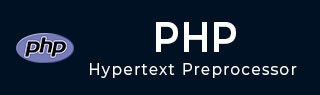
- PHP Tutorial
- PHP - Home
- PHP - Introduction
- PHP - Installation
- PHP - History
- PHP - Features
- PHP - Syntax
- PHP - Hello World
- PHP - Comments
- PHP - Variables
- PHP - Echo/Print
- PHP - var_dump
- PHP - $ and $$ Variables
- PHP - Constants
- PHP - Magic Constants
- PHP - Data Types
- PHP - Type Casting
- PHP - Type Juggling
- PHP - Strings
- PHP - Boolean
- PHP - Integers
- PHP - Files & I/O
- PHP - Maths Functions
- PHP - Heredoc & Nowdoc
- PHP - Compound Types
- PHP - File Include
- PHP - Date & Time
- PHP - Scalar Type Declarations
- PHP - Return Type Declarations
- PHP Operators
- PHP - Operators
- PHP - Arithmatic Operators
- PHP - Comparison Operators
- PHP - Logical Operators
- PHP - Assignment Operators
- PHP - String Operators
- PHP - Array Operators
- PHP - Conditional Operators
- PHP - Spread Operator
- PHP - Null Coalescing Operator
- PHP - Spaceship Operator
- PHP Control Statements
- PHP - Decision Making
- PHP - If…Else Statement
- PHP - Switch Statement
- PHP - Loop Types
- PHP - For Loop
- PHP - Foreach Loop
- PHP - While Loop
- PHP - Do…While Loop
- PHP - Break Statement
- PHP - Continue Statement
- PHP Arrays
- PHP - Arrays
- PHP - Indexed Array
- PHP - Associative Array
- PHP - Multidimensional Array
- PHP - Array Functions
- PHP - Constant Arrays
- PHP Functions
- PHP - Functions
- PHP - Function Parameters
- PHP - Call by value
- PHP - Call by Reference
- PHP - Default Arguments
- PHP - Named Arguments
- PHP - Variable Arguments
- PHP - Returning Values
- PHP - Passing Functions
- PHP - Recursive Functions
- PHP - Type Hints
- PHP - Variable Scope
- PHP - Strict Typing
- PHP - Anonymous Functions
- PHP - Arrow Functions
- PHP - Variable Functions
- PHP - Local Variables
- PHP - Global Variables
- PHP Superglobals
- PHP - Superglobals
- PHP - $GLOBALS
- PHP - $_SERVER
- PHP - $_REQUEST
- PHP - $_POST
- PHP - $_GET
- PHP - $_FILES
- PHP - $_ENV
- PHP - $_COOKIE
- PHP - $_SESSION
- PHP File Handling
- PHP - File Handling
- PHP - Open File
- PHP - Read File
- PHP - Write File
- PHP - File Existence
- PHP - Download File
- PHP - Copy File
- PHP - Append File
- PHP - Delete File
- PHP - Handle CSV File
- PHP - File Permissions
- PHP - Create Directory
- PHP - Listing Files
- Object Oriented PHP
- PHP - Object Oriented Programming
- PHP - Classes and Objects
- PHP - Constructor and Destructor
- PHP - Access Modifiers
- PHP - Inheritance
- PHP - Class Constants
- PHP - Abstract Classes
- PHP - Interfaces
- PHP - Traits
- PHP - Static Methods
- PHP - Static Properties
- PHP - Namespaces
- PHP - Object Iteration
- PHP - Encapsulation
- PHP - Final Keyword
- PHP - Overloading
- PHP - Cloning Objects
- PHP - Anonymous Classes
- PHP Web Development
- PHP - Web Concepts
- PHP - Form Handling
- PHP - Form Validation
- PHP - Form Email/URL
- PHP - Complete Form
- PHP - File Inclusion
- PHP - GET & POST
- PHP - File Uploading
- PHP - Cookies
- PHP - Sessions
- PHP - Session Options
- PHP - Sending Emails
- PHP - Sanitize Input
- PHP - Post-Redirect-Get (PRG)
- PHP - Flash Messages
- PHP AJAX
- PHP - AJAX Introduction
- PHP - AJAX Search
- PHP - AJAX XML Parser
- PHP - AJAX Auto Complete Search
- PHP - AJAX RSS Feed Example
- PHP XML
- PHP - XML Introduction
- PHP - Simple XML Parser
- PHP - SAX Parser Example
- PHP - DOM Parser Example
- PHP Login Example
- PHP - Login Example
- PHP - Facebook Login
- PHP - Paypal Integration
- PHP - MySQL Login
- PHP Advanced
- PHP - MySQL
- PHP.INI File Configuration
- PHP - Array Destructuring
- PHP - Coding Standard
- PHP - Regular Expression
- PHP - Error Handling
- PHP - Try…Catch
- PHP - Bugs Debugging
- PHP - For C Developers
- PHP - For PERL Developers
- PHP - Frameworks
- PHP - Core PHP vs Frame Works
- PHP - Design Patterns
- PHP - Filters
- PHP - JSON
- PHP - Exceptions
- PHP - Special Types
- PHP - Hashing
- PHP - Encryption
- PHP - is_null() Function
- PHP - System Calls
- PHP - HTTP Authentication
- PHP - Swapping Variables
- PHP - Closure::call()
- PHP - Filtered unserialize()
- PHP - IntlChar
- PHP - CSPRNG
- PHP - Expectations
- PHP - Use Statement
- PHP - Integer Division
- PHP - Deprecated Features
- PHP - Removed Extensions & SAPIs
- PHP - PEAR
- PHP - CSRF
- PHP - FastCGI Process
- PHP - PDO Extension
- PHP - Built-In Functions
- PHP Useful Resources
- PHP - Questions & Answers
- PHP - Quick Guide
- PHP - Useful Resources
- PHP - Discussion
PHP - For Loop
A program by default follows a sequential execution of statements. If the program flow is directed towards any of earlier statements in the program, it constitutes a loop. The for statement in PHP is a convenient tool to constitute a loop in a PHP script. In this chapter, we will discuss PHP’s for statement.
Flowchart of "for" Loop
The following flowchart explains how a for loop works −
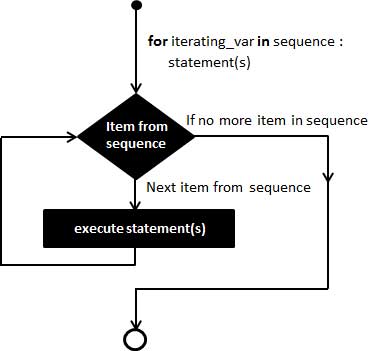
The for statement is used when you know how many times you want to execute a statement or a block of statements.
Syntax of "for" Loop
The syntax of for statement in PHP is similar to the for statement in C language.
for (expr1; expr2; expr3){ code to be executed; }
The for keyword is followed by a parenthesis containing three expressions separated by a semicolon. Each of them may be empty or may contain multiple expressions separated by commas. The parenthesis is followed by one or more statements put inside curly brackets. It forms the body of the loop.
The first expression in the parenthesis is executed only at the start of the loop. It generally acts as the initializer used to set the start value for the counter of the number of loop iterations.
In the beginning of each iteration, expr2 is evaluated. If it evaluates to true, the loop continues and the statements in the body block are executed. If it evaluates to false, the execution of the loop ends. Generally, the expr2 specifies the final value of the counter.
The expr3 is executed at the end of each iteration. In most cases, this expression increments the counter variable.
Example
The most general example of a for loop is as follows −
<?php for ($i=1; $i<=10; $i++){ echo "Iteration No: $i \n"; } ?>
Here is its output −
Iteration No: 1 Iteration No: 2 Iteration No: 3 Iteration No: 4 Iteration No: 5 Iteration No: 6 Iteration No: 7 Iteration No: 8 Iteration No: 9 Iteration No: 10
An infinite "for" loop
Note that all the three expressions in the parenthesis are optional. A for statement with only two semicolons constitutes an infinite loop.
for (; ;) { Loop body }
To stop the infinite iteration, you need to use a break statement inside the body of the loop.
A decrementing "for" loop
You can also form a decrementing for loop. To have a for loop that goes from 10 to 1, initialize the looping variable with 10, the expression in the middle that is evaluated at the beginning of each iteration checks whether it is greater than 1. The last expression to be executed at the end of each iteration should decrement it by 1.
<?php for ($i=10; $i>=1; $i--){ echo "Iteration No: $i \n"; } ?>
It will produce the following output −
Iteration No: 10 Iteration No: 9 Iteration No: 8 Iteration No: 7 Iteration No: 6 Iteration No: 5 Iteration No: 4 Iteration No: 3 Iteration No: 2 Iteration No: 1
Using the "for…endfor" construct
You can also use the ":" (colon) symbol to start the looping block and put endfor statement at the end of the block.
<?php for ($i=1; $i<=10; $i++): echo "Iteration No: $i \n"; endfor; ?>
Iterating an indexed array using "for" loop
Each element in the array is identified by an incrementing index starting with "0". If an array of 5 elements is present, its lower bound is 0 and is upper bound is 4 (size of array -1).
To obtain the number of elements in an array, there is a count() function. Hence, we can iterate over an indexed array by using the following for statement −
<?php $numbers = array(10, 20, 30, 40, 50); for ($i=0; $i<count($numbers); $i++){ echo "numbers[$i] = $numbers[$i] \n"; } ?>
It will produce the following output −
numbers[0] = 10 numbers[1] = 20 numbers[2] = 30 numbers[3] = 40 numbers[4] = 50
Iterating an Associative Array Using "for" Loop
An associative array in PHP is a collection of key-value pairs. An arrow symbol (=>) is used to show the association between the key and its value. We use the array_keys() function to obtain array of keys.
The following for loop prints the capital of each state from an associative array $capitals defined in the code −
<?php $capitals = array( "Maharashtra"=>"Mumbai", "Telangana"=>"Hyderabad", "UP"=>"Lucknow", "Tamilnadu"=>"Chennai" ); $keys=array_keys($capitals); for ($i=0; $i<count($keys); $i++){ $cap = $keys[$i]; echo "Capital of $cap is $capitals[$cap] \n"; } ?>
Here is its output −
Capital of Maharashtra is Mumbai Capital of Telangana is Hyderabad Capital of UP is Lucknow Capital of Tamilnadu is Chennai
Using Nested "for" Loops in PHP
If another for loop is used inside the body of an existing loop, the two loops are said to have been nested.
For each value of counter variable of the outer loop, all the iterations of inner loop are completed.
<?php for ($i=1; $i<=3; $i++){ for ($j=1; $j<=3; $j++){ echo "i= $i j= $j \n"; } } ?>
It will produce the following output −
i= 1 j= 1 i= 1 j= 2 i= 1 j= 3 i= 2 j= 1 i= 2 j= 2 i= 2 j= 3 i= 3 j= 1 i= 3 j= 2 i= 3 j= 3
Note that a string is a form of an array. The strlen() function gives the number of characters in a string.
Example
The following PHP script uses two nested loops to print incrementing number of characters from a string in each line.
<?php $str = "TutorialsPoint"; for ($i=0; $i<strlen($str); $i++){ for ($j=0; $j<=$i; $j++){ echo "$str[$j]"; } echo "\n"; } ?>
It will produce the following output −
T Tu Tut Tuto Tutor Tutori Tutoria Tutorial Tutorials TutorialsP TutorialsPo TutorialsPoi TutorialsPoin TutorialsPoint