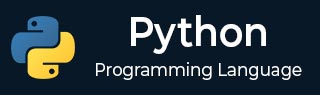
- Python Basics
- Python - Home
- Python - Overview
- Python - History
- Python - Features
- Python vs C++
- Python - Hello World Program
- Python - Application Areas
- Python - Interpreter
- Python - Environment Setup
- Python - Virtual Environment
- Python - Basic Syntax
- Python - Variables
- Python - Data Types
- Python - Type Casting
- Python - Unicode System
- Python - Literals
- Python - Operators
- Python - Arithmetic Operators
- Python - Comparison Operators
- Python - Assignment Operators
- Python - Logical Operators
- Python - Bitwise Operators
- Python - Membership Operators
- Python - Identity Operators
- Python - Operator Precedence
- Python - Comments
- Python - User Input
- Python - Numbers
- Python - Booleans
- Python Control Statements
- Python - Control Flow
- Python - Decision Making
- Python - If Statement
- Python - If else
- Python - Nested If
- Python - Match-Case Statement
- Python - Loops
- Python - for Loops
- Python - for-else Loops
- Python - While Loops
- Python - break Statement
- Python - continue Statement
- Python - pass Statement
- Python - Nested Loops
- Python Functions & Modules
- Python - Functions
- Python - Default Arguments
- Python - Keyword Arguments
- Python - Keyword-Only Arguments
- Python - Positional Arguments
- Python - Positional-Only Arguments
- Python - Arbitrary Arguments
- Python - Variables Scope
- Python - Function Annotations
- Python - Modules
- Python - Built in Functions
- Python Strings
- Python - Strings
- Python - Slicing Strings
- Python - Modify Strings
- Python - String Concatenation
- Python - String Formatting
- Python - Escape Characters
- Python - String Methods
- Python - String Exercises
- Python Lists
- Python - Lists
- Python - Access List Items
- Python - Change List Items
- Python - Add List Items
- Python - Remove List Items
- Python - Loop Lists
- Python - List Comprehension
- Python - Sort Lists
- Python - Copy Lists
- Python - Join Lists
- Python - List Methods
- Python - List Exercises
- Python Tuples
- Python - Tuples
- Python - Access Tuple Items
- Python - Update Tuples
- Python - Unpack Tuples
- Python - Loop Tuples
- Python - Join Tuples
- Python - Tuple Methods
- Python - Tuple Exercises
- Python Sets
- Python - Sets
- Python - Access Set Items
- Python - Add Set Items
- Python - Remove Set Items
- Python - Loop Sets
- Python - Join Sets
- Python - Copy Sets
- Python - Set Operators
- Python - Set Methods
- Python - Set Exercises
- Python Dictionaries
- Python - Dictionaries
- Python - Access Dictionary Items
- Python - Change Dictionary Items
- Python - Add Dictionary Items
- Python - Remove Dictionary Items
- Python - Dictionary View Objects
- Python - Loop Dictionaries
- Python - Copy Dictionaries
- Python - Nested Dictionaries
- Python - Dictionary Methods
- Python - Dictionary Exercises
- Python Arrays
- Python - Arrays
- Python - Access Array Items
- Python - Add Array Items
- Python - Remove Array Items
- Python - Loop Arrays
- Python - Copy Arrays
- Python - Reverse Arrays
- Python - Sort Arrays
- Python - Join Arrays
- Python - Array Methods
- Python - Array Exercises
- Python File Handling
- Python - File Handling
- Python - Write to File
- Python - Read Files
- Python - Renaming and Deleting Files
- Python - Directories
- Python - File Methods
- Python - OS File/Directory Methods
- Python - OS Path Methods
- Object Oriented Programming
- Python - OOPs Concepts
- Python - Classes & Objects
- Python - Class Attributes
- Python - Class Methods
- Python - Static Methods
- Python - Constructors
- Python - Access Modifiers
- Python - Inheritance
- Python - Polymorphism
- Python - Method Overriding
- Python - Method Overloading
- Python - Dynamic Binding
- Python - Dynamic Typing
- Python - Abstraction
- Python - Encapsulation
- Python - Interfaces
- Python - Packages
- Python - Inner Classes
- Python - Anonymous Class and Objects
- Python - Singleton Class
- Python - Wrapper Classes
- Python - Enums
- Python - Reflection
- Python Errors & Exceptions
- Python - Syntax Errors
- Python - Exceptions
- Python - try-except Block
- Python - try-finally Block
- Python - Raising Exceptions
- Python - Exception Chaining
- Python - Nested try Block
- Python - User-defined Exception
- Python - Logging
- Python - Assertions
- Python - Built-in Exceptions
- Python Multithreading
- Python - Multithreading
- Python - Thread Life Cycle
- Python - Creating a Thread
- Python - Starting a Thread
- Python - Joining Threads
- Python - Naming Thread
- Python - Thread Scheduling
- Python - Thread Pools
- Python - Main Thread
- Python - Thread Priority
- Python - Daemon Threads
- Python - Synchronizing Threads
- Python Synchronization
- Python - Inter-thread Communication
- Python - Thread Deadlock
- Python - Interrupting a Thread
- Python Networking
- Python - Networking
- Python - Socket Programming
- Python - URL Processing
- Python - Generics
- Python Libraries
- NumPy Tutorial
- Pandas Tutorial
- SciPy Tutorial
- Matplotlib Tutorial
- Django Tutorial
- OpenCV Tutorial
- Python Miscellenous
- Python - Date & Time
- Python - Maths
- Python - Iterators
- Python - Generators
- Python - Closures
- Python - Decorators
- Python - Recursion
- Python - Reg Expressions
- Python - PIP
- Python - Database Access
- Python - Weak References
- Python - Serialization
- Python - Templating
- Python - Output Formatting
- Python - Performance Measurement
- Python - Data Compression
- Python - CGI Programming
- Python - XML Processing
- Python - GUI Programming
- Python - Command-Line Arguments
- Python - Docstrings
- Python - JSON
- Python - Sending Email
- Python - Further Extensions
- Python - Tools/Utilities
- Python - GUIs
- Python Useful Resources
- Python Compiler
- NumPy Compiler
- Matplotlib Compiler
- SciPy Compiler
- Python - Questions & Answers
- Python - Online Quiz
- Python - Programming Examples
- Python - Quick Guide
- Python - Useful Resources
- Python - Discussion
Python - Join Lists
Join Lists in Python
Joining lists in Python refers to combining the elements of multiple lists into a single list. This can be achieved using various methods, such as concatenation, list comprehension, or using built-in functions like extend() or + operator.
Joining lists does not modify the original lists but creates a new list containing the combined elements.
Join Lists Using Concatenation Operator
The concatenation operator in Python, denoted by +, is used to join two sequences, such as strings, lists, or tuples, into a single sequence. When applied to lists, the concatenation operator joins the elements of the two (or more) lists to create a new list containing all the elements from both lists.
We can join a list using the concatenation operator by simply using the + symbol to concatenate the lists.
Example
In the following example, we are concatenating the elements of two lists "L1" and "L2", creating a new list "joined_list" containing all the elements from both lists −
# Two lists to be joined L1 = [10,20,30,40] L2 = ['one', 'two', 'three', 'four'] # Joining the lists joined_list = L1 + L2 # Printing the joined list print("Joined List:", joined_list)
Following is the output of the above code −
Joined List: [10, 20, 30, 40, 'one', 'two', 'three', 'four']
Join Lists Using List Comprehension
List comprehension is a concise way to create lists in Python. It is used to generate new lists by applying an expression to each item in an existing iterable, such as a list, tuple, or range. The syntax for list comprehension is −
new_list = [expression for item in iterable]
This creates a new list where expression is evaluated for each item in the iterable.
We can join a list using list comprehension by iterating over multiple lists and appending their elements to a new list.
Example
In this example, we are joining two lists, L1 and L2, into a single list using list comprehension. The resulting list, joined_list, contains all elements from both L1 and L2 −
# Two lists to be joined L1 = [36, 24, 3] L2 = [84, 5, 81] # Joining the lists using list comprehension joined_list = [item for sublist in [L1, L2] for item in sublist] # Printing the joined list print("Joined List:", joined_list)
Output of the above code is as follows −
Joined List: [36, 24, 3, 84, 5, 81]
Join Lists Using append() Function
The append() function in Python is used to add a single element to the end of a list. This function modifies the original list by adding the element to the end of the list.
We can join a list using the append() function by iterating over the elements of one list and appending each element to another list.
Example
In the example below, we are appending elements from "list2" to "list1" using the append() function. We achieve this by iterating over "list2" and adding each element to "list1" −
# List to which elements will be appended list1 = ['Fruit', 'Number', 'Animal'] # List from which elements will be appended list2 = ['Apple', 5, 'Dog'] # Joining the lists using the append() function for element in list2: list1.append(element) # Printing the joined list print("Joined List:", list1)
We get the output as shown below −
Joined List: ['Fruit', 'Number', 'Animal', 'Apple', 5, 'Dog']
Join Lists Using extend() Function
The Python extend() function is used to append elements from an iterable (such as another list) to the end of the list. This function modifies the original list in place, adding the elements of the iterable to the end of the list.
We can join a list using the extend() function by calling it on one list and passing another list (or any iterable) as an argument. This will append all the elements from the second list to the end of the first list.
Example
In the following example, we are extending "list1" by appending the elements of "list2" using the extend() function −
# List to be extended list1 = [10, 15, 20] # List to be added list2 = [25, 30, 35] # Joining the lists using the extend() function list1.extend(list2) # Printing the extended list print("Extended List:", list1)
The output obtained is as shown below −
Extended List: [10, 15, 20, 25, 30, 35]