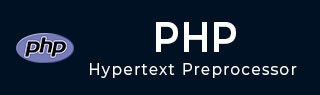
- PHP Tutorial
- PHP - Home
- PHP - Introduction
- PHP - Installation
- PHP - History
- PHP - Features
- PHP - Syntax
- PHP - Hello World
- PHP - Comments
- PHP - Variables
- PHP - Echo/Print
- PHP - var_dump
- PHP - $ and $$ Variables
- PHP - Constants
- PHP - Magic Constants
- PHP - Data Types
- PHP - Type Casting
- PHP - Type Juggling
- PHP - Strings
- PHP - Boolean
- PHP - Integers
- PHP - Files & I/O
- PHP - Maths Functions
- PHP - Heredoc & Nowdoc
- PHP - Compound Types
- PHP - File Include
- PHP - Date & Time
- PHP - Scalar Type Declarations
- PHP - Return Type Declarations
- PHP Operators
- PHP - Operators
- PHP - Arithmatic Operators
- PHP - Comparison Operators
- PHP - Logical Operators
- PHP - Assignment Operators
- PHP - String Operators
- PHP - Array Operators
- PHP - Conditional Operators
- PHP - Spread Operator
- PHP - Null Coalescing Operator
- PHP - Spaceship Operator
- PHP Control Statements
- PHP - Decision Making
- PHP - If…Else Statement
- PHP - Switch Statement
- PHP - Loop Types
- PHP - For Loop
- PHP - Foreach Loop
- PHP - While Loop
- PHP - Do…While Loop
- PHP - Break Statement
- PHP - Continue Statement
- PHP Arrays
- PHP - Arrays
- PHP - Indexed Array
- PHP - Associative Array
- PHP - Multidimensional Array
- PHP - Array Functions
- PHP - Constant Arrays
- PHP Functions
- PHP - Functions
- PHP - Function Parameters
- PHP - Call by value
- PHP - Call by Reference
- PHP - Default Arguments
- PHP - Named Arguments
- PHP - Variable Arguments
- PHP - Returning Values
- PHP - Passing Functions
- PHP - Recursive Functions
- PHP - Type Hints
- PHP - Variable Scope
- PHP - Strict Typing
- PHP - Anonymous Functions
- PHP - Arrow Functions
- PHP - Variable Functions
- PHP - Local Variables
- PHP - Global Variables
- PHP Superglobals
- PHP - Superglobals
- PHP - $GLOBALS
- PHP - $_SERVER
- PHP - $_REQUEST
- PHP - $_POST
- PHP - $_GET
- PHP - $_FILES
- PHP - $_ENV
- PHP - $_COOKIE
- PHP - $_SESSION
- PHP File Handling
- PHP - File Handling
- PHP - Open File
- PHP - Read File
- PHP - Write File
- PHP - File Existence
- PHP - Download File
- PHP - Copy File
- PHP - Append File
- PHP - Delete File
- PHP - Handle CSV File
- PHP - File Permissions
- PHP - Create Directory
- PHP - Listing Files
- Object Oriented PHP
- PHP - Object Oriented Programming
- PHP - Classes and Objects
- PHP - Constructor and Destructor
- PHP - Access Modifiers
- PHP - Inheritance
- PHP - Class Constants
- PHP - Abstract Classes
- PHP - Interfaces
- PHP - Traits
- PHP - Static Methods
- PHP - Static Properties
- PHP - Namespaces
- PHP - Object Iteration
- PHP - Encapsulation
- PHP - Final Keyword
- PHP - Overloading
- PHP - Cloning Objects
- PHP - Anonymous Classes
- PHP Web Development
- PHP - Web Concepts
- PHP - Form Handling
- PHP - Form Validation
- PHP - Form Email/URL
- PHP - Complete Form
- PHP - File Inclusion
- PHP - GET & POST
- PHP - File Uploading
- PHP - Cookies
- PHP - Sessions
- PHP - Session Options
- PHP - Sending Emails
- PHP - Sanitize Input
- PHP - Post-Redirect-Get (PRG)
- PHP - Flash Messages
- PHP AJAX
- PHP - AJAX Introduction
- PHP - AJAX Search
- PHP - AJAX XML Parser
- PHP - AJAX Auto Complete Search
- PHP - AJAX RSS Feed Example
- PHP XML
- PHP - XML Introduction
- PHP - Simple XML Parser
- PHP - SAX Parser Example
- PHP - DOM Parser Example
- PHP Login Example
- PHP - Login Example
- PHP - Facebook Login
- PHP - Paypal Integration
- PHP - MySQL Login
- PHP Advanced
- PHP - MySQL
- PHP.INI File Configuration
- PHP - Array Destructuring
- PHP - Coding Standard
- PHP - Regular Expression
- PHP - Error Handling
- PHP - Try…Catch
- PHP - Bugs Debugging
- PHP - For C Developers
- PHP - For PERL Developers
- PHP - Frameworks
- PHP - Core PHP vs Frame Works
- PHP - Design Patterns
- PHP - Filters
- PHP - JSON
- PHP - Exceptions
- PHP - Special Types
- PHP - Hashing
- PHP - Encryption
- PHP - is_null() Function
- PHP - System Calls
- PHP - HTTP Authentication
- PHP - Swapping Variables
- PHP - Closure::call()
- PHP - Filtered unserialize()
- PHP - IntlChar
- PHP - CSPRNG
- PHP - Expectations
- PHP - Use Statement
- PHP - Integer Division
- PHP - Deprecated Features
- PHP - Removed Extensions & SAPIs
- PHP - PEAR
- PHP - CSRF
- PHP - FastCGI Process
- PHP - PDO Extension
- PHP - Built-In Functions
- PHP Useful Resources
- PHP - Questions & Answers
- PHP - Quick Guide
- PHP - Useful Resources
- PHP - Discussion
PHP - Spaceship Operator
The Spaceship operator is one of the many new features introduced in PHP with its 7.0 version. It is a three-way comparison operator.
The conventional comparison operators (<, >, !=, ==, etc.) return true or false (equivalent to 1 or 0). On the other hand, the spaceship operator has three possible return values: -1,0,or 1. This operator can be used with integers, floats, strings, arrays, objects, etc.
Syntax
The symbol used for spaceship operator is "<=>".
$retval = operand1 <=> operand2
Here, $retval is -1 if operand1 is less than operand2, 0 if both the operands are equal, and 1 if operand1 is greater than operand2.
The spaceship operator is implemented as a combined comparison operator. Conventional comparison operators could be considered mere shorthands for <=> as the following table shows −
Operator |
<=> equivalent |
---|---|
$a < $b |
($a <=> $b) === -1 |
$a <= $b |
($a <=> $b) === -1 || ($a <=> $b) === 0 |
$a == $b |
($a <=> $b) === 0 |
$a != $b |
($a <=> $b) !== 0 |
$a >= $b |
($a <=> $b) === 1 || ($a <=> $b) === 0 |
$a > $b |
($a <=> $b) === 1 |
Example 1
The following example shows how you can use the spaceship operator in PHP −
<?php $x = 5; $y = 10; $z = $x <=> $y/2; echo "$x <=> $y/2 = $z"; ?>
It will produce the following output −
5 <=> 10/2 = 0
Example 2
Change $x=4 and check the result −
<?php $x = 4; $y = 10; $z = $x <=> $y/2; echo "$x <=> $y/2 = $z"; ?>
It will produce the following output −
4 <=> 10/2 = -1
Example 3
Change $y=7 and check the result again −
<?php $x = 7; $y = 10; $z = $x <=> $y/2; echo "$x <=> $y/2 = $z"; ?>
It will produce the following output −
7 <=> 10/2 = 1
Example 4
When used with string operands, the spaceship operand works just like the strcmp() function.
<?php $x = "bat"; $y = "ball"; $z = $x <=> $y; echo "$x <=> $y = $z"; ?>
It will produce the following output −
bat <=> ball = 1
Example 5
Change $y = "baz" and check the result −
<?php $x = "bat"; $y = "baz"; $z = $x <=> $y; echo "$x <=> $y = $z"; ?>
It will produce the following output −
bat <=> baz = -1
Spaceship Operator with Boolean Operands
The spaceship operator also works with Boolean operands −
true <=> false returns 1 false <=> true returns -1 true <=> true as well as false <=> false returns 0