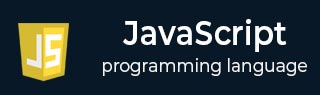
- Javascript Basics Tutorial
- Javascript - Home
- JavaScript - Overview
- JavaScript - Features
- JavaScript - Enabling
- JavaScript - Placement
- JavaScript - Syntax
- JavaScript - Hello World
- JavaScript - Console.log()
- JavaScript - Comments
- JavaScript - Variables
- JavaScript - let Statement
- JavaScript - Constants
- JavaScript - Data Types
- JavaScript - Type Conversions
- JavaScript - Strict Mode
- JavaScript - Reserved Keywords
- JavaScript Operators
- JavaScript - Operators
- JavaScript - Arithmetic Operators
- JavaScript - Comparison Operators
- JavaScript - Logical Operators
- JavaScript - Bitwise Operators
- JavaScript - Assignment Operators
- JavaScript - Conditional Operators
- JavaScript - typeof Operator
- JavaScript - Nullish Coalescing Operator
- JavaScript - Delete Operator
- JavaScript - Comma Operator
- JavaScript - Grouping Operator
- JavaScript - Yield Operator
- JavaScript - Spread Operator
- JavaScript - Exponentiation Operator
- JavaScript - Operator Precedence
- JavaScript Control Flow
- JavaScript - If...Else
- JavaScript - While Loop
- JavaScript - For Loop
- JavaScript - For...in
- Javascript - For...of
- JavaScript - Loop Control
- JavaScript - Break Statement
- JavaScript - Continue Statement
- JavaScript - Switch Case
- JavaScript - User Defined Iterators
- JavaScript Functions
- JavaScript - Functions
- JavaScript - Function Expressions
- JavaScript - Function Parameters
- JavaScript - Default Parameters
- JavaScript - Function() Constructor
- JavaScript - Function Hoisting
- JavaScript - Self-Invoking Functions
- JavaScript - Arrow Functions
- JavaScript - Function Invocation
- JavaScript - Function call()
- JavaScript - Function apply()
- JavaScript - Function bind()
- JavaScript - Closures
- JavaScript - Variable Scope
- JavaScript - Global Variables
- JavaScript - Smart Function Parameters
- JavaScript Objects
- JavaScript - Number
- JavaScript - Boolean
- JavaScript - Strings
- JavaScript - Arrays
- JavaScript - Date
- JavaScript - DataView
- JavaScript - Math
- JavaScript - RegExp
- JavaScript - Symbol
- JavaScript - Sets
- JavaScript - WeakSet
- JavaScript - Maps
- JavaScript - WeakMap
- JavaScript - Iterables
- JavaScript - Reflect
- JavaScript - TypedArray
- JavaScript - Template Literals
- JavaScript - Tagged Templates
- Object Oriented JavaScript
- JavaScript - Objects
- JavaScript - Classes
- JavaScript - Object Properties
- JavaScript - Object Methods
- JavaScript - Static Methods
- JavaScript - Display Objects
- JavaScript - Object Accessors
- JavaScript - Object Constructors
- JavaScript - Native Prototypes
- JavaScript - ES5 Object Methods
- JavaScript - Encapsulation
- JavaScript - Inheritance
- JavaScript - Abstraction
- JavaScript - Polymorphism
- JavaScript - Destructuring Assignment
- JavaScript - Object Destructuring
- JavaScript - Array Destructuring
- JavaScript - Nested Destructuring
- JavaScript - Optional Chaining
- JavaScript - Global Object
- JavaScript - Mixins
- JavaScript - Proxies
- JavaScript Versions
- JavaScript - History
- JavaScript - Versions
- JavaScript - ES5
- JavaScript - ES6
- ECMAScript 2016
- ECMAScript 2017
- ECMAScript 2018
- ECMAScript 2019
- ECMAScript 2020
- ECMAScript 2021
- ECMAScript 2022
- JavaScript Cookies
- JavaScript - Cookies
- JavaScript - Cookie Attributes
- JavaScript - Deleting Cookies
- JavaScript Browser BOM
- JavaScript - Browser Object Model
- JavaScript - Window Object
- JavaScript - Document Object
- JavaScript - Screen Object
- JavaScript - History Object
- JavaScript - Navigator Object
- JavaScript - Location Object
- JavaScript - Console Object
- JavaScript Web APIs
- JavaScript - Web API
- JavaScript - History API
- JavaScript - Storage API
- JavaScript - Forms API
- JavaScript - Worker API
- JavaScript - Fetch API
- JavaScript - Geolocation API
- JavaScript Events
- JavaScript - Events
- JavaScript - DOM Events
- JavaScript - addEventListener()
- JavaScript - Mouse Events
- JavaScript - Keyboard Events
- JavaScript - Form Events
- JavaScript - Window/Document Events
- JavaScript - Event Delegation
- JavaScript - Event Bubbling
- JavaScript - Event Capturing
- JavaScript - Custom Events
- JavaScript Error Handling
- JavaScript - Error Handling
- JavaScript - try...catch
- JavaScript - Debugging
- JavaScript - Custom Errors
- JavaScript - Extending Errors
- JavaScript Important Keywords
- JavaScript - this Keyword
- JavaScript - void Keyword
- JavaScript - new Keyword
- JavaScript - var Keyword
- JavaScript HTML DOM
- JavaScript - HTML DOM
- JavaScript - DOM Methods
- JavaScript - DOM Document
- JavaScript - DOM Elements
- JavaScript - DOM Forms
- JavaScript - Changing HTML
- JavaScript - Changing CSS
- JavaScript - DOM Animation
- JavaScript - DOM Navigation
- JavaScript - DOM Collections
- JavaScript - DOM Node Lists
- JavaScript Miscellaneous
- JavaScript - Ajax
- JavaScript - Async Iteration
- JavaScript - Atomics Objects
- JavaScript - Rest Parameter
- JavaScript - Page Redirect
- JavaScript - Dialog Boxes
- JavaScript - Page Printing
- JavaScript - Validations
- JavaScript - Animation
- JavaScript - Multimedia
- JavaScript - Image Map
- JavaScript - Browsers
- JavaScript - JSON
- JavaScript - Multiline Strings
- JavaScript - Date Formats
- JavaScript - Get Date Methods
- JavaScript - Set Date Methods
- JavaScript - Modules
- JavaScript - Dynamic Imports
- JavaScript - BigInt
- JavaScript - Blob
- JavaScript - Unicode
- JavaScript - Shallow Copy
- JavaScript - Call Stack
- JavaScript - Reference Type
- JavaScript - IndexedDB
- JavaScript - Clickjacking Attack
- JavaScript - Currying
- JavaScript - Graphics
- JavaScript - Canvas
- JavaScript - Debouncing
- JavaScript - Performance
- JavaScript - Style Guide
- JavaScript Useful Resources
- JavaScript - Questions And Answers
- JavaScript - Quick Guide
- JavaScript - Functions
- JavaScript - Resources
JavaScript - Function Invocation
Function Invocation
The function invocation in JavaScript is the process of executing a function. A JavaScript function can be invoked using the function name followed by a pair of parentheses. When you write a function code in JavaScript, it defines the function with expressions and statements. Now, to evaluate these expressions, it is necessary to invoke the function. The function invocation is the synonym of the function call or function execution.
A JavaScript function can be defined using function declaration or function expression. The code inside the curly braces in the function definition are not executed when function is defined. To execute the code, we need to invoke the function.
The call a function and invoke a function are two interchangeable terms are commonly used. But we can invoke a function without calling it. For example, self-invoking functions are invoked without calling them.
Syntax
The syntax of function invocation in JavaScript is as follows –
functionName() OR functionName(arg1, arg2, ... argN);
Here 'functionName' is the function to be invoked. We can pass as many arguments as the number of parameters listed in function definition.
Example
In the example below, we have defined the merge() function taking two parameters. After that, we used the function name to invoke the function by passing the arguments.
<html> <body> <p id = "output"> </p> <script> function merge(str1, str2) { return str1 + str2; } document.getElementById("output").innerHTML = merge("Hello", " World!"); </script> </body> </html>
Output
Hello World!
Invocation of Function Constructor
When you invoke a function with the 'new' keyword, it works as a function constructor. The function constructor is used to create an object from the function definition.
Syntax
Following is the syntax to invoke the function as a constructor.
const varName = new funcName(arguments);
In the above syntax, we invoked the function with a 'new' keyword and passed the arguments.
Example
In the example below, we use the function as an object template. Here, the 'this' keyword represents the function object, and we use it to initialize the variables.
After that, we invoke the function car with the 'new' keyword to create an object using the function template.
<html> <body> <p id = "output"> The ODCar object is: </p> <script> function Car(name, model, year) { this.name = name; this.model = model; this.year = year; } const ODCar = new Car("OD", "Q6", 2020); document.getElementById("output").innerHTML += JSON.stringify(ODCar); </script> </body> </html>
Output
The ODCar object is: {"name":"OD","model":"Q6","year":2020}
Object Method Invocation
We haven't covered JavaScript objects yet in this tutorial but we will cover it in upcoming chapters. Here, let's learn the object method invocation in short.
The JavaScript object can also contain the function, and it is called the method.
Syntax
Following is the syntax below to invoke the JavaScript object method.
obj.func();
In the above syntax, the 'obj' is an object containing the method, and 'func' is a method name to execute.
Example
In the example below, we have defined the 'obj' object containing the 'name' property and the 'getAge()' method.
Outside the object, we access the method by the object reference and invoke the method. In the output, the method prints 10.
<html> <body> <p id = "output">The age of John is: </p> <script> const obj = { name: "John", getAge: () => { return 10; } } document.getElementById("output").innerHTML += obj.getAge(); </script> </body> </html>
Output
The age of John is: 10
Self-Invoking Functions
The self-invoking functions in JavaScript are executed just after they are defined. There is no need to call these types of function to invoke them. The self-invoking functions are always defined using anonymous function expression. These types of functions are also called immediately invoked function expressions (IIFEs). To invoke these function, we wrap the function expression within a grouping operator (parentheses) and then add a pair of parentheses.
Example
Try the following example. In this example, we define a function to show a "Hello world" message in alert box.
<html> <head> <script> (function () {alert("Hello World")})(); </script> </head> <body> </body> </html>
Other methods to invoke the function
JavaScript contains two special methods to invoke the function differently. Here, we have explained each method in the table below.
Method | Function invocation | Arguments |
---|---|---|
Call() | Immediate invocation | Separate arguments |
Apply() | Immediate invocation | Array of arguments |
The difference between the call() and apply() method is how it takes the function arguments. We will learn each of the above methods with examples in the next chapters one by one.