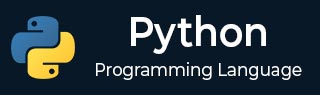
- Python Basics
- Python - Home
- Python - Overview
- Python - History
- Python - Features
- Python vs C++
- Python - Hello World Program
- Python - Application Areas
- Python - Interpreter
- Python - Environment Setup
- Python - Virtual Environment
- Python - Basic Syntax
- Python - Variables
- Python - Data Types
- Python - Type Casting
- Python - Unicode System
- Python - Literals
- Python - Operators
- Python - Arithmetic Operators
- Python - Comparison Operators
- Python - Assignment Operators
- Python - Logical Operators
- Python - Bitwise Operators
- Python - Membership Operators
- Python - Identity Operators
- Python - Operator Precedence
- Python - Comments
- Python - User Input
- Python - Numbers
- Python - Booleans
- Python Control Statements
- Python - Control Flow
- Python - Decision Making
- Python - If Statement
- Python - If else
- Python - Nested If
- Python - Match-Case Statement
- Python - Loops
- Python - for Loops
- Python - for-else Loops
- Python - While Loops
- Python - break Statement
- Python - continue Statement
- Python - pass Statement
- Python - Nested Loops
- Python Functions & Modules
- Python - Functions
- Python - Default Arguments
- Python - Keyword Arguments
- Python - Keyword-Only Arguments
- Python - Positional Arguments
- Python - Positional-Only Arguments
- Python - Arbitrary Arguments
- Python - Variables Scope
- Python - Function Annotations
- Python - Modules
- Python - Built in Functions
- Python Strings
- Python - Strings
- Python - Slicing Strings
- Python - Modify Strings
- Python - String Concatenation
- Python - String Formatting
- Python - Escape Characters
- Python - String Methods
- Python - String Exercises
- Python Lists
- Python - Lists
- Python - Access List Items
- Python - Change List Items
- Python - Add List Items
- Python - Remove List Items
- Python - Loop Lists
- Python - List Comprehension
- Python - Sort Lists
- Python - Copy Lists
- Python - Join Lists
- Python - List Methods
- Python - List Exercises
- Python Tuples
- Python - Tuples
- Python - Access Tuple Items
- Python - Update Tuples
- Python - Unpack Tuples
- Python - Loop Tuples
- Python - Join Tuples
- Python - Tuple Methods
- Python - Tuple Exercises
- Python Sets
- Python - Sets
- Python - Access Set Items
- Python - Add Set Items
- Python - Remove Set Items
- Python - Loop Sets
- Python - Join Sets
- Python - Copy Sets
- Python - Set Operators
- Python - Set Methods
- Python - Set Exercises
- Python Dictionaries
- Python - Dictionaries
- Python - Access Dictionary Items
- Python - Change Dictionary Items
- Python - Add Dictionary Items
- Python - Remove Dictionary Items
- Python - Dictionary View Objects
- Python - Loop Dictionaries
- Python - Copy Dictionaries
- Python - Nested Dictionaries
- Python - Dictionary Methods
- Python - Dictionary Exercises
- Python Arrays
- Python - Arrays
- Python - Access Array Items
- Python - Add Array Items
- Python - Remove Array Items
- Python - Loop Arrays
- Python - Copy Arrays
- Python - Reverse Arrays
- Python - Sort Arrays
- Python - Join Arrays
- Python - Array Methods
- Python - Array Exercises
- Python File Handling
- Python - File Handling
- Python - Write to File
- Python - Read Files
- Python - Renaming and Deleting Files
- Python - Directories
- Python - File Methods
- Python - OS File/Directory Methods
- Python - OS Path Methods
- Object Oriented Programming
- Python - OOPs Concepts
- Python - Classes & Objects
- Python - Class Attributes
- Python - Class Methods
- Python - Static Methods
- Python - Constructors
- Python - Access Modifiers
- Python - Inheritance
- Python - Polymorphism
- Python - Method Overriding
- Python - Method Overloading
- Python - Dynamic Binding
- Python - Dynamic Typing
- Python - Abstraction
- Python - Encapsulation
- Python - Interfaces
- Python - Packages
- Python - Inner Classes
- Python - Anonymous Class and Objects
- Python - Singleton Class
- Python - Wrapper Classes
- Python - Enums
- Python - Reflection
- Python Errors & Exceptions
- Python - Syntax Errors
- Python - Exceptions
- Python - try-except Block
- Python - try-finally Block
- Python - Raising Exceptions
- Python - Exception Chaining
- Python - Nested try Block
- Python - User-defined Exception
- Python - Logging
- Python - Assertions
- Python - Built-in Exceptions
- Python Multithreading
- Python - Multithreading
- Python - Thread Life Cycle
- Python - Creating a Thread
- Python - Starting a Thread
- Python - Joining Threads
- Python - Naming Thread
- Python - Thread Scheduling
- Python - Thread Pools
- Python - Main Thread
- Python - Thread Priority
- Python - Daemon Threads
- Python - Synchronizing Threads
- Python Synchronization
- Python - Inter-thread Communication
- Python - Thread Deadlock
- Python - Interrupting a Thread
- Python Networking
- Python - Networking
- Python - Socket Programming
- Python - URL Processing
- Python - Generics
- Python Libraries
- NumPy Tutorial
- Pandas Tutorial
- SciPy Tutorial
- Matplotlib Tutorial
- Django Tutorial
- OpenCV Tutorial
- Python Miscellenous
- Python - Date & Time
- Python - Maths
- Python - Iterators
- Python - Generators
- Python - Closures
- Python - Decorators
- Python - Recursion
- Python - Reg Expressions
- Python - PIP
- Python - Database Access
- Python - Weak References
- Python - Serialization
- Python - Templating
- Python - Output Formatting
- Python - Performance Measurement
- Python - Data Compression
- Python - CGI Programming
- Python - XML Processing
- Python - GUI Programming
- Python - Command-Line Arguments
- Python - Docstrings
- Python - JSON
- Python - Sending Email
- Python - Further Extensions
- Python - Tools/Utilities
- Python - GUIs
- Python Useful Resources
- Python Compiler
- NumPy Compiler
- Matplotlib Compiler
- SciPy Compiler
- Python - Questions & Answers
- Python - Online Quiz
- Python - Programming Examples
- Python - Quick Guide
- Python - Useful Resources
- Python - Discussion
Python - Built-in Functions
Built-in Functions in Python?
Built-in functions are those functions that are pre-defined in the Python interpreter and you don't need to import any module to use them. These functions help to perform a wide variety of operations on strings, iterators, and numbers. For instance, the built-in functions like sum(), min(), and max() are used to simplify mathematical operations.
How to Use Built-in Function in Python?
To use built-in functions in your code, simply call the specific function by passing the required parameter (if any) inside the parentheses. Since these functions are pre-defined, you don't need to import any module or package.
Example of Using Built-in Functions
Consider the following example demonstrating the use of built-in functions in your code:
# Using print() and len() function text = "Tutorials Point" print(len(text)) # Prints 15
In the above example, we are using two built-in functions print() and len().
List of Python Built-in Functions
As of Python 3.12.2 version, the list of built-in functions is given below −
Sr.No. | Function & Description |
---|---|
1 |
Returns an asynchronous iterator for an asynchronous iterable. |
2 |
Returns true when all elements in iterable is true. |
3 |
Returns the next item from the given asynchronous iterator. |
4 |
Checks if any Element of an Iterable is True. |
5 |
Returns String Containing Printable Representation. |
6 |
Converts integer to binary string. |
7 |
Converts a Value to Boolean. |
8 |
This function drops you into the debugger at the call site and calls sys.breakpointhook(). |
9 |
Returns array of given byte size. |
10 |
Returns immutable bytes object. |
11 |
Checks if the Object is Callable. |
12 |
Returns a Character (a string) from an Integer. |
13 |
Returns class method for given function. |
14 |
Returns a code object. |
15 |
Creates a Complex Number. |
16 |
Deletes Attribute From the Object. |
17 |
Creates a Dictionary. |
18 |
Tries to Return Attributes of Object. |
19 |
Returns a Tuple of Quotient and Remainder. |
20 |
Returns an Enumerate Object. |
21 |
Runs Code Within Program. |
22 |
Executes Dynamically Created Program. |
23 |
Constructs iterator from elements which are true. |
24 |
Returns floating point number from number, string. |
25 |
Returns formatted representation of a value. |
26 |
Returns immutable frozenset object. |
27 |
Returns value of named attribute of an object. |
28 |
Returns dictionary of current global symbol table. |
29 |
Returns whether object has named attribute. |
30 |
Returns hash value of an object. |
31 |
Invokes the built-in Help System. |
32 |
Converts to Integer to Hexadecimal. |
33 |
Returns Identify of an Object. |
34 |
Reads and returns a line of string. |
35 |
Returns integer from a number or string. |
36 |
Checks if a Object is an Instance of Class. |
37 |
Checks if a Class is Subclass of another Class. |
38 |
Returns an iterator. |
39 |
Returns Length of an Object. |
40 |
Creates a list in Python. |
41 |
Returns dictionary of a current local symbol table. |
42 |
Applies Function and Returns a List. |
43 |
Returns memory view of an argument. |
44 |
Retrieves next item from the iterator. |
45 |
Creates a featureless object. |
46 |
Returns the octal representation of an integer. |
47 |
Returns a file object. |
48 |
Returns an integer of the Unicode character. |
49 |
Prints the Given Object. |
50 |
Returns the property attribute. |
51 |
Returns a sequence of integers. |
52 |
Returns a printable representation of the object. |
53 |
Returns the reversed iterator of a sequence. |
54 |
Constructs and returns a set. |
55 |
Sets the value of an attribute of an object. |
56 |
Returns a slice object. |
57 |
Returns a sorted list from the given iterable. |
58 |
Python staticmethod() function Transforms a method into a static method. |
59 |
Returns the string version of the object. |
60 |
Returns a proxy object of the base class. |
61 |
Returns a tuple. |
62 |
Returns the type of the object. |
63 |
Returns the __dict__ attribute. |
64 |
Returns an iterator of tuples. |
65 |
Function called by the import statement. |
66 |
Converts a Unicode code point to its corresponding Unicode character. |
67 |
Represents integers of arbitrary size. |
Built-in Mathematical Functions
There are some additional built-in functions that are used for performing only mathematical operations in Python, they are listed below −
Sr.No. | Function & Description |
---|---|
1 |
The abs() function returns the absolute value of x, i.e. the positive distance between x and zero. |
2 |
The max() function returns the largest of its arguments or largest number from the iterable (list or tuple). |
3 |
The function min() returns the smallest of its arguments i.e. the value closest to negative infinity, or smallest number from the iterable (list or tuple) |
4 |
The pow() function returns x raised to y. It is equivalent to x**y. The function has third optional argument mod. If given, it returns (x**y) % mod value |
5 |
round() is a built-in function in Python. It returns x rounded to n digits from the decimal point. |
6 |
The sum() function returns the sum of all numeric items in any iterable (list or tuple). An optional start argument is 0 by default. If given, the numbers in the list are added to start value. |
Advantages of Using Built-in Functions
The following are the advantages of using built-in functions:
- The use of the built-in functions simplifies and reduces the code length and enhances the readability of the code.
- Instead of writing the same logic repeatedly, you can use these functions across different sections of the program. This not only saves time but also helps in maintaining consistency of code.
- These functions provide a wide range of functionalities including mathematical operations, datatype conversion, and performing operations on iterators.
- These functions have descriptive names that make the code easier to understand and maintain. Developers need not write additional complex code for performing certain operations.
Frequently Asked Questions about Built-in Functions
How do I handle errors with built-in functions?
While working with built-in functions, you may encounter errors and to handle those errors you can use the try-except blocks. This may help you identify the type of error and exceptions raised.
Can we extend the functionality of built-in functions?
Yes, we can extend the functionality of built-in functions by using it with other methods and by applying your logic as per the need. However, it will not affect the pre-defined feature of the used function.
Can I create my built-in functions?
No, you cannot create your built-in function. But, Python allows a user to create user-defined functions.
How do I use built-in functions?
Using a built-in function is very simple, call it by its name followed by parentheses, and pass the required arguments inside the parentheses.