
- Matplotlib Basics
- Matplotlib - Home
- Matplotlib - Introduction
- Matplotlib - Vs Seaborn
- Matplotlib - Environment Setup
- Matplotlib - Anaconda distribution
- Matplotlib - Jupyter Notebook
- Matplotlib - Pyplot API
- Matplotlib - Simple Plot
- Matplotlib - Saving Figures
- Matplotlib - Markers
- Matplotlib - Figures
- Matplotlib - Styles
- Matplotlib - Legends
- Matplotlib - Colors
- Matplotlib - Colormaps
- Matplotlib - Colormap Normalization
- Matplotlib - Choosing Colormaps
- Matplotlib - Colorbars
- Matplotlib - Text
- Matplotlib - Text properties
- Matplotlib - Subplot Titles
- Matplotlib - Images
- Matplotlib - Image Masking
- Matplotlib - Annotations
- Matplotlib - Arrows
- Matplotlib - Fonts
- Matplotlib - What are Fonts?
- Setting Font Properties Globally
- Matplotlib - Font Indexing
- Matplotlib - Font Properties
- Matplotlib - Scales
- Matplotlib - Linear and Logarthmic Scales
- Matplotlib - Symmetrical Logarithmic and Logit Scales
- Matplotlib - LaTeX
- Matplotlib - What is LaTeX?
- Matplotlib - LaTeX for Mathematical Expressions
- Matplotlib - LaTeX Text Formatting in Annotations
- Matplotlib - PostScript
- Enabling LaTex Rendering in Annotations
- Matplotlib - Mathematical Expressions
- Matplotlib - Animations
- Matplotlib - Artists
- Matplotlib - Styling with Cycler
- Matplotlib - Paths
- Matplotlib - Path Effects
- Matplotlib - Transforms
- Matplotlib - Ticks and Tick Labels
- Matplotlib - Radian Ticks
- Matplotlib - Dateticks
- Matplotlib - Tick Formatters
- Matplotlib - Tick Locators
- Matplotlib - Basic Units
- Matplotlib - Autoscaling
- Matplotlib - Reverse Axes
- Matplotlib - Logarithmic Axes
- Matplotlib - Symlog
- Matplotlib - Unit Handling
- Matplotlib - Ellipse with Units
- Matplotlib - Spines
- Matplotlib - Axis Ranges
- Matplotlib - Axis Scales
- Matplotlib - Axis Ticks
- Matplotlib - Formatting Axes
- Matplotlib - Axes Class
- Matplotlib - Twin Axes
- Matplotlib - Figure Class
- Matplotlib - Multiplots
- Matplotlib - Grids
- Matplotlib - Object-oriented Interface
- Matplotlib - PyLab module
- Matplotlib - Subplots() Function
- Matplotlib - Subplot2grid() Function
- Matplotlib - Anchored Artists
- Matplotlib - Manual Contour
- Matplotlib - Coords Report
- Matplotlib - AGG filter
- Matplotlib - Ribbon Box
- Matplotlib - Fill Spiral
- Matplotlib - Findobj Demo
- Matplotlib - Hyperlinks
- Matplotlib - Image Thumbnail
- Matplotlib - Plotting with Keywords
- Matplotlib - Create Logo
- Matplotlib - Multipage PDF
- Matplotlib - Multiprocessing
- Matplotlib - Print Stdout
- Matplotlib - Compound Path
- Matplotlib - Sankey Class
- Matplotlib - MRI with EEG
- Matplotlib - Stylesheets
- Matplotlib - Background Colors
- Matplotlib - Basemap
- Matplotlib Event Handling
- Matplotlib - Event Handling
- Matplotlib - Close Event
- Matplotlib - Mouse Move
- Matplotlib - Click Events
- Matplotlib - Scroll Event
- Matplotlib - Keypress Event
- Matplotlib - Pick Event
- Matplotlib - Looking Glass
- Matplotlib - Path Editor
- Matplotlib - Poly Editor
- Matplotlib - Timers
- Matplotlib - Viewlims
- Matplotlib - Zoom Window
- Matplotlib Plotting
- Matplotlib - Bar Graphs
- Matplotlib - Histogram
- Matplotlib - Pie Chart
- Matplotlib - Scatter Plot
- Matplotlib - Box Plot
- Matplotlib - Violin Plot
- Matplotlib - Contour Plot
- Matplotlib - 3D Plotting
- Matplotlib - 3D Contours
- Matplotlib - 3D Wireframe Plot
- Matplotlib - 3D Surface Plot
- Matplotlib - Quiver Plot
- Matplotlib Useful Resources
- Matplotlib - Quick Guide
- Matplotlib - Useful Resources
- Matplotlib - Discussion
Matplotlib - Findobj Demo
In Matplotlib, the findobj is a method used for locating and manipulating graphical objects within a figure. Its primary purpose is to provide a flexible and convenient way to search for specific artist instances and modify their properties within a plot.
This method is available in both the interfaces such as pyplot.findobj() and axes.Axes.findobj() (pyplot and Object-oriented interfaces). All Artist objects in Matplotlib inherit this method, allowing for recursive searches within their hierarchy. The findobj method takes a matching criterion and optionally includes the object itself in the search.
In this tutorial, we will explore the fundamental concepts behind the findobj method in Matplotlib.
Finding object
The findobj() method can be used to locate specific types of objects within a plot. For example, users can search for Line2D instances to identify plotted lines within the current Axes.
Example
The following example creates two sets of line plots using the plot() method then you can use the findobj() method to find out the Line2D instance corresponding to the sinusoidal line.
import matplotlib.pyplot as plt from matplotlib.lines import Line2D import numpy as np # Generate data t = np.arange(0, 4, 0.1) F1 = np.sin(2 * t) F2 = np.exp(-t*4) # Plot two lines fig, ax = plt.subplots(figsize=(7, 4)) line1, = ax.plot(t, F1, 'green', label='Sinusoidal') line2, = ax.plot(t, F2, 'b--', label='Exponential Decay') # Find the Line object corresponding to the sinusoidal line line_handle = plt.gca().findobj(Line2D)[0] # Display the results print("Found Line2D instance:", line_handle) # Display the modified plot plt.legend() plt.show()
Output
On executing the above code we will get the following output −
Found Line2D instance: Line2D(Sinusoidal)
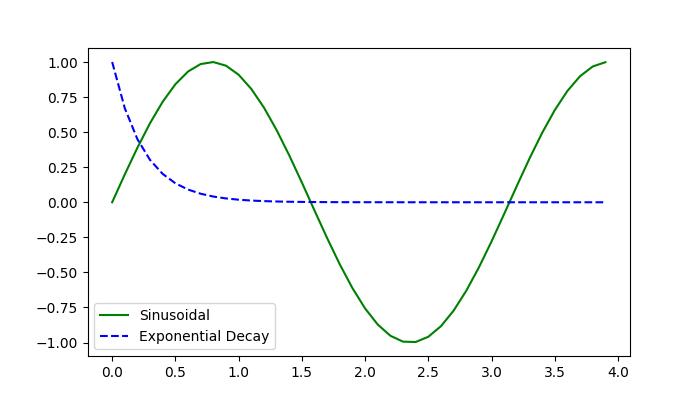
Modifying Objects
Once an object is located/identified using the findobj() method, users can modify its properties to achieve the desired visualization. This includes changing attributes such as color, linestyle, text content, and more.
Example 1
Here is an example that creates a plot with three Text objects containing different text content. The Axes.findobj() method is used to locate a Text object with specific text content ('tutorialspoint'). Once found, the text content is modified to 'Tutorialspoint :)' and its color is changed to green.
import matplotlib.pyplot as plt from matplotlib.text import Text # Create a plot with a text object fig, ax = plt.subplots() ax.text(.4, .5, s='tutorialspoint') ax.text(.1, .1, s='Python') ax.text(.8, .8, s='Matplotlib') # Find the Text object with the text 'tutorialspoint' text_handle = ax.findobj( lambda artist: isinstance(artist, Text) and artist.get_text() == 'tutorialspoint')[0] # Modify the text text_handle.set_text('Tutorialspoint :)') text_handle.set_color('green') plt.show()
Output
On executing the above code we will get the following output −
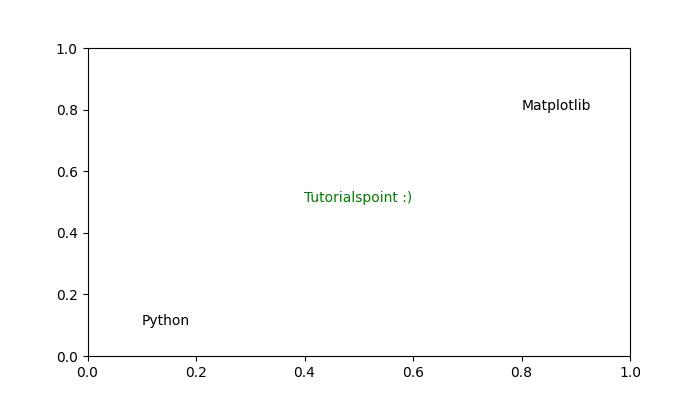
Users can also define custom matching functions to filter objects based on specific criteria. This allows for more complex searches and enables advanced manipulation of graphical objects.
Example 2
Here is another example that demonstrates how to use of a custom matching function to modify objects using the Figure.findobj() method.
import matplotlib.pyplot as plt import matplotlib.text as text import numpy as np # Generate data t = np.arange(0, 4, 0.1) F1 = np.sin(2 * t) F2 = np.exp(-t * 4) # Plot two lines fig, ax = plt.subplots(figsize=(7, 4)) line1, = ax.plot(t, F1, 'red', label='Sinusoidal') line2, = ax.plot(t, F2, 'k--', label='Exponential Decay') plt.grid(False) plt.xlabel('x label') plt.ylabel('y label') plt.title('Modifying the Objects') # Define a custom function for matching def myfunc(x): return hasattr(x, 'set_color') and not hasattr(x, 'set_facecolor') # Modify objects based on the custom function for o in fig.findobj(myfunc): o.set_color('green') # Display the modified plot plt.show()
Output
On executing the above code we will get the following output −
