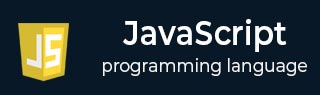
- Javascript Basics Tutorial
- Javascript - Home
- JavaScript - Overview
- JavaScript - Features
- JavaScript - Enabling
- JavaScript - Placement
- JavaScript - Syntax
- JavaScript - Hello World
- JavaScript - Console.log()
- JavaScript - Comments
- JavaScript - Variables
- JavaScript - let Statement
- JavaScript - Constants
- JavaScript - Data Types
- JavaScript - Type Conversions
- JavaScript - Strict Mode
- JavaScript - Reserved Keywords
- JavaScript Operators
- JavaScript - Operators
- JavaScript - Arithmetic Operators
- JavaScript - Comparison Operators
- JavaScript - Logical Operators
- JavaScript - Bitwise Operators
- JavaScript - Assignment Operators
- JavaScript - Conditional Operators
- JavaScript - typeof Operator
- JavaScript - Nullish Coalescing Operator
- JavaScript - Delete Operator
- JavaScript - Comma Operator
- JavaScript - Grouping Operator
- JavaScript - Yield Operator
- JavaScript - Spread Operator
- JavaScript - Exponentiation Operator
- JavaScript - Operator Precedence
- JavaScript Control Flow
- JavaScript - If...Else
- JavaScript - While Loop
- JavaScript - For Loop
- JavaScript - For...in
- Javascript - For...of
- JavaScript - Loop Control
- JavaScript - Break Statement
- JavaScript - Continue Statement
- JavaScript - Switch Case
- JavaScript - User Defined Iterators
- JavaScript Functions
- JavaScript - Functions
- JavaScript - Function Expressions
- JavaScript - Function Parameters
- JavaScript - Default Parameters
- JavaScript - Function() Constructor
- JavaScript - Function Hoisting
- JavaScript - Self-Invoking Functions
- JavaScript - Arrow Functions
- JavaScript - Function Invocation
- JavaScript - Function call()
- JavaScript - Function apply()
- JavaScript - Function bind()
- JavaScript - Closures
- JavaScript - Variable Scope
- JavaScript - Global Variables
- JavaScript - Smart Function Parameters
- JavaScript Objects
- JavaScript - Number
- JavaScript - Boolean
- JavaScript - Strings
- JavaScript - Arrays
- JavaScript - Date
- JavaScript - DataView
- JavaScript - Math
- JavaScript - RegExp
- JavaScript - Symbol
- JavaScript - Sets
- JavaScript - WeakSet
- JavaScript - Maps
- JavaScript - WeakMap
- JavaScript - Iterables
- JavaScript - Reflect
- JavaScript - TypedArray
- JavaScript - Template Literals
- JavaScript - Tagged Templates
- Object Oriented JavaScript
- JavaScript - Objects
- JavaScript - Classes
- JavaScript - Object Properties
- JavaScript - Object Methods
- JavaScript - Static Methods
- JavaScript - Display Objects
- JavaScript - Object Accessors
- JavaScript - Object Constructors
- JavaScript - Native Prototypes
- JavaScript - ES5 Object Methods
- JavaScript - Encapsulation
- JavaScript - Inheritance
- JavaScript - Abstraction
- JavaScript - Polymorphism
- JavaScript - Destructuring Assignment
- JavaScript - Object Destructuring
- JavaScript - Array Destructuring
- JavaScript - Nested Destructuring
- JavaScript - Optional Chaining
- JavaScript - Global Object
- JavaScript - Mixins
- JavaScript - Proxies
- JavaScript Versions
- JavaScript - History
- JavaScript - Versions
- JavaScript - ES5
- JavaScript - ES6
- ECMAScript 2016
- ECMAScript 2017
- ECMAScript 2018
- ECMAScript 2019
- ECMAScript 2020
- ECMAScript 2021
- ECMAScript 2022
- JavaScript Cookies
- JavaScript - Cookies
- JavaScript - Cookie Attributes
- JavaScript - Deleting Cookies
- JavaScript Browser BOM
- JavaScript - Browser Object Model
- JavaScript - Window Object
- JavaScript - Document Object
- JavaScript - Screen Object
- JavaScript - History Object
- JavaScript - Navigator Object
- JavaScript - Location Object
- JavaScript - Console Object
- JavaScript Web APIs
- JavaScript - Web API
- JavaScript - History API
- JavaScript - Storage API
- JavaScript - Forms API
- JavaScript - Worker API
- JavaScript - Fetch API
- JavaScript - Geolocation API
- JavaScript Events
- JavaScript - Events
- JavaScript - DOM Events
- JavaScript - addEventListener()
- JavaScript - Mouse Events
- JavaScript - Keyboard Events
- JavaScript - Form Events
- JavaScript - Window/Document Events
- JavaScript - Event Delegation
- JavaScript - Event Bubbling
- JavaScript - Event Capturing
- JavaScript - Custom Events
- JavaScript Error Handling
- JavaScript - Error Handling
- JavaScript - try...catch
- JavaScript - Debugging
- JavaScript - Custom Errors
- JavaScript - Extending Errors
- JavaScript Important Keywords
- JavaScript - this Keyword
- JavaScript - void Keyword
- JavaScript - new Keyword
- JavaScript - var Keyword
- JavaScript HTML DOM
- JavaScript - HTML DOM
- JavaScript - DOM Methods
- JavaScript - DOM Document
- JavaScript - DOM Elements
- JavaScript - DOM Forms
- JavaScript - Changing HTML
- JavaScript - Changing CSS
- JavaScript - DOM Animation
- JavaScript - DOM Navigation
- JavaScript - DOM Collections
- JavaScript - DOM Node Lists
- JavaScript Miscellaneous
- JavaScript - Ajax
- JavaScript - Async Iteration
- JavaScript - Atomics Objects
- JavaScript - Rest Parameter
- JavaScript - Page Redirect
- JavaScript - Dialog Boxes
- JavaScript - Page Printing
- JavaScript - Validations
- JavaScript - Animation
- JavaScript - Multimedia
- JavaScript - Image Map
- JavaScript - Browsers
- JavaScript - JSON
- JavaScript - Multiline Strings
- JavaScript - Date Formats
- JavaScript - Get Date Methods
- JavaScript - Set Date Methods
- JavaScript - Modules
- JavaScript - Dynamic Imports
- JavaScript - BigInt
- JavaScript - Blob
- JavaScript - Unicode
- JavaScript - Shallow Copy
- JavaScript - Call Stack
- JavaScript - Reference Type
- JavaScript - IndexedDB
- JavaScript - Clickjacking Attack
- JavaScript - Currying
- JavaScript - Graphics
- JavaScript - Canvas
- JavaScript - Debouncing
- JavaScript - Performance
- JavaScript - Style Guide
- JavaScript Useful Resources
- JavaScript - Questions And Answers
- JavaScript - Quick Guide
- JavaScript - Functions
- JavaScript - Resources
JavaScript - Navigator Object
Window Navigator Object
The navigator object in JavaScript is used to access the information of the user's browser. Using the 'navigator' object, you can get the browser version and name and check whether the cookie is enabled in the browser.
The 'navigator' object is a property of the window object. The navigator object can be accessed using the read-only window.navigator property.
Navigator Object Properties
There are many properties of navigator object that can be used to access the information about the user's browser.
Syntax
Follow the syntax below to use to access a property of navigator object in JavaScript.
window.navigator.proeprty; OR navigator.property;
You may use the 'window' object to access the 'navigator' object.
Here, we have listed all properties of the Navigator object.
Property | Description |
---|---|
appName | It gives you a browser name. |
appVersion | It gives you the browser version. |
appCodeName | It gives you the browser code name. |
cookieEnabled | It returns a boolean value based on whether the cookie is enabled. |
language | It returns the browser language. It is supported by Firefox and Netscape only. |
plugins | It returns the browser plugins. It is supported by Firefox and Netscape only. |
mimeTypes[] | It gives you an array of Mime types. It is supported by Firefox and Netscape only. |
platform | It gives you a platform or operating system in which the browser is used. |
online | Returns a boolean value based on whether the browser is online. |
product | It gives you a browser engine. |
userAgent | It gives you a user-agent header of the browser. |
Example: Accessing Navigator Object Properties
We used the different properties in the below code to get the browser information.
The appName property returns the browser's name, appCodeName returns the code name of the browser, appVersion returns the browser's version, and the cookieEnabled property checks whether the cookies are enabled in the browser.
<html> <body> <p> Browser Information</p> <p id = "demo"> </p> <script> document.getElementById("demo").innerHTML = "App Name: " + navigator.appName + "<br>" + "App Code Name: " + navigator.appCodeName + "<br>" + "App Version: " + navigator.appVersion + "<br>" + "Cookie Enabled: " + navigator.cookieEnabled + "<br>" + "Language: " + navigator.language + "<br>" + "Plugins: " + navigator.plugins + "<br>" + "mimeTypes[]: " + navigator.mimeTypes + "<br>" + "platform: " + navigator.platform + "<br>" + "online: " + navigator.online + "<br>" + "product: " + navigator.product + "<br>" + "userAgent: " + navigator.userAgent; </script> <p>Please note you may get different result depending on your browser. </p> </body> </html>
Output
Browser Information App Name: Netscape App Code Name: Mozilla App Version: 5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/102.0.0.0 Safari/537.36 Cookie Enabled: true Language: en-US Plugins: [object PluginArray] mimeTypes[]: [object MimeTypeArray] platform: Win32 online: undefined product: Gecko userAgent: Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/102.0.0.0 Safari/537.36 Please note you may get different result depending on your browser.
Example
In the example below, we accessed the navigator object as a property of the window object. Then we accessed different properties of this navigator object.
<html> <body> <p> Browser Information</p> <p id = "demo"> </p> <script> document.getElementById("demo").innerHTML = "App Name: " + window.navigator.appName + "<br>" + "App Code Name: " + window.navigator.appCodeName + "<br>" + "App Version: " + window.navigator.appVersion + "<br>" + "Cookie Enabled: " + window.navigator.cookieEnabled + "<br>" + "Language: " + window.navigator.language + "<br>" + "Plugins: " + window.navigator.plugins + "<br>" + "mimeTypes[]: " + window.navigator.mimeTypes + "<br>" + "platform: " + window.navigator.platform + "<br>" + "online: " + window.navigator.online + "<br>" + "product: " + window.navigator.product + "<br>" + "userAgent: " + window.navigator.userAgent; </script> <p>Please note you may get different result depending on your browser. </p> </body> </html>
Output
Browser Information App Name: Netscape App Code Name: Mozilla App Version: 5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/102.0.0.0 Safari/537.36 Cookie Enabled: true Language: en-US Plugins: [object PluginArray] mimeTypes[]: [object MimeTypeArray] platform: Win32 online: undefined product: Gecko userAgent: Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/102.0.0.0 Safari/537.36 Please note you may get different result depending on your browser.
JavaScript Navigator Object Methods
The Navigator object contains only one method.
Method | Description |
---|---|
javaEnabled() | It checks whether the Java is enabled in the web browser. |
Example: Navigator javaEnabled() Method
In the below code, we used the javaEnabled() method of the navigator object to check whether the java is enabled in the browser.
<html> <body> <p id = "output"> </p> <script> const output = document.getElementById("output"); if (navigator.javaEnabled()) { output.innerHTML += "Java is enabled in the browser!"; } else { output.innerHTML += "Please, enable the Java!"; } </script> <p>Please note you may get different result depending on your browser. </p> </body> </html>
Output
Please, enable the Java! Please note you may get different result depending on your browser.