
- Matplotlib Basics
- Matplotlib - Home
- Matplotlib - Introduction
- Matplotlib - Vs Seaborn
- Matplotlib - Environment Setup
- Matplotlib - Anaconda distribution
- Matplotlib - Jupyter Notebook
- Matplotlib - Pyplot API
- Matplotlib - Simple Plot
- Matplotlib - Saving Figures
- Matplotlib - Markers
- Matplotlib - Figures
- Matplotlib - Styles
- Matplotlib - Legends
- Matplotlib - Colors
- Matplotlib - Colormaps
- Matplotlib - Colormap Normalization
- Matplotlib - Choosing Colormaps
- Matplotlib - Colorbars
- Matplotlib - Text
- Matplotlib - Text properties
- Matplotlib - Subplot Titles
- Matplotlib - Images
- Matplotlib - Image Masking
- Matplotlib - Annotations
- Matplotlib - Arrows
- Matplotlib - Fonts
- Matplotlib - What are Fonts?
- Setting Font Properties Globally
- Matplotlib - Font Indexing
- Matplotlib - Font Properties
- Matplotlib - Scales
- Matplotlib - Linear and Logarthmic Scales
- Matplotlib - Symmetrical Logarithmic and Logit Scales
- Matplotlib - LaTeX
- Matplotlib - What is LaTeX?
- Matplotlib - LaTeX for Mathematical Expressions
- Matplotlib - LaTeX Text Formatting in Annotations
- Matplotlib - PostScript
- Enabling LaTex Rendering in Annotations
- Matplotlib - Mathematical Expressions
- Matplotlib - Animations
- Matplotlib - Artists
- Matplotlib - Styling with Cycler
- Matplotlib - Paths
- Matplotlib - Path Effects
- Matplotlib - Transforms
- Matplotlib - Ticks and Tick Labels
- Matplotlib - Radian Ticks
- Matplotlib - Dateticks
- Matplotlib - Tick Formatters
- Matplotlib - Tick Locators
- Matplotlib - Basic Units
- Matplotlib - Autoscaling
- Matplotlib - Reverse Axes
- Matplotlib - Logarithmic Axes
- Matplotlib - Symlog
- Matplotlib - Unit Handling
- Matplotlib - Ellipse with Units
- Matplotlib - Spines
- Matplotlib - Axis Ranges
- Matplotlib - Axis Scales
- Matplotlib - Axis Ticks
- Matplotlib - Formatting Axes
- Matplotlib - Axes Class
- Matplotlib - Twin Axes
- Matplotlib - Figure Class
- Matplotlib - Multiplots
- Matplotlib - Grids
- Matplotlib - Object-oriented Interface
- Matplotlib - PyLab module
- Matplotlib - Subplots() Function
- Matplotlib - Subplot2grid() Function
- Matplotlib - Anchored Artists
- Matplotlib - Manual Contour
- Matplotlib - Coords Report
- Matplotlib - AGG filter
- Matplotlib - Ribbon Box
- Matplotlib - Fill Spiral
- Matplotlib - Findobj Demo
- Matplotlib - Hyperlinks
- Matplotlib - Image Thumbnail
- Matplotlib - Plotting with Keywords
- Matplotlib - Create Logo
- Matplotlib - Multipage PDF
- Matplotlib - Multiprocessing
- Matplotlib - Print Stdout
- Matplotlib - Compound Path
- Matplotlib - Sankey Class
- Matplotlib - MRI with EEG
- Matplotlib - Stylesheets
- Matplotlib - Background Colors
- Matplotlib - Basemap
- Matplotlib Event Handling
- Matplotlib - Event Handling
- Matplotlib - Close Event
- Matplotlib - Mouse Move
- Matplotlib - Click Events
- Matplotlib - Scroll Event
- Matplotlib - Keypress Event
- Matplotlib - Pick Event
- Matplotlib - Looking Glass
- Matplotlib - Path Editor
- Matplotlib - Poly Editor
- Matplotlib - Timers
- Matplotlib - Viewlims
- Matplotlib - Zoom Window
- Matplotlib Plotting
- Matplotlib - Bar Graphs
- Matplotlib - Histogram
- Matplotlib - Pie Chart
- Matplotlib - Scatter Plot
- Matplotlib - Box Plot
- Matplotlib - Violin Plot
- Matplotlib - Contour Plot
- Matplotlib - 3D Plotting
- Matplotlib - 3D Contours
- Matplotlib - 3D Wireframe Plot
- Matplotlib - 3D Surface Plot
- Matplotlib - Quiver Plot
- Matplotlib Useful Resources
- Matplotlib - Quick Guide
- Matplotlib - Useful Resources
- Matplotlib - Discussion
Matplotlib - Figures
In Matplotlib library a figure is the top-level container that holds all elements of a plot or visualization. It can be thought of as the window or canvas where plots are created. A single figure can contain multiple subplots i.e. axes, titles, labels, legends and other elements. The figure() function in Matplotlib is used to create a new figure.
Syntax
The below is the syntax and parameters of the figure() method.
plt.figure(figsize=(width, height), dpi=resolution)
Where,
figsize=(width, height) − Specifies the width and height of the figure in inches. This parameter is optional.
dpi=resolution − Sets the resolution or dots per inch for the figure. Optional and by default is 100.
Creating a Figure
To create the Figure by using the figure() method we have to pass the figure size and resolution values as the input parameters.
Example
import matplotlib.pyplot as plt # Create a figure plt.figure(figsize=(3,3), dpi=100) plt.show()
Output
Figure size 300x300 with 0 Axes
Adding Plots to a Figure
After creating a figure we can add plots or subplots (axes) within that figure using various plot() or subplot() functions in Matplotlib.
Example
import matplotlib.pyplot as plt # Create a figure plt.figure(figsize=(3, 3), dpi=100) x = [12,4,56,77] y = [23,5,7,21] plt.plot(x,y) plt.show()
Output

Displaying and Customizing Figures
To display and customize the figures we have the functions plt.show(),plt.title(), plt.xlabel(), plt.ylabel(), plt.legend().
plt.show()
This function displays the figure with all the added plots and elements.
Customization
We can perform customizations such as adding titles, labels, legends and other elements to the figure using functions like plt.title(), plt.xlabel(), plt.ylabel(), plt.legend() etc.
Example
In this example we are using the figure() method of the pyplot module by passing the figsize as (8,6) and dpi as 100 to create a figure with a line plot and includes customization options such as a title, labels and a legend. Figures can contain multiple plots or subplots allowing for complex visualizations within a single window.
import matplotlib.pyplot as plt # Data x = [1, 2, 3, 4, 5] y = [2, 4, 6, 8, 10] # Create a figure plt.figure(figsize=(8, 6), dpi=100) # Add a line plot to the figure plt.plot(x, y, label='Line Plot') # Customize the plot plt.title('Figure with Line Plot') plt.xlabel('X-axis') plt.ylabel('Y-axis') plt.legend() # Display the figure plt.show()
Output
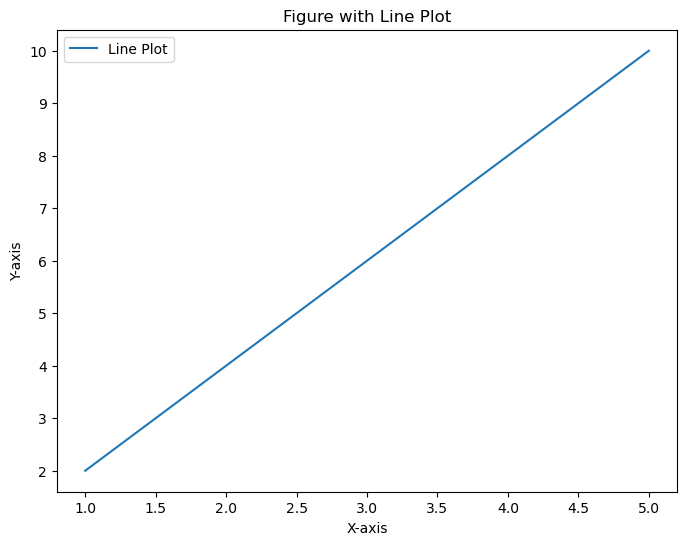
Example
Here this is another example of using the figure() method of the pyplot module to create the subplots.
import matplotlib.pyplot as plt # Create a figure plt.figure(figsize=(8, 6)) # Add plots or subplots within the figure plt.plot([1, 2, 3], [2, 4, 6], label='Line 1') plt.scatter([1, 2, 3], [3, 5, 7], label='Points') # Customize the figure plt.title('Example Figure') plt.xlabel('X-axis') plt.ylabel('Y-axis') plt.legend() # Display the figure plt.show()
Output
