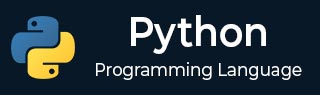
- Python Basics
- Python - Home
- Python - Overview
- Python - History
- Python - Features
- Python vs C++
- Python - Hello World Program
- Python - Application Areas
- Python - Interpreter
- Python - Environment Setup
- Python - Virtual Environment
- Python - Basic Syntax
- Python - Variables
- Python - Data Types
- Python - Type Casting
- Python - Unicode System
- Python - Literals
- Python - Operators
- Python - Arithmetic Operators
- Python - Comparison Operators
- Python - Assignment Operators
- Python - Logical Operators
- Python - Bitwise Operators
- Python - Membership Operators
- Python - Identity Operators
- Python - Operator Precedence
- Python - Comments
- Python - User Input
- Python - Numbers
- Python - Booleans
- Python Control Statements
- Python - Control Flow
- Python - Decision Making
- Python - If Statement
- Python - If else
- Python - Nested If
- Python - Match-Case Statement
- Python - Loops
- Python - for Loops
- Python - for-else Loops
- Python - While Loops
- Python - break Statement
- Python - continue Statement
- Python - pass Statement
- Python - Nested Loops
- Python Functions & Modules
- Python - Functions
- Python - Default Arguments
- Python - Keyword Arguments
- Python - Keyword-Only Arguments
- Python - Positional Arguments
- Python - Positional-Only Arguments
- Python - Arbitrary Arguments
- Python - Variables Scope
- Python - Function Annotations
- Python - Modules
- Python - Built in Functions
- Python Strings
- Python - Strings
- Python - Slicing Strings
- Python - Modify Strings
- Python - String Concatenation
- Python - String Formatting
- Python - Escape Characters
- Python - String Methods
- Python - String Exercises
- Python Lists
- Python - Lists
- Python - Access List Items
- Python - Change List Items
- Python - Add List Items
- Python - Remove List Items
- Python - Loop Lists
- Python - List Comprehension
- Python - Sort Lists
- Python - Copy Lists
- Python - Join Lists
- Python - List Methods
- Python - List Exercises
- Python Tuples
- Python - Tuples
- Python - Access Tuple Items
- Python - Update Tuples
- Python - Unpack Tuples
- Python - Loop Tuples
- Python - Join Tuples
- Python - Tuple Methods
- Python - Tuple Exercises
- Python Sets
- Python - Sets
- Python - Access Set Items
- Python - Add Set Items
- Python - Remove Set Items
- Python - Loop Sets
- Python - Join Sets
- Python - Copy Sets
- Python - Set Operators
- Python - Set Methods
- Python - Set Exercises
- Python Dictionaries
- Python - Dictionaries
- Python - Access Dictionary Items
- Python - Change Dictionary Items
- Python - Add Dictionary Items
- Python - Remove Dictionary Items
- Python - Dictionary View Objects
- Python - Loop Dictionaries
- Python - Copy Dictionaries
- Python - Nested Dictionaries
- Python - Dictionary Methods
- Python - Dictionary Exercises
- Python Arrays
- Python - Arrays
- Python - Access Array Items
- Python - Add Array Items
- Python - Remove Array Items
- Python - Loop Arrays
- Python - Copy Arrays
- Python - Reverse Arrays
- Python - Sort Arrays
- Python - Join Arrays
- Python - Array Methods
- Python - Array Exercises
- Python File Handling
- Python - File Handling
- Python - Write to File
- Python - Read Files
- Python - Renaming and Deleting Files
- Python - Directories
- Python - File Methods
- Python - OS File/Directory Methods
- Python - OS Path Methods
- Object Oriented Programming
- Python - OOPs Concepts
- Python - Classes & Objects
- Python - Class Attributes
- Python - Class Methods
- Python - Static Methods
- Python - Constructors
- Python - Access Modifiers
- Python - Inheritance
- Python - Polymorphism
- Python - Method Overriding
- Python - Method Overloading
- Python - Dynamic Binding
- Python - Dynamic Typing
- Python - Abstraction
- Python - Encapsulation
- Python - Interfaces
- Python - Packages
- Python - Inner Classes
- Python - Anonymous Class and Objects
- Python - Singleton Class
- Python - Wrapper Classes
- Python - Enums
- Python - Reflection
- Python Errors & Exceptions
- Python - Syntax Errors
- Python - Exceptions
- Python - try-except Block
- Python - try-finally Block
- Python - Raising Exceptions
- Python - Exception Chaining
- Python - Nested try Block
- Python - User-defined Exception
- Python - Logging
- Python - Assertions
- Python - Built-in Exceptions
- Python Multithreading
- Python - Multithreading
- Python - Thread Life Cycle
- Python - Creating a Thread
- Python - Starting a Thread
- Python - Joining Threads
- Python - Naming Thread
- Python - Thread Scheduling
- Python - Thread Pools
- Python - Main Thread
- Python - Thread Priority
- Python - Daemon Threads
- Python - Synchronizing Threads
- Python Synchronization
- Python - Inter-thread Communication
- Python - Thread Deadlock
- Python - Interrupting a Thread
- Python Networking
- Python - Networking
- Python - Socket Programming
- Python - URL Processing
- Python - Generics
- Python Libraries
- NumPy Tutorial
- Pandas Tutorial
- SciPy Tutorial
- Matplotlib Tutorial
- Django Tutorial
- OpenCV Tutorial
- Python Miscellenous
- Python - Date & Time
- Python - Maths
- Python - Iterators
- Python - Generators
- Python - Closures
- Python - Decorators
- Python - Recursion
- Python - Reg Expressions
- Python - PIP
- Python - Database Access
- Python - Weak References
- Python - Serialization
- Python - Templating
- Python - Output Formatting
- Python - Performance Measurement
- Python - Data Compression
- Python - CGI Programming
- Python - XML Processing
- Python - GUI Programming
- Python - Command-Line Arguments
- Python - Docstrings
- Python - JSON
- Python - Sending Email
- Python - Further Extensions
- Python - Tools/Utilities
- Python - GUIs
- Python Useful Resources
- Python Compiler
- NumPy Compiler
- Matplotlib Compiler
- SciPy Compiler
- Python - Questions & Answers
- Python - Online Quiz
- Python - Programming Examples
- Python - Quick Guide
- Python - Useful Resources
- Python - Discussion
Python - Nested Dictionaries
Nested Dictionaries
Nested dictionaries in Python refer to dictionaries that are stored as values within another dictionary. In other words, a dictionary can contain other dictionaries as its values, forming a hierarchical or nested structure.
Nested dictionaries can be modified, updated, or extended in the same way as regular dictionaries. You can add, remove, or update key-value pairs at any level of the nested structure.
Creating a Nested Dictionary in Python
We can create a nested dictionary in Python by defining a dictionary where the values of certain keys are themselves dictionaries. This allows for the creation of a hierarchical structure where each key-value pair represents a level of nested information. This can be achieved in several ways −
Example: Direct Assignment
In this approach, we can directly assign dictionaries as values to outer keys within a single dictionary definition −
# Define the outer dictionary nested_dict = { "outer_key1": {"inner_key1": "value1", "inner_key2": "value2"}, "outer_key2": {"inner_key3": "value3", "inner_key4": "value4"} } print(nested_dict)
Example: Using a Loop
With this method, an empty outer dictionary is initialized, and then populated with dictionaries as values using a loop to define nested dictionaries −
# Define an empty outer dictionary nested_dict = {} # Add key-value pairs to the outer dictionary outer_keys = ["outer_key1", "outer_key2"] for key in outer_keys: nested_dict[key] = {"inner_key1": "value1", "inner_key2": "value2"} print(nested_dict)
Adding Items to a Nested Dictionary in Python
Once a nested dictionary is created, we can add items to it by accessing the specific nested dictionary using its key and then assigning a new key-value pair to it.
Example
In the following example, we are defining a nested dictionary "students" where each key represents a student's name and its value is another dictionary containing details about the student.
Then, we add a new key-value pair to Alice's nested dictionary and add a new nested dictionary for a new student, Charlie −
# Initial nested dictionary students = { "Alice": {"age": 21, "major": "Computer Science"}, "Bob": {"age": 20, "major": "Engineering"} } # Adding a new key-value pair to Alice's nested dictionary students["Alice"]["GPA"] = 3.8 # Adding a new nested dictionary for a new student students["Charlie"] = {"age": 22, "major": "Mathematics"} print(students)
It will produce the following output −
{'Alice': {'age': 21, 'major': 'Computer Science', 'GPA': 3.8}, 'Bob': {'age': 20, 'major': 'Engineering'}, 'Charlie': {'age': 22, 'major': 'Mathematics'}}
Accessing Items of a Nested Dictionary in Python
Accessing items of a nested dictionary in Python refers to retrieving values stored within the nested structure by using a series of keys. Each key corresponds to a level in the hierarchy of the dictionary.
We can achieve this through direct indexing with square brackets or by using the get() method
Example: Using Direct Indexing
In this approach, we access values in a nested dictionary by specifying each key in a sequence of square brackets. Each key in the sequence refers to a level in the nested dictionary, progressing one level deeper with each key −
# Define a nested dictionary students = { "Alice": {"age": 21, "major": "Computer Science"}, "Bob": {"age": 20, "major": "Engineering"}, "Charlie": {"age": 22, "major": "Mathematics"} } # Access Alice's major alice_major = students["Alice"]["major"] print("Alice's major:", alice_major) # Access Bob's age bob_age = students["Bob"]["age"] print("Bob's age:", bob_age)
Following is the output of the above code −
Alice's major: Computer Science Bob's age: 20
Example: Using the get() Method
The get() method is used to fetch the value associated with the specified key. If the key does not exist, it returns a default value (which is None if not specified) −
# Define a nested dictionary students = { "Alice": {"age": 21, "major": "Computer Science"}, "Bob": {"age": 20, "major": "Engineering"}, "Charlie": {"age": 22, "major": "Mathematics"} } # Access Alice's major using .get() alice_major = students.get("Alice", {}).get("major", "Not Found") print("Alice's major:", alice_major) # Safely access a non-existing key using .get() dave_major = students.get("Dave", {}).get("major", "Not Found") print("Dave's major:", dave_major)
Output of the above code is as follows −
Alice's major: Computer Science Dave's major: Not Found
Deleting a Dictionary from a Nested Dictionary
We can delete dictionaries from a nested dictionary by using the del keyword. This keyword allows us to remove a specific key-value pair from the nested dictionary.
Example
In the following example, we delete the nested dictionary for "Bob" from "students" dictionary using the del statement −
# Define a nested dictionary students = { "Alice": {"age": 21, "major": "Computer Science"}, "Bob": {"age": 20, "major": "Engineering"}, "Charlie": {"age": 22, "major": "Mathematics"} } # Delete the dictionary for Bob del students["Bob"] # Print the updated nested dictionary print(students)
We get the output as shown below −
{'Alice': {'age': 21, 'major': 'Computer Science'}, 'Charlie': {'age': 22, 'major': 'Mathematics'}}
Iterating Through a Nested Dictionary in Python
Iterating through a nested dictionary refers to looping through the keys and values at each level of the dictionary. This allows you to access and manipulate items within the nested structure.
We can iterate through a nested dictionary by using nested loops. The outer loop iterates over the keys and values of the main dictionary, while the inner loop iterates over the keys and values of the nested dictionaries.
Example
In this example, we are iterating through the "students" dictionary, retrieving each student's name and their corresponding details by iterating through the nested dictionaries −
# Defining a nested dictionary students = { "Alice": {"age": 21, "major": "Computer Science"}, "Bob": {"age": 20, "major": "Engineering"}, "Charlie": {"age": 22, "major": "Mathematics"} } # Iterating through the Nested Dictionary: for student, details in students.items(): print(f"Student: {student}") for key, value in details.items(): print(f" {key}: {value}")
The output obtained is as shown below −
Student: Alice age: 21 major: Computer Science Student: Bob age: 20 major: Engineering Student: Charlie age: 22 major: Mathematics