lowest.
Sr.No. | Operator & Description |
---|---|
1 | ** Exponentiation (raise to the power) |
2 | ~ + - Complement, unary plus and minus (method names for the last two are +@ and -@) |
3 | * / % // Multiply, divide, modulo and floor division |
4 | + - Addition and subtraction |
5 | >> << Right and left bitwise shift |
6 | & Bitwise 'AND' |
7 | ^ | Bitwise exclusive `OR' and regular `OR' |
8 | <= < > >= Comparison operators |
9 | <> == != Equality operators |
10 | = %= /= //= -= += *= **= Assignment operators |
11 | is is not Identity operators |
12 | in not in Membership operators |
13 | not or and Logical operators |