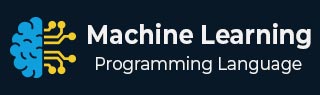
- Machine Learning Basics
- Machine Learning - Home
- Machine Learning - Getting Started
- Machine Learning - Basic Concepts
- Machine Learning - Python Libraries
- Machine Learning - Applications
- Machine Learning - Life Cycle
- Machine Learning - Required Skills
- Machine Learning - Implementation
- Machine Learning - Challenges & Common Issues
- Machine Learning - Limitations
- Machine Learning - Reallife Examples
- Machine Learning - Data Structure
- Machine Learning - Mathematics
- Machine Learning - Artificial Intelligence
- Machine Learning - Neural Networks
- Machine Learning - Deep Learning
- Machine Learning - Getting Datasets
- Machine Learning - Categorical Data
- Machine Learning - Data Loading
- Machine Learning - Data Understanding
- Machine Learning - Data Preparation
- Machine Learning - Models
- Machine Learning - Supervised
- Machine Learning - Unsupervised
- Machine Learning - Semi-supervised
- Machine Learning - Reinforcement
- Machine Learning - Supervised vs. Unsupervised
- Machine Learning Data Visualization
- Machine Learning - Data Visualization
- Machine Learning - Histograms
- Machine Learning - Density Plots
- Machine Learning - Box and Whisker Plots
- Machine Learning - Correlation Matrix Plots
- Machine Learning - Scatter Matrix Plots
- Statistics for Machine Learning
- Machine Learning - Statistics
- Machine Learning - Mean, Median, Mode
- Machine Learning - Standard Deviation
- Machine Learning - Percentiles
- Machine Learning - Data Distribution
- Machine Learning - Skewness and Kurtosis
- Machine Learning - Bias and Variance
- Machine Learning - Hypothesis
- Regression Analysis In ML
- Machine Learning - Regression Analysis
- Machine Learning - Linear Regression
- Machine Learning - Simple Linear Regression
- Machine Learning - Multiple Linear Regression
- Machine Learning - Polynomial Regression
- Classification Algorithms In ML
- Machine Learning - Classification Algorithms
- Machine Learning - Logistic Regression
- Machine Learning - K-Nearest Neighbors (KNN)
- Machine Learning - Naïve Bayes Algorithm
- Machine Learning - Decision Tree Algorithm
- Machine Learning - Support Vector Machine
- Machine Learning - Random Forest
- Machine Learning - Confusion Matrix
- Machine Learning - Stochastic Gradient Descent
- Clustering Algorithms In ML
- Machine Learning - Clustering Algorithms
- Machine Learning - Centroid-Based Clustering
- Machine Learning - K-Means Clustering
- Machine Learning - K-Medoids Clustering
- Machine Learning - Mean-Shift Clustering
- Machine Learning - Hierarchical Clustering
- Machine Learning - Density-Based Clustering
- Machine Learning - DBSCAN Clustering
- Machine Learning - OPTICS Clustering
- Machine Learning - HDBSCAN Clustering
- Machine Learning - BIRCH Clustering
- Machine Learning - Affinity Propagation
- Machine Learning - Distribution-Based Clustering
- Machine Learning - Agglomerative Clustering
- Dimensionality Reduction In ML
- Machine Learning - Dimensionality Reduction
- Machine Learning - Feature Selection
- Machine Learning - Feature Extraction
- Machine Learning - Backward Elimination
- Machine Learning - Forward Feature Construction
- Machine Learning - High Correlation Filter
- Machine Learning - Low Variance Filter
- Machine Learning - Missing Values Ratio
- Machine Learning - Principal Component Analysis
- Machine Learning Miscellaneous
- Machine Learning - Performance Metrics
- Machine Learning - Automatic Workflows
- Machine Learning - Boost Model Performance
- Machine Learning - Gradient Boosting
- Machine Learning - Bootstrap Aggregation (Bagging)
- Machine Learning - Cross Validation
- Machine Learning - AUC-ROC Curve
- Machine Learning - Grid Search
- Machine Learning - Data Scaling
- Machine Learning - Train and Test
- Machine Learning - Association Rules
- Machine Learning - Apriori Algorithm
- Machine Learning - Gaussian Discriminant Analysis
- Machine Learning - Cost Function
- Machine Learning - Bayes Theorem
- Machine Learning - Precision and Recall
- Machine Learning - Adversarial
- Machine Learning - Stacking
- Machine Learning - Epoch
- Machine Learning - Perceptron
- Machine Learning - Regularization
- Machine Learning - Overfitting
- Machine Learning - P-value
- Machine Learning - Entropy
- Machine Learning - MLOps
- Machine Learning - Data Leakage
- Machine Learning - Resources
- Machine Learning - Quick Guide
- Machine Learning - Useful Resources
- Machine Learning - Discussion
Machine Learning - Epoch
In machine learning, an epoch refers to a complete iteration over the entire training dataset during the model training process. In simpler terms, it is the number of times the algorithm goes through the entire dataset during the training phase.
During the training process, the algorithm makes predictions on the training data, computes the loss, and updates the model parameters to reduce the loss. The objective is to optimize the model's performance by minimizing the loss function. One epoch is considered complete when the model has made predictions on all the training data.
Epochs are an essential parameter in the training process as they can significantly affect the performance of the model. Setting the number of epochs too low can result in an underfit model, while setting it too high can lead to overfitting.
Underfitting occurs when the model fails to capture the underlying patterns in the data and performs poorly on both the training and testing datasets. It happens when the model is too simple or not trained enough. In such cases, increasing the number of epochs can help the model learn more from the data and improve its performance.
Overfitting, on the other hand, happens when the model learns the noise in the training data and performs well on the training set but poorly on the testing data. It occurs when the model is too complex or trained for too many epochs. To avoid overfitting, the number of epochs must be limited, and other regularization techniques like early stopping or dropout should be used.
Implementation in Python
In Python, the number of epochs is specified in the training loop of the machine learning model. For example, when training a neural network using the Keras library, you can set the number of epochs using the "epochs" argument in the "fit" method.
Example
# import necessary libraries import numpy as np from keras.models import Sequential from keras.layers import Dense # generate some random data for training X_train = np.random.rand(100, 10) y_train = np.random.randint(0, 2, size=(100,)) # create a neural network model model = Sequential() model.add(Dense(16, input_dim=10, activation='relu')) model.add(Dense(1, activation='sigmoid')) # compile the model with binary cross-entropy loss and adam optimizer model.compile(loss='binary_crossentropy', optimizer='adam', metrics=['accuracy']) # train the model with 10 epochs model.fit(X_train, y_train, epochs=10)
In this example, we generate some random data for training and create a simple neural network model with one input layer, one hidden layer, and one output layer. We compile the model with binary cross-entropy loss and the Adam optimizer and set the number of epochs to 10 in the "fit" method.
During the training process, the model makes predictions on the training data, computes the loss, and updates the weights to minimize the loss. After completing 10 epochs, the model is considered trained, and we can use it to make predictions on new, unseen data.
Output
When you execute this code, it will produce an output like this −
Epoch 1/10 4/4 [==============================] - 31s 2ms/step - loss: 0.7012 - accuracy: 0.4976 Epoch 2/10 4/4 [==============================] - 0s 1ms/step - loss: 0.6995 - accuracy: 0.4390 Epoch 3/10 4/4 [==============================] - 0s 1ms/step - loss: 0.6921 - accuracy: 0.5123 Epoch 4/10 4/4 [==============================] - 0s 1ms/step - loss: 0.6778 - accuracy: 0.5474 Epoch 5/10 4/4 [==============================] - 0s 1ms/step - loss: 0.6819 - accuracy: 0.5542 Epoch 6/10 4/4 [==============================] - 0s 1ms/step - loss: 0.6795 - accuracy: 0.5377 Epoch 7/10 4/4 [==============================] - 0s 1ms/step - loss: 0.6840 - accuracy: 0.5303 Epoch 8/10 4/4 [==============================] - 0s 1ms/step - loss: 0.6795 - accuracy: 0.5554 Epoch 9/10 4/4 [==============================] - 0s 1ms/step - loss: 0.6706 - accuracy: 0.5545 Epoch 10/10 4/4 [==============================] - 0s 1ms/step - loss: 0.6722 - accuracy: 0.5556