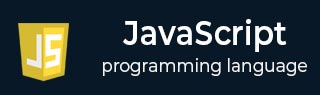
- Javascript Basics Tutorial
- Javascript - Home
- JavaScript - Overview
- JavaScript - Features
- JavaScript - Enabling
- JavaScript - Placement
- JavaScript - Syntax
- JavaScript - Hello World
- JavaScript - Console.log()
- JavaScript - Comments
- JavaScript - Variables
- JavaScript - let Statement
- JavaScript - Constants
- JavaScript - Data Types
- JavaScript - Type Conversions
- JavaScript - Strict Mode
- JavaScript - Reserved Keywords
- JavaScript Operators
- JavaScript - Operators
- JavaScript - Arithmetic Operators
- JavaScript - Comparison Operators
- JavaScript - Logical Operators
- JavaScript - Bitwise Operators
- JavaScript - Assignment Operators
- JavaScript - Conditional Operators
- JavaScript - typeof Operator
- JavaScript - Nullish Coalescing Operator
- JavaScript - Delete Operator
- JavaScript - Comma Operator
- JavaScript - Grouping Operator
- JavaScript - Yield Operator
- JavaScript - Spread Operator
- JavaScript - Exponentiation Operator
- JavaScript - Operator Precedence
- JavaScript Control Flow
- JavaScript - If...Else
- JavaScript - While Loop
- JavaScript - For Loop
- JavaScript - For...in
- Javascript - For...of
- JavaScript - Loop Control
- JavaScript - Break Statement
- JavaScript - Continue Statement
- JavaScript - Switch Case
- JavaScript - User Defined Iterators
- JavaScript Functions
- JavaScript - Functions
- JavaScript - Function Expressions
- JavaScript - Function Parameters
- JavaScript - Default Parameters
- JavaScript - Function() Constructor
- JavaScript - Function Hoisting
- JavaScript - Self-Invoking Functions
- JavaScript - Arrow Functions
- JavaScript - Function Invocation
- JavaScript - Function call()
- JavaScript - Function apply()
- JavaScript - Function bind()
- JavaScript - Closures
- JavaScript - Variable Scope
- JavaScript - Global Variables
- JavaScript - Smart Function Parameters
- JavaScript Objects
- JavaScript - Number
- JavaScript - Boolean
- JavaScript - Strings
- JavaScript - Arrays
- JavaScript - Date
- JavaScript - DataView
- JavaScript - Math
- JavaScript - RegExp
- JavaScript - Symbol
- JavaScript - Sets
- JavaScript - WeakSet
- JavaScript - Maps
- JavaScript - WeakMap
- JavaScript - Iterables
- JavaScript - Reflect
- JavaScript - TypedArray
- JavaScript - Template Literals
- JavaScript - Tagged Templates
- Object Oriented JavaScript
- JavaScript - Objects
- JavaScript - Classes
- JavaScript - Object Properties
- JavaScript - Object Methods
- JavaScript - Static Methods
- JavaScript - Display Objects
- JavaScript - Object Accessors
- JavaScript - Object Constructors
- JavaScript - Native Prototypes
- JavaScript - ES5 Object Methods
- JavaScript - Encapsulation
- JavaScript - Inheritance
- JavaScript - Abstraction
- JavaScript - Polymorphism
- JavaScript - Destructuring Assignment
- JavaScript - Object Destructuring
- JavaScript - Array Destructuring
- JavaScript - Nested Destructuring
- JavaScript - Optional Chaining
- JavaScript - Global Object
- JavaScript - Mixins
- JavaScript - Proxies
- JavaScript Versions
- JavaScript - History
- JavaScript - Versions
- JavaScript - ES5
- JavaScript - ES6
- ECMAScript 2016
- ECMAScript 2017
- ECMAScript 2018
- ECMAScript 2019
- ECMAScript 2020
- ECMAScript 2021
- ECMAScript 2022
- JavaScript Cookies
- JavaScript - Cookies
- JavaScript - Cookie Attributes
- JavaScript - Deleting Cookies
- JavaScript Browser BOM
- JavaScript - Browser Object Model
- JavaScript - Window Object
- JavaScript - Document Object
- JavaScript - Screen Object
- JavaScript - History Object
- JavaScript - Navigator Object
- JavaScript - Location Object
- JavaScript - Console Object
- JavaScript Web APIs
- JavaScript - Web API
- JavaScript - History API
- JavaScript - Storage API
- JavaScript - Forms API
- JavaScript - Worker API
- JavaScript - Fetch API
- JavaScript - Geolocation API
- JavaScript Events
- JavaScript - Events
- JavaScript - DOM Events
- JavaScript - addEventListener()
- JavaScript - Mouse Events
- JavaScript - Keyboard Events
- JavaScript - Form Events
- JavaScript - Window/Document Events
- JavaScript - Event Delegation
- JavaScript - Event Bubbling
- JavaScript - Event Capturing
- JavaScript - Custom Events
- JavaScript Error Handling
- JavaScript - Error Handling
- JavaScript - try...catch
- JavaScript - Debugging
- JavaScript - Custom Errors
- JavaScript - Extending Errors
- JavaScript Important Keywords
- JavaScript - this Keyword
- JavaScript - void Keyword
- JavaScript - new Keyword
- JavaScript - var Keyword
- JavaScript HTML DOM
- JavaScript - HTML DOM
- JavaScript - DOM Methods
- JavaScript - DOM Document
- JavaScript - DOM Elements
- JavaScript - DOM Forms
- JavaScript - Changing HTML
- JavaScript - Changing CSS
- JavaScript - DOM Animation
- JavaScript - DOM Navigation
- JavaScript - DOM Collections
- JavaScript - DOM Node Lists
- JavaScript Miscellaneous
- JavaScript - Ajax
- JavaScript - Async Iteration
- JavaScript - Atomics Objects
- JavaScript - Rest Parameter
- JavaScript - Page Redirect
- JavaScript - Dialog Boxes
- JavaScript - Page Printing
- JavaScript - Validations
- JavaScript - Animation
- JavaScript - Multimedia
- JavaScript - Image Map
- JavaScript - Browsers
- JavaScript - JSON
- JavaScript - Multiline Strings
- JavaScript - Date Formats
- JavaScript - Get Date Methods
- JavaScript - Set Date Methods
- JavaScript - Modules
- JavaScript - Dynamic Imports
- JavaScript - BigInt
- JavaScript - Blob
- JavaScript - Unicode
- JavaScript - Shallow Copy
- JavaScript - Call Stack
- JavaScript - Reference Type
- JavaScript - IndexedDB
- JavaScript - Clickjacking Attack
- JavaScript - Currying
- JavaScript - Graphics
- JavaScript - Canvas
- JavaScript - Debouncing
- JavaScript - Performance
- JavaScript - Style Guide
- JavaScript Useful Resources
- JavaScript - Questions And Answers
- JavaScript - Quick Guide
- JavaScript - Functions
- JavaScript - Resources
JavaScript - History Object
Window History Object
In JavaScript, the window 'history' object contains information about the browser's session history. It contains the array of visited URLs in the current session.
The 'history' object is a property of the 'window' object. The history property can be accessed with or without referencing its owner object, i.e., window object.
Using the 'history' object's method, you can go to the browser's session's previous, following, or particular URL.
History Object Methods
The window history object provides useful methods that allow us to navigate back and forth in the history list.
Follow the syntax below to use the 'history' object in JavaScript.
window.history.methodName(); OR history.methodName();
You may use the 'window' object to access the 'history' object.
JavaScript History back() Method
The JavaScript back() method of the history object loads the previous URL in the history list.
Syntax
Follow the syntax below to use the history back() method.
history.back();
Example
In the below code's output, you can click the 'go back' button to go to the previous URL. It works as a back button of the browser.
<html> <body> <p> Click "Go Back" button to load previous URL </p> <button onclick="goback()"> Go Back </button> <p id = "output"> </p> <script> function goback() { history.back(); document.getElementById("output").innerHTML += "You will have gone to previous URL if it exists"; } </script> </body> </html>
JavaScript History forward() Method
The forward() method of the history object takes you to the next URL.
Syntax
Follow the syntax below to use the forward() method.
history.forward();
Example
In the below code, click the button to go to the next URL. It works as the forward button of the browser.
<html> <body> <p> Click "Go Forward" button to load next URL</p> <button onclick = "goforward()"> Go Forward </button> <p id = "output"> </p> <script> function goforward() { history.forward(); document.getElementById("output").innerHTML = "You will have forwarded to next URL if it exists." } </script> </body> </html>
JavaScript History go() Method
The go() method of the history object takes you to the specific URL of the browser's session.
Syntax
Follow the syntax below to use the go() method.
history.go(count);
In the above syntax, 'count' is a numeric value representing which page you want to visit.
Example
In the below code, we use the go() method to move to the 2nd previous page from the current web page.
<html> <body> <p> Click the below button to load 2nd previous URL</p> <button onclick = "moveTo()"> Move at 2nd previous URL </button> <p id = "output"> </p> <script> function moveTo() { history.go(-2); document.getElementById("output").innerHTML = "You will have forwarded to 2nd previous URL if it exists."; } </script> </body> </html>
Example
In the below code, we use the go() method to move to the 2nd previous page from the current web page.
<html> <body> <p> Click the below button to load 2nd next URL</p> <button onclick = "moveTo()"> Move at 2nd next URL </button> <p id = "output"> </p> <script> function moveTo() { history.go(2); document.getElementById("output").innerHTML = "You will have forwarded to 2nd next URL if it exists."; } </script> </body> </html>
Following is the complete window history object reference including both properties and methods −
History Object Property List
The history object contains only the 'length' property.
Property | Description |
---|---|
length | It returns the object's length, representing the number of URLS present in the object. |
History Object Methods List
The history object contains the below 3 methods.
Method | Description |
---|---|
back() | It takes you to the previous web page. |
forward() | It takes you to the next web page. |
go() | It can take you to a specific web page. |