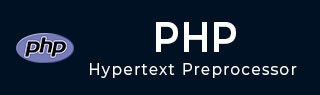
- PHP Tutorial
- PHP - Home
- PHP - Introduction
- PHP - Installation
- PHP - History
- PHP - Features
- PHP - Syntax
- PHP - Hello World
- PHP - Comments
- PHP - Variables
- PHP - Echo/Print
- PHP - var_dump
- PHP - $ and $$ Variables
- PHP - Constants
- PHP - Magic Constants
- PHP - Data Types
- PHP - Type Casting
- PHP - Type Juggling
- PHP - Strings
- PHP - Boolean
- PHP - Integers
- PHP - Files & I/O
- PHP - Maths Functions
- PHP - Heredoc & Nowdoc
- PHP - Compound Types
- PHP - File Include
- PHP - Date & Time
- PHP - Scalar Type Declarations
- PHP - Return Type Declarations
- PHP Operators
- PHP - Operators
- PHP - Arithmatic Operators
- PHP - Comparison Operators
- PHP - Logical Operators
- PHP - Assignment Operators
- PHP - String Operators
- PHP - Array Operators
- PHP - Conditional Operators
- PHP - Spread Operator
- PHP - Null Coalescing Operator
- PHP - Spaceship Operator
- PHP Control Statements
- PHP - Decision Making
- PHP - If…Else Statement
- PHP - Switch Statement
- PHP - Loop Types
- PHP - For Loop
- PHP - Foreach Loop
- PHP - While Loop
- PHP - Do…While Loop
- PHP - Break Statement
- PHP - Continue Statement
- PHP Arrays
- PHP - Arrays
- PHP - Indexed Array
- PHP - Associative Array
- PHP - Multidimensional Array
- PHP - Array Functions
- PHP - Constant Arrays
- PHP Functions
- PHP - Functions
- PHP - Function Parameters
- PHP - Call by value
- PHP - Call by Reference
- PHP - Default Arguments
- PHP - Named Arguments
- PHP - Variable Arguments
- PHP - Returning Values
- PHP - Passing Functions
- PHP - Recursive Functions
- PHP - Type Hints
- PHP - Variable Scope
- PHP - Strict Typing
- PHP - Anonymous Functions
- PHP - Arrow Functions
- PHP - Variable Functions
- PHP - Local Variables
- PHP - Global Variables
- PHP Superglobals
- PHP - Superglobals
- PHP - $GLOBALS
- PHP - $_SERVER
- PHP - $_REQUEST
- PHP - $_POST
- PHP - $_GET
- PHP - $_FILES
- PHP - $_ENV
- PHP - $_COOKIE
- PHP - $_SESSION
- PHP File Handling
- PHP - File Handling
- PHP - Open File
- PHP - Read File
- PHP - Write File
- PHP - File Existence
- PHP - Download File
- PHP - Copy File
- PHP - Append File
- PHP - Delete File
- PHP - Handle CSV File
- PHP - File Permissions
- PHP - Create Directory
- PHP - Listing Files
- Object Oriented PHP
- PHP - Object Oriented Programming
- PHP - Classes and Objects
- PHP - Constructor and Destructor
- PHP - Access Modifiers
- PHP - Inheritance
- PHP - Class Constants
- PHP - Abstract Classes
- PHP - Interfaces
- PHP - Traits
- PHP - Static Methods
- PHP - Static Properties
- PHP - Namespaces
- PHP - Object Iteration
- PHP - Encapsulation
- PHP - Final Keyword
- PHP - Overloading
- PHP - Cloning Objects
- PHP - Anonymous Classes
- PHP Web Development
- PHP - Web Concepts
- PHP - Form Handling
- PHP - Form Validation
- PHP - Form Email/URL
- PHP - Complete Form
- PHP - File Inclusion
- PHP - GET & POST
- PHP - File Uploading
- PHP - Cookies
- PHP - Sessions
- PHP - Session Options
- PHP - Sending Emails
- PHP - Sanitize Input
- PHP - Post-Redirect-Get (PRG)
- PHP - Flash Messages
- PHP AJAX
- PHP - AJAX Introduction
- PHP - AJAX Search
- PHP - AJAX XML Parser
- PHP - AJAX Auto Complete Search
- PHP - AJAX RSS Feed Example
- PHP XML
- PHP - XML Introduction
- PHP - Simple XML Parser
- PHP - SAX Parser Example
- PHP - DOM Parser Example
- PHP Login Example
- PHP - Login Example
- PHP - Facebook Login
- PHP - Paypal Integration
- PHP - MySQL Login
- PHP Advanced
- PHP - MySQL
- PHP.INI File Configuration
- PHP - Array Destructuring
- PHP - Coding Standard
- PHP - Regular Expression
- PHP - Error Handling
- PHP - Try…Catch
- PHP - Bugs Debugging
- PHP - For C Developers
- PHP - For PERL Developers
- PHP - Frameworks
- PHP - Core PHP vs Frame Works
- PHP - Design Patterns
- PHP - Filters
- PHP - JSON
- PHP - Exceptions
- PHP - Special Types
- PHP - Hashing
- PHP - Encryption
- PHP - is_null() Function
- PHP - System Calls
- PHP - HTTP Authentication
- PHP - Swapping Variables
- PHP - Closure::call()
- PHP - Filtered unserialize()
- PHP - IntlChar
- PHP - CSPRNG
- PHP - Expectations
- PHP - Use Statement
- PHP - Integer Division
- PHP - Deprecated Features
- PHP - Removed Extensions & SAPIs
- PHP - PEAR
- PHP - CSRF
- PHP - FastCGI Process
- PHP - PDO Extension
- PHP - Built-In Functions
- PHP Useful Resources
- PHP - Questions & Answers
- PHP - Quick Guide
- PHP - Useful Resources
- PHP - Discussion
PHP - Constants
A constant in PHP is a name or an identifier for a simple value. A constant value cannot change during the execution of the PHP script.
By default, a PHP constant is case-sensitive.
By convention, constant identifiers are always uppercase.
A constant name starts with a letter or underscore, followed by any number of letters, numbers, or underscore.
There is no need to write a dollar sign ($) before a constant, however one has to use a dollar sign before a variable.
Examples of Valid and Invalid Constant Names in PHP
Here are some examples of valid and invalid constant names in PHP −
// Valid constant names define("ONE", "first thing"); define("TWO2", "second thing"); define("THREE_3", "third thing"); define("__THREE__", "third value"); // Invalid constant names define("2TWO", "second thing");
Difference between Constants and Variables in PHP
Constants cannot be defined by simple assignment; they can only be defined using the define() function.
Constants may be defined and accessed anywhere without regard to variable scoping rules.
Once the Constants have been set, they may not be redefined or undefined.
Defining a Named Constant
The define() function in PHP library is used to define a named constant at runtime.
define(string $const_name, mixed $value, bool $case = false): bool
Parameters
const_name − The name of the constant.
value − The value of the constant. It can be a scalar value (int, float, string, bool, or null) or array values are also accepted.
case − If set to true, the constant will be defined case-insensitive. The default behavior is case-sensitive, i.e., CONSTANT and Constant represent different values.
The define() function returns "true" on success and "false" on failure.
Example 1
The following example demonstrates how the define() function works −
<?php define("CONSTANT", "Hello world."); echo CONSTANT; // echo Constant; ?>
The first echo statement outputs the value of CONSTANT. You will get the following output −
Hello world.
But, when you uncomment the second echo statement, it will display the following error −
Fatal error: Uncaught Error: Undefined constant "Constant" in hello.php: on line 5
If you set the case parameter to False, PHP doesn’t differentiate upper and lowercase constants.
Example 2
You can also use an array as the value of a constant. Take a look at the following example −
<?php define( $name="LANGS", $value=array('PHP', 'Java', 'Python') ); var_dump(LANGS); ?>
It will produce the following output −
array(3) { [0]=> string(3) "PHP" [1]=> string(4) "Java" [2]=> string(6) "Python" }
Using the constant() Function
The echo statement outputs the value of the defined constant. You can also use the constant() function. It returns the value of the constant indicated by name.
constant(string $name): mixed
The constant() function is useful if you need to retrieve the value of a constant, but do not know its name. I.e. it is stored in a variable or returned by a function.
<?php define("MINSIZE", 50); echo MINSIZE; echo PHP_EOL; echo constant("MINSIZE"); // same thing as the previous line ?>
It will produce the following output −
50 50
Using the defined() Function
The PHP library provides a defined() function that checks whether a given named constant exists. Take a look at the following example −
<?php define('MAX', 100); if (defined('MAX')) { echo MAX; } ?>
It will produce the following output −
100
PHP also has a function called "get_defined_constants()" that returns an associative array of all the defined constants and their values.