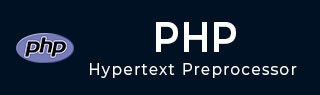
- PHP Tutorial
- PHP - Home
- PHP - Introduction
- PHP - Installation
- PHP - History
- PHP - Features
- PHP - Syntax
- PHP - Hello World
- PHP - Comments
- PHP - Variables
- PHP - Echo/Print
- PHP - var_dump
- PHP - $ and $$ Variables
- PHP - Constants
- PHP - Magic Constants
- PHP - Data Types
- PHP - Type Casting
- PHP - Type Juggling
- PHP - Strings
- PHP - Boolean
- PHP - Integers
- PHP - Files & I/O
- PHP - Maths Functions
- PHP - Heredoc & Nowdoc
- PHP - Compound Types
- PHP - File Include
- PHP - Date & Time
- PHP - Scalar Type Declarations
- PHP - Return Type Declarations
- PHP Operators
- PHP - Operators
- PHP - Arithmatic Operators
- PHP - Comparison Operators
- PHP - Logical Operators
- PHP - Assignment Operators
- PHP - String Operators
- PHP - Array Operators
- PHP - Conditional Operators
- PHP - Spread Operator
- PHP - Null Coalescing Operator
- PHP - Spaceship Operator
- PHP Control Statements
- PHP - Decision Making
- PHP - If…Else Statement
- PHP - Switch Statement
- PHP - Loop Types
- PHP - For Loop
- PHP - Foreach Loop
- PHP - While Loop
- PHP - Do…While Loop
- PHP - Break Statement
- PHP - Continue Statement
- PHP Arrays
- PHP - Arrays
- PHP - Indexed Array
- PHP - Associative Array
- PHP - Multidimensional Array
- PHP - Array Functions
- PHP - Constant Arrays
- PHP Functions
- PHP - Functions
- PHP - Function Parameters
- PHP - Call by value
- PHP - Call by Reference
- PHP - Default Arguments
- PHP - Named Arguments
- PHP - Variable Arguments
- PHP - Returning Values
- PHP - Passing Functions
- PHP - Recursive Functions
- PHP - Type Hints
- PHP - Variable Scope
- PHP - Strict Typing
- PHP - Anonymous Functions
- PHP - Arrow Functions
- PHP - Variable Functions
- PHP - Local Variables
- PHP - Global Variables
- PHP Superglobals
- PHP - Superglobals
- PHP - $GLOBALS
- PHP - $_SERVER
- PHP - $_REQUEST
- PHP - $_POST
- PHP - $_GET
- PHP - $_FILES
- PHP - $_ENV
- PHP - $_COOKIE
- PHP - $_SESSION
- PHP File Handling
- PHP - File Handling
- PHP - Open File
- PHP - Read File
- PHP - Write File
- PHP - File Existence
- PHP - Download File
- PHP - Copy File
- PHP - Append File
- PHP - Delete File
- PHP - Handle CSV File
- PHP - File Permissions
- PHP - Create Directory
- PHP - Listing Files
- Object Oriented PHP
- PHP - Object Oriented Programming
- PHP - Classes and Objects
- PHP - Constructor and Destructor
- PHP - Access Modifiers
- PHP - Inheritance
- PHP - Class Constants
- PHP - Abstract Classes
- PHP - Interfaces
- PHP - Traits
- PHP - Static Methods
- PHP - Static Properties
- PHP - Namespaces
- PHP - Object Iteration
- PHP - Encapsulation
- PHP - Final Keyword
- PHP - Overloading
- PHP - Cloning Objects
- PHP - Anonymous Classes
- PHP Web Development
- PHP - Web Concepts
- PHP - Form Handling
- PHP - Form Validation
- PHP - Form Email/URL
- PHP - Complete Form
- PHP - File Inclusion
- PHP - GET & POST
- PHP - File Uploading
- PHP - Cookies
- PHP - Sessions
- PHP - Session Options
- PHP - Sending Emails
- PHP - Sanitize Input
- PHP - Post-Redirect-Get (PRG)
- PHP - Flash Messages
- PHP AJAX
- PHP - AJAX Introduction
- PHP - AJAX Search
- PHP - AJAX XML Parser
- PHP - AJAX Auto Complete Search
- PHP - AJAX RSS Feed Example
- PHP XML
- PHP - XML Introduction
- PHP - Simple XML Parser
- PHP - SAX Parser Example
- PHP - DOM Parser Example
- PHP Login Example
- PHP - Login Example
- PHP - Facebook Login
- PHP - Paypal Integration
- PHP - MySQL Login
- PHP Advanced
- PHP - MySQL
- PHP.INI File Configuration
- PHP - Array Destructuring
- PHP - Coding Standard
- PHP - Regular Expression
- PHP - Error Handling
- PHP - Try…Catch
- PHP - Bugs Debugging
- PHP - For C Developers
- PHP - For PERL Developers
- PHP - Frameworks
- PHP - Core PHP vs Frame Works
- PHP - Design Patterns
- PHP - Filters
- PHP - JSON
- PHP - Exceptions
- PHP - Special Types
- PHP - Hashing
- PHP - Encryption
- PHP - is_null() Function
- PHP - System Calls
- PHP - HTTP Authentication
- PHP - Swapping Variables
- PHP - Closure::call()
- PHP - Filtered unserialize()
- PHP - IntlChar
- PHP - CSPRNG
- PHP - Expectations
- PHP - Use Statement
- PHP - Integer Division
- PHP - Deprecated Features
- PHP - Removed Extensions & SAPIs
- PHP - PEAR
- PHP - CSRF
- PHP - FastCGI Process
- PHP - PDO Extension
- PHP - Built-In Functions
- PHP Useful Resources
- PHP - Questions & Answers
- PHP - Quick Guide
- PHP - Useful Resources
- PHP - Discussion
PHP - Strict Typing
PHP is widely regarded as a weakly typed language. In PHP, you need not declare the type of a variable before assigning it any value. The PHP parser tries to cast the variables into compatible type as far as possible.
For example, if one of the values passed is a string representation of a number, and the second is a numeric variable, PHP casts the string variable to numeric in order to perform the addition operation.
Example
Take a look at the following example −
<?php function addition($x, $y) { echo "First number: $x Second number: $y Addition: " . $x+$y; } $x="10"; $y=20; addition($x, $y); ?>
It will produce the following output −
First number: 10 Second number: 20 Addition: 30
However, if $x in the above example is a string that doesn’t hold a valid numeric representation, then you will encounter an error.
<?php function addition($x, $y) { echo "First number: $x Second number: $y Addition: " . $x+$y; } $x="Hello"; $y=20; addition($x, $y); ?>
It will produce the following output −
PHP Fatal error: Uncaught TypeError: Unsupported operand types: string + int in hello.php:5
Type Hints
Type-hinting is supported from PHP 5.6 version onwards. It means you can explicitly state the expected type of a variable declared in your code. PHP allows you to type-hint function arguments, return values, and class properties. With this, it is possible to write more robust code.
Let us incorporate type-hinting in the addition function in the above program −
function addition(int $x, int $y) { echo "First number: $x Second number: $y Addition: " . $x+$y; }
Note that by merely using the data types in the variable declarations doesn’t prevent the unmatched type exception raised, as PHP is a dynamically typed language. In other words, $x="10" and $y=20 will still result in the addition as 30, whereas $x="Hello" makes the parser raise the error.
Example
<?php function addition($x, $y) { echo "First number: $x \n"; echo "Second number: $y \n"; echo "Addition: " . $x+$y . "\n\n"; } $x=10; $y=20; addition($x, $y); $x="10"; $y=20; addition($x, $y); $x="Hello"; $y=20; addition($x, $y); ?>
It will produce the following output −
First number: 10 Second number: 20 Addition: 30 First number: 10 Second number: 20 Addition: 30 First number: Hello Second number: 20 PHP Fatal error: Uncaught TypeError: Unsupported operand types: string + int in hello.php:5
strict_types
PHP can be made to impose stricter rules for type conversion, so that "10" is not implicitly converted to 10. This can be enforced by setting strict_types directive to 1 in a declare() statement.
The declare() statement must be the first statement in the PHP code, just after the "<?php" tag.
Example
Take a look at the following example −
<?php declare (strict_types=1); function addition(int $x, int $y) { echo "First number: $x Second number: $y Addition: " . $x+$y; } $x=10; $y=20; addition($x, $y); ?>
It will produce the following output −
First number: 10 Second number: 20 Addition: 30
Now, if $x is set to "10", the implicit conversion won't take place, resulting in the following error −
PHP Fatal error: Uncaught TypeError: addition(): Argument #1 ($x) must be of type int, string given
From PHP 7 onwards, type-hinting support has been extended for function returns to prevent unexpected return values. You can type-hint the return values by adding the intended type after the parameter list prefixed with a colon (:) symbol.
Example
Let us add a type hint to the return value of the division() function below.
<?php declare (strict_types=1); function division(int $x, int $y) : int { return $x/$y; } $x=10; $y=20; $result = division($x, $y); echo "First number: $x Second number: $y Addition: " . $result; ?>
Because the function returns 0.5, which is not of int type (that is, the type hint used for the return value of the function), the following error is displayed −
Fatal error: Uncaught TypeError: division(): Return value must be of type int, float returned in hello.php:5