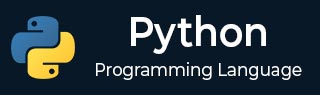
- Python Basics
- Python - Home
- Python - Overview
- Python - History
- Python - Features
- Python vs C++
- Python - Hello World Program
- Python - Application Areas
- Python - Interpreter
- Python - Environment Setup
- Python - Virtual Environment
- Python - Basic Syntax
- Python - Variables
- Python - Data Types
- Python - Type Casting
- Python - Unicode System
- Python - Literals
- Python - Operators
- Python - Arithmetic Operators
- Python - Comparison Operators
- Python - Assignment Operators
- Python - Logical Operators
- Python - Bitwise Operators
- Python - Membership Operators
- Python - Identity Operators
- Python - Operator Precedence
- Python - Comments
- Python - User Input
- Python - Numbers
- Python - Booleans
- Python Control Statements
- Python - Control Flow
- Python - Decision Making
- Python - If Statement
- Python - If else
- Python - Nested If
- Python - Match-Case Statement
- Python - Loops
- Python - for Loops
- Python - for-else Loops
- Python - While Loops
- Python - break Statement
- Python - continue Statement
- Python - pass Statement
- Python - Nested Loops
- Python Functions & Modules
- Python - Functions
- Python - Default Arguments
- Python - Keyword Arguments
- Python - Keyword-Only Arguments
- Python - Positional Arguments
- Python - Positional-Only Arguments
- Python - Arbitrary Arguments
- Python - Variables Scope
- Python - Function Annotations
- Python - Modules
- Python - Built in Functions
- Python Strings
- Python - Strings
- Python - Slicing Strings
- Python - Modify Strings
- Python - String Concatenation
- Python - String Formatting
- Python - Escape Characters
- Python - String Methods
- Python - String Exercises
- Python Lists
- Python - Lists
- Python - Access List Items
- Python - Change List Items
- Python - Add List Items
- Python - Remove List Items
- Python - Loop Lists
- Python - List Comprehension
- Python - Sort Lists
- Python - Copy Lists
- Python - Join Lists
- Python - List Methods
- Python - List Exercises
- Python Tuples
- Python - Tuples
- Python - Access Tuple Items
- Python - Update Tuples
- Python - Unpack Tuples
- Python - Loop Tuples
- Python - Join Tuples
- Python - Tuple Methods
- Python - Tuple Exercises
- Python Sets
- Python - Sets
- Python - Access Set Items
- Python - Add Set Items
- Python - Remove Set Items
- Python - Loop Sets
- Python - Join Sets
- Python - Copy Sets
- Python - Set Operators
- Python - Set Methods
- Python - Set Exercises
- Python Dictionaries
- Python - Dictionaries
- Python - Access Dictionary Items
- Python - Change Dictionary Items
- Python - Add Dictionary Items
- Python - Remove Dictionary Items
- Python - Dictionary View Objects
- Python - Loop Dictionaries
- Python - Copy Dictionaries
- Python - Nested Dictionaries
- Python - Dictionary Methods
- Python - Dictionary Exercises
- Python Arrays
- Python - Arrays
- Python - Access Array Items
- Python - Add Array Items
- Python - Remove Array Items
- Python - Loop Arrays
- Python - Copy Arrays
- Python - Reverse Arrays
- Python - Sort Arrays
- Python - Join Arrays
- Python - Array Methods
- Python - Array Exercises
- Python File Handling
- Python - File Handling
- Python - Write to File
- Python - Read Files
- Python - Renaming and Deleting Files
- Python - Directories
- Python - File Methods
- Python - OS File/Directory Methods
- Python - OS Path Methods
- Object Oriented Programming
- Python - OOPs Concepts
- Python - Classes & Objects
- Python - Class Attributes
- Python - Class Methods
- Python - Static Methods
- Python - Constructors
- Python - Access Modifiers
- Python - Inheritance
- Python - Polymorphism
- Python - Method Overriding
- Python - Method Overloading
- Python - Dynamic Binding
- Python - Dynamic Typing
- Python - Abstraction
- Python - Encapsulation
- Python - Interfaces
- Python - Packages
- Python - Inner Classes
- Python - Anonymous Class and Objects
- Python - Singleton Class
- Python - Wrapper Classes
- Python - Enums
- Python - Reflection
- Python Errors & Exceptions
- Python - Syntax Errors
- Python - Exceptions
- Python - try-except Block
- Python - try-finally Block
- Python - Raising Exceptions
- Python - Exception Chaining
- Python - Nested try Block
- Python - User-defined Exception
- Python - Logging
- Python - Assertions
- Python - Built-in Exceptions
- Python Multithreading
- Python - Multithreading
- Python - Thread Life Cycle
- Python - Creating a Thread
- Python - Starting a Thread
- Python - Joining Threads
- Python - Naming Thread
- Python - Thread Scheduling
- Python - Thread Pools
- Python - Main Thread
- Python - Thread Priority
- Python - Daemon Threads
- Python - Synchronizing Threads
- Python Synchronization
- Python - Inter-thread Communication
- Python - Thread Deadlock
- Python - Interrupting a Thread
- Python Networking
- Python - Networking
- Python - Socket Programming
- Python - URL Processing
- Python - Generics
- Python Libraries
- NumPy Tutorial
- Pandas Tutorial
- SciPy Tutorial
- Matplotlib Tutorial
- Django Tutorial
- OpenCV Tutorial
- Python Miscellenous
- Python - Date & Time
- Python - Maths
- Python - Iterators
- Python - Generators
- Python - Closures
- Python - Decorators
- Python - Recursion
- Python - Reg Expressions
- Python - PIP
- Python - Database Access
- Python - Weak References
- Python - Serialization
- Python - Templating
- Python - Output Formatting
- Python - Performance Measurement
- Python - Data Compression
- Python - CGI Programming
- Python - XML Processing
- Python - GUI Programming
- Python - Command-Line Arguments
- Python - Docstrings
- Python - JSON
- Python - Sending Email
- Python - Further Extensions
- Python - Tools/Utilities
- Python - GUIs
- Python Useful Resources
- Python Compiler
- NumPy Compiler
- Matplotlib Compiler
- SciPy Compiler
- Python - Questions & Answers
- Python - Online Quiz
- Python - Programming Examples
- Python - Quick Guide
- Python - Useful Resources
- Python - Discussion
Python - Directories
Directories in Python
In Python, directories, commonly known as folders in operating systems, are locations on the filesystem used to store files and other directories. They serve as a way to group and manage files hierarchically.
Python provides several modules, primarily os and os.path, along with shutil, that allows you to perform various operations on directories.
These operations include creating new directories, navigating through existing directories, listing directory contents, changing the current working directory, and removing directories.
Checking if a Directory Exists
Before performing operations on a directory, you often need to check if it exists. We can check if a directory exists or not using the os.path.exists() function in Python.
This function accepts a single argument, which is a string representing a path in the filesystem. This argument can be −
Relative path − A path relative to the current working directory.
Absolute path − A complete path starting from the root directory.
Example
In this example, we check whether the given directory path exists using the os.path.exists() function −
import os directory_path = "D:\\Test\\MyFolder\\" if os.path.exists(directory_path): print(f"The directory '{directory_path}' exists.") else: print(f"The directory '{directory_path}' does not exist.")
Following is the output of the above code −
The directory 'D:\\Test\\MyFolder\\' exists.
Creating a Directory
You create a new directory in Python using the os.makedirs() function. This function creates intermediate directories if they do not exist.
The os.makedirs() function accepts a "path" you want to create as an argument. It optionally accepts a "mode" argument that specifies the permissions o set for the newly created directories. It is an integer, represented in octal format (e.g., 0o755). If not specified, the default permissions are used based on your system's umask.
Example
In the following example, we are creating a new directory using the os.makedirs() function −
import os new_directory = "new_dir.txt" try: os.makedirs(new_directory) print(f"Directory '{new_directory}' created successfully.") except OSError as e: print(f"Error: Failed to create directory '{new_directory}'. {e}")
After executing the above code, we get the following output −
Directory 'new_dir.txt' created successfully.
The mkdir() Method
You can use the mkdir() method of the os module to create directories in the current directory. You need to supply an argument to this method, which contains the name of the directory to be created.
Following is the syntax of the mkdir() method in Python −
os.mkdir("newdir")
Example
Following is an example to create a directory test in the current directory −
import os # Create a directory "test" os.mkdir("test") print ("Directory created successfully")
The result obtained is as shown below −
Directory created successfully
Get Current Working Directory
To retrieve the current working directory in Python, you can use the os.getcwd() function. This function returns a string representing the current working directory where the Python script is executing.
Syntax
Following is the basic syntax of the getcwd() function in Python −
os.getcwd()
Example
Following is an example to display the current working directory using the getcwd() function −
import os current_directory = os.getcwd() print(f"Current working directory: {current_directory}")
We get the output as follows −
Current working directory: /home/cg/root/667ba7570a5b7
Listing Files and Directories
You can list the contents of a directory using the os.listdir() function. This function returns a list of all files and directories within the specified directory path.
Example
In the example below, we are listing the contents of the specified directory path using the listdir() function −
import os directory_path = r"D:\MyFolder\Pictures" try: contents = os.listdir(directory_path) print(f"Contents of '{directory_path}':") for item in contents: print(item) except OSError as e: print(f"Error: Failed to list contents of directory '{directory_path}'. {e}")
Output of the above code is as shown below −
Contents of 'D:\MyFolder\Pictures': Camera Roll desktop.ini Saved Pictures Screenshots
Changing the Current Working Directory
You can change the current directory using the chdir() method. This method takes an argument, which is the name of the directory that you want to make the current directory.
Syntax
Following is the syntax of the chdir() method in Python −
os.chdir("newdir")
Example
Following is an example to change the current directory to Desktop using the chdir() method −
import os new_directory = r"D:\MyFolder\Pictures" try: os.chdir(new_directory) print(f"Current working directory changed to '{new_directory}'.") except OSError as e: print(f"Error: Failed to change working directory to '{new_directory}'. {e}")
We get the output as shown below −
Current working directory changed to 'D:\MyFolder\Pictures'.
Removing a Directory
You can remove an empty directory in Python using the os.rmdir() method. If the directory contains files or other directories, you can use shutil.rmtree() method to delete it recursively.
Syntax
Following is the basic syntax to delete a directory in Python −
os.rmdir(directory_path) # or shutil.rmtree(directory_path)
Example
In the following example, we remove an empty directory using the os.rmdir() method −
import os directory_path = r"D:\MyFolder\new_dir" try: os.rmdir(directory_path) print(f"Directory '{directory_path}' successfully removed.") except OSError as e: print(f"Error: Failed to remove directory '{directory_path}'. {e}")
It will produce the following output −
Directory 'D:\MyFolder\new_dir' successfully removed.