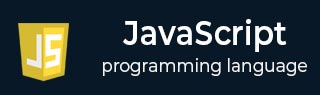
- Javascript Basics Tutorial
- Javascript - Home
- JavaScript - Overview
- JavaScript - Features
- JavaScript - Enabling
- JavaScript - Placement
- JavaScript - Syntax
- JavaScript - Hello World
- JavaScript - Console.log()
- JavaScript - Comments
- JavaScript - Variables
- JavaScript - let Statement
- JavaScript - Constants
- JavaScript - Data Types
- JavaScript - Type Conversions
- JavaScript - Strict Mode
- JavaScript - Reserved Keywords
- JavaScript Operators
- JavaScript - Operators
- JavaScript - Arithmetic Operators
- JavaScript - Comparison Operators
- JavaScript - Logical Operators
- JavaScript - Bitwise Operators
- JavaScript - Assignment Operators
- JavaScript - Conditional Operators
- JavaScript - typeof Operator
- JavaScript - Nullish Coalescing Operator
- JavaScript - Delete Operator
- JavaScript - Comma Operator
- JavaScript - Grouping Operator
- JavaScript - Yield Operator
- JavaScript - Spread Operator
- JavaScript - Exponentiation Operator
- JavaScript - Operator Precedence
- JavaScript Control Flow
- JavaScript - If...Else
- JavaScript - While Loop
- JavaScript - For Loop
- JavaScript - For...in
- Javascript - For...of
- JavaScript - Loop Control
- JavaScript - Break Statement
- JavaScript - Continue Statement
- JavaScript - Switch Case
- JavaScript - User Defined Iterators
- JavaScript Functions
- JavaScript - Functions
- JavaScript - Function Expressions
- JavaScript - Function Parameters
- JavaScript - Default Parameters
- JavaScript - Function() Constructor
- JavaScript - Function Hoisting
- JavaScript - Self-Invoking Functions
- JavaScript - Arrow Functions
- JavaScript - Function Invocation
- JavaScript - Function call()
- JavaScript - Function apply()
- JavaScript - Function bind()
- JavaScript - Closures
- JavaScript - Variable Scope
- JavaScript - Global Variables
- JavaScript - Smart Function Parameters
- JavaScript Objects
- JavaScript - Number
- JavaScript - Boolean
- JavaScript - Strings
- JavaScript - Arrays
- JavaScript - Date
- JavaScript - DataView
- JavaScript - Math
- JavaScript - RegExp
- JavaScript - Symbol
- JavaScript - Sets
- JavaScript - WeakSet
- JavaScript - Maps
- JavaScript - WeakMap
- JavaScript - Iterables
- JavaScript - Reflect
- JavaScript - TypedArray
- JavaScript - Template Literals
- JavaScript - Tagged Templates
- Object Oriented JavaScript
- JavaScript - Objects
- JavaScript - Classes
- JavaScript - Object Properties
- JavaScript - Object Methods
- JavaScript - Static Methods
- JavaScript - Display Objects
- JavaScript - Object Accessors
- JavaScript - Object Constructors
- JavaScript - Native Prototypes
- JavaScript - ES5 Object Methods
- JavaScript - Encapsulation
- JavaScript - Inheritance
- JavaScript - Abstraction
- JavaScript - Polymorphism
- JavaScript - Destructuring Assignment
- JavaScript - Object Destructuring
- JavaScript - Array Destructuring
- JavaScript - Nested Destructuring
- JavaScript - Optional Chaining
- JavaScript - Global Object
- JavaScript - Mixins
- JavaScript - Proxies
- JavaScript Versions
- JavaScript - History
- JavaScript - Versions
- JavaScript - ES5
- JavaScript - ES6
- ECMAScript 2016
- ECMAScript 2017
- ECMAScript 2018
- ECMAScript 2019
- ECMAScript 2020
- ECMAScript 2021
- ECMAScript 2022
- JavaScript Cookies
- JavaScript - Cookies
- JavaScript - Cookie Attributes
- JavaScript - Deleting Cookies
- JavaScript Browser BOM
- JavaScript - Browser Object Model
- JavaScript - Window Object
- JavaScript - Document Object
- JavaScript - Screen Object
- JavaScript - History Object
- JavaScript - Navigator Object
- JavaScript - Location Object
- JavaScript - Console Object
- JavaScript Web APIs
- JavaScript - Web API
- JavaScript - History API
- JavaScript - Storage API
- JavaScript - Forms API
- JavaScript - Worker API
- JavaScript - Fetch API
- JavaScript - Geolocation API
- JavaScript Events
- JavaScript - Events
- JavaScript - DOM Events
- JavaScript - addEventListener()
- JavaScript - Mouse Events
- JavaScript - Keyboard Events
- JavaScript - Form Events
- JavaScript - Window/Document Events
- JavaScript - Event Delegation
- JavaScript - Event Bubbling
- JavaScript - Event Capturing
- JavaScript - Custom Events
- JavaScript Error Handling
- JavaScript - Error Handling
- JavaScript - try...catch
- JavaScript - Debugging
- JavaScript - Custom Errors
- JavaScript - Extending Errors
- JavaScript Important Keywords
- JavaScript - this Keyword
- JavaScript - void Keyword
- JavaScript - new Keyword
- JavaScript - var Keyword
- JavaScript HTML DOM
- JavaScript - HTML DOM
- JavaScript - DOM Methods
- JavaScript - DOM Document
- JavaScript - DOM Elements
- JavaScript - DOM Forms
- JavaScript - Changing HTML
- JavaScript - Changing CSS
- JavaScript - DOM Animation
- JavaScript - DOM Navigation
- JavaScript - DOM Collections
- JavaScript - DOM Node Lists
- JavaScript Miscellaneous
- JavaScript - Ajax
- JavaScript - Async Iteration
- JavaScript - Atomics Objects
- JavaScript - Rest Parameter
- JavaScript - Page Redirect
- JavaScript - Dialog Boxes
- JavaScript - Page Printing
- JavaScript - Validations
- JavaScript - Animation
- JavaScript - Multimedia
- JavaScript - Image Map
- JavaScript - Browsers
- JavaScript - JSON
- JavaScript - Multiline Strings
- JavaScript - Date Formats
- JavaScript - Get Date Methods
- JavaScript - Set Date Methods
- JavaScript - Modules
- JavaScript - Dynamic Imports
- JavaScript - BigInt
- JavaScript - Blob
- JavaScript - Unicode
- JavaScript - Shallow Copy
- JavaScript - Call Stack
- JavaScript - Reference Type
- JavaScript - IndexedDB
- JavaScript - Clickjacking Attack
- JavaScript - Currying
- JavaScript - Graphics
- JavaScript - Canvas
- JavaScript - Debouncing
- JavaScript - Performance
- JavaScript - Style Guide
- JavaScript Useful Resources
- JavaScript - Questions And Answers
- JavaScript - Quick Guide
- JavaScript - Functions
- JavaScript - Resources
JavaScript - Window/Document Events
JavaScript window events are actions that occur when the user does something affecting the entire browser window, like loading, resizing, closing, or moving the window. The most common window event is simply loading the window by opening a particular web page. This event is handled by the onload event handler.
Developers can use JavaScript to create dynamic, interactive web pages that respond to user actions. The interactivity depends on two core aspects: window events and document events. Operating at the browser's panoramic level, window events bestow control over the overall state of the browser window; alternatively, document events interact with the HTML document, empowering developers to react specifically towards elements or actions within a page
Window Events
At the browser level, window events happen and hold association with the window object; this global object represents the web browser's window. Frequently employed to oversee the overall state of a browser window or manage global interactions are these types of events.
Event Name | Description |
---|---|
load | Triggered when the entire web page, including all its resources, has finished loading. |
unload | Fired when the user is leaving the page or closing the browser window or tab. |
resize | Activated when the size of the browser window is changed. |
scroll | Fired when the user scrolls the page. |
Example: Demonstrating Window Events
In this example, a script actively listens for the 'load,' 'resize,' and 'scroll' events on the window; it includes an initial page load alert to inform users that loading is complete. Should they subsequently resize their window, an alert will trigger thereby displaying the new size of their updated viewport. Moreover, when the user scrolls on the page, an alert is triggered to indicate their action.
<!DOCTYPE html> <html> <head> <title>Window Events Example</title> <style> body { height: 2000px; /* Adding some content just to enable scrolling */ } #resizeInfo { position: fixed; top: 10px; left: 10px; background-color: #fff; padding: 10px; border: 1px solid #ccc; } </style> <script> window.addEventListener('load', function() { var initialSizeInfo = 'Initial window size: ' + window.innerWidth + ' x ' + window.innerHeight; document.getElementById('resizeInfo').innerText = initialSizeInfo; alert('The page has finished loading!'); }); window.addEventListener('resize', function() { var newSizeInfo = 'New window size: ' + window.innerWidth + ' x ' + window.innerHeight; document.getElementById('resizeInfo').innerText = newSizeInfo; alert("Page has been resized"); }); window.addEventListener('scroll', function() { alert('You have scrolled on this page.'); },{once:true}); </script> </head> <body> <div id="resizeInfo">Initial window size: ${window.innerWidth} x ${window.innerHeight}</div> </body> </html>
Document Events
Document events on the other hand occur at the level of the HTML document within the window and are associated with the document object which represents the HTML document thereby providing an interface to interact with the content of the page.
Event Name | Description |
---|---|
DOMContentLoaded | Triggered when the HTML document has been completely loaded and parsed, without waiting for external resources like images. |
click | Fired when the user clicks on an element. |
submit | Triggered when a form is submitted. |
keydown/keyup/keypress | These events are triggered when a key is pressed, released, or both, respectively. |
change | Fired when the value of an input element changes, such as with text inputs or dropdowns. |
Example: Demonstrating Document Events
In this example, we've included a script that listens for the 'DOMContentLoaded,' 'click,' 'submit,' and 'keydown' events on the document. Upon the 'DOMContentLoaded' event, it logs to the console that the DOM content has been fully loaded. Subsequently, clicking an element triggers an alert displaying the tag name of the clicked element. Submitting a form also alerts that the form has been submitted. Furthermore, pressing a key like entering the username with characters, alerts every keypress to the screen.
<!DOCTYPE html> <html> <head> <title>Document Events Example</title> <script> document.addEventListener('DOMContentLoaded', function() { alert('DOM content has been fully loaded!'); }); document.addEventListener('click', function(event) { alert('Element clicked! Tag Name: ' + event.target.tagName); },{once:true}); document.addEventListener('submit', function() { alert('Form submitted!'); }); document.addEventListener('keydown', function(event) { alert('Key pressed: ' + event.key); },{once:true}); </script> </head> <body> <form> <label for="username">Username:</label> <input type="text" id="username" name="username"> <button type="submit">Submit</button> </form> </body> </html>