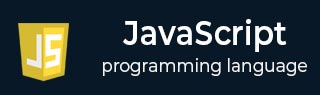
- Javascript Basics Tutorial
- Javascript - Home
- JavaScript - Overview
- JavaScript - Features
- JavaScript - Enabling
- JavaScript - Placement
- JavaScript - Syntax
- JavaScript - Hello World
- JavaScript - Console.log()
- JavaScript - Comments
- JavaScript - Variables
- JavaScript - let Statement
- JavaScript - Constants
- JavaScript - Data Types
- JavaScript - Type Conversions
- JavaScript - Strict Mode
- JavaScript - Reserved Keywords
- JavaScript Operators
- JavaScript - Operators
- JavaScript - Arithmetic Operators
- JavaScript - Comparison Operators
- JavaScript - Logical Operators
- JavaScript - Bitwise Operators
- JavaScript - Assignment Operators
- JavaScript - Conditional Operators
- JavaScript - typeof Operator
- JavaScript - Nullish Coalescing Operator
- JavaScript - Delete Operator
- JavaScript - Comma Operator
- JavaScript - Grouping Operator
- JavaScript - Yield Operator
- JavaScript - Spread Operator
- JavaScript - Exponentiation Operator
- JavaScript - Operator Precedence
- JavaScript Control Flow
- JavaScript - If...Else
- JavaScript - While Loop
- JavaScript - For Loop
- JavaScript - For...in
- Javascript - For...of
- JavaScript - Loop Control
- JavaScript - Break Statement
- JavaScript - Continue Statement
- JavaScript - Switch Case
- JavaScript - User Defined Iterators
- JavaScript Functions
- JavaScript - Functions
- JavaScript - Function Expressions
- JavaScript - Function Parameters
- JavaScript - Default Parameters
- JavaScript - Function() Constructor
- JavaScript - Function Hoisting
- JavaScript - Self-Invoking Functions
- JavaScript - Arrow Functions
- JavaScript - Function Invocation
- JavaScript - Function call()
- JavaScript - Function apply()
- JavaScript - Function bind()
- JavaScript - Closures
- JavaScript - Variable Scope
- JavaScript - Global Variables
- JavaScript - Smart Function Parameters
- JavaScript Objects
- JavaScript - Number
- JavaScript - Boolean
- JavaScript - Strings
- JavaScript - Arrays
- JavaScript - Date
- JavaScript - DataView
- JavaScript - Math
- JavaScript - RegExp
- JavaScript - Symbol
- JavaScript - Sets
- JavaScript - WeakSet
- JavaScript - Maps
- JavaScript - WeakMap
- JavaScript - Iterables
- JavaScript - Reflect
- JavaScript - TypedArray
- JavaScript - Template Literals
- JavaScript - Tagged Templates
- Object Oriented JavaScript
- JavaScript - Objects
- JavaScript - Classes
- JavaScript - Object Properties
- JavaScript - Object Methods
- JavaScript - Static Methods
- JavaScript - Display Objects
- JavaScript - Object Accessors
- JavaScript - Object Constructors
- JavaScript - Native Prototypes
- JavaScript - ES5 Object Methods
- JavaScript - Encapsulation
- JavaScript - Inheritance
- JavaScript - Abstraction
- JavaScript - Polymorphism
- JavaScript - Destructuring Assignment
- JavaScript - Object Destructuring
- JavaScript - Array Destructuring
- JavaScript - Nested Destructuring
- JavaScript - Optional Chaining
- JavaScript - Global Object
- JavaScript - Mixins
- JavaScript - Proxies
- JavaScript Versions
- JavaScript - History
- JavaScript - Versions
- JavaScript - ES5
- JavaScript - ES6
- ECMAScript 2016
- ECMAScript 2017
- ECMAScript 2018
- ECMAScript 2019
- ECMAScript 2020
- ECMAScript 2021
- ECMAScript 2022
- JavaScript Cookies
- JavaScript - Cookies
- JavaScript - Cookie Attributes
- JavaScript - Deleting Cookies
- JavaScript Browser BOM
- JavaScript - Browser Object Model
- JavaScript - Window Object
- JavaScript - Document Object
- JavaScript - Screen Object
- JavaScript - History Object
- JavaScript - Navigator Object
- JavaScript - Location Object
- JavaScript - Console Object
- JavaScript Web APIs
- JavaScript - Web API
- JavaScript - History API
- JavaScript - Storage API
- JavaScript - Forms API
- JavaScript - Worker API
- JavaScript - Fetch API
- JavaScript - Geolocation API
- JavaScript Events
- JavaScript - Events
- JavaScript - DOM Events
- JavaScript - addEventListener()
- JavaScript - Mouse Events
- JavaScript - Keyboard Events
- JavaScript - Form Events
- JavaScript - Window/Document Events
- JavaScript - Event Delegation
- JavaScript - Event Bubbling
- JavaScript - Event Capturing
- JavaScript - Custom Events
- JavaScript Error Handling
- JavaScript - Error Handling
- JavaScript - try...catch
- JavaScript - Debugging
- JavaScript - Custom Errors
- JavaScript - Extending Errors
- JavaScript Important Keywords
- JavaScript - this Keyword
- JavaScript - void Keyword
- JavaScript - new Keyword
- JavaScript - var Keyword
- JavaScript HTML DOM
- JavaScript - HTML DOM
- JavaScript - DOM Methods
- JavaScript - DOM Document
- JavaScript - DOM Elements
- JavaScript - DOM Forms
- JavaScript - Changing HTML
- JavaScript - Changing CSS
- JavaScript - DOM Animation
- JavaScript - DOM Navigation
- JavaScript - DOM Collections
- JavaScript - DOM Node Lists
- JavaScript Miscellaneous
- JavaScript - Ajax
- JavaScript - Async Iteration
- JavaScript - Atomics Objects
- JavaScript - Rest Parameter
- JavaScript - Page Redirect
- JavaScript - Dialog Boxes
- JavaScript - Page Printing
- JavaScript - Validations
- JavaScript - Animation
- JavaScript - Multimedia
- JavaScript - Image Map
- JavaScript - Browsers
- JavaScript - JSON
- JavaScript - Multiline Strings
- JavaScript - Date Formats
- JavaScript - Get Date Methods
- JavaScript - Set Date Methods
- JavaScript - Modules
- JavaScript - Dynamic Imports
- JavaScript - BigInt
- JavaScript - Blob
- JavaScript - Unicode
- JavaScript - Shallow Copy
- JavaScript - Call Stack
- JavaScript - Reference Type
- JavaScript - IndexedDB
- JavaScript - Clickjacking Attack
- JavaScript - Currying
- JavaScript - Graphics
- JavaScript - Canvas
- JavaScript - Debouncing
- JavaScript - Performance
- JavaScript - Style Guide
- JavaScript Useful Resources
- JavaScript - Questions And Answers
- JavaScript - Quick Guide
- JavaScript - Functions
- JavaScript - Resources
JavaScript - For Loop
The JavaScript for loop is used to execute a block of code repeteatedly, until a specified condition evaluates to false. It can be used for iteration if the number of iteration is fixed and known.
The JavaScript loops are used to execute the particular block of code repeatedly. The 'for' loop is the most compact form of looping. It includes the following three important parts −
Initialization − The loop initialization expression is where we initialize our counter to a starting value. The initialization statement is executed before the loop begins.
Condition − The condition expression which will test if a given condition is true or not. If the condition is true, then the code given inside the loop will be executed. Otherwise, the control will come out of the loop.
Iteration − The iteration expression is where you can increase or decrease your counter.
You can put all the three parts in a single line separated by semicolons.
Flow Chart
The flow chart of a for loop in JavaScript would be as follows −

Syntax
The syntax of for loop is JavaScript is as follows −
for (initialization; condition; iteration) { Statement(s) to be executed if condition is true }
Above all 3 statements are optional.
Examples
Try the following examples to learn how a for loop works in JavaScript.
Example: Executing a code block repeatedly
In the example below, we used the for loop to print the output's updated value of the 'count' variable. In each iteration of the loop, we increment the value of 'count' by 1 and print in the output.
<html> <head> <title> JavaScript - for loop </title> </head> <body> <p id = "output"> </p> <script> const output = document.getElementById("output"); output.innerHTML = "Starting Loop <br>"; let count; for (let count = 0; count < 10; count++) { output.innerHTML += "Current Count : " + count + "<br/>"; } output.innerHTML += "Loop stopped!"; </script> </body> </html>
Output
Starting Loop Current Count : 0 Current Count : 1 Current Count : 2 Current Count : 3 Current Count : 4 Current Count : 5 Current Count : 6 Current Count : 7 Current Count : 8 Current Count : 9 Loop stopped!
Example: Initialization is optional
The below code demonstrates that the first statement is optional in the for loop. You can also initialize the variable outside the loop and use it with the loop.
Whenever you need to use the looping variable, even after the execution of the loop is completed, you can initialize a variable in the parent scope of the loop, as we have done in the below code. We also print the value of p outside the loop.
<html> <head> <title> Initialization is optional in for loop </title> </head> <body> <p id = "output"> </p> <script> let output = document.getElementById("output"); var p = 0; for (; p < 5; p++) { output.innerHTML += "P -> " + p + "<br/>"; } output.innerHTML += "Outside the loop! <br>"; output.innerHTML += "P -> " + p + "<br/>"; </script> </body> </html>Output
P -> 0 P -> 1 P -> 2 P -> 3 P -> 4 Outside the loop! P -> 5
Example: Conditional statement is optional
The below code demonstrates that the conditional statement in the for loop is optional. However, if you don't write any condition, it will make infinite iterations. So, you can use the 'break' keyword with the for loop to stop the execution of the loop, as we have done in the below code.
<html> <head> <title> Conditional statement is optional in for loop </title> </head> <body> <p id = "output"> </p> <script> let output = document.getElementById("output"); let arr = [10, 3, 76, 23, 890, 123, 54] var p = 0; for (; ; p++) { if (p >= arr.length) { break; } output.innerHTML += "arr[" + p + "] -> " + arr[p] + "<br/>"; } </script> </body> </html>Output
arr[0] -> 10 arr[1] -> 3 arr[2] -> 76 arr[3] -> 23 arr[4] -> 890 arr[5] -> 123 arr[6] -> 54
Example: Iteration statement is optional
In the for loop, the third statement is also optional and is used to increment the iterative variable. The alternative solution is that we can update the iterative variable inside the loop body.
<html> <head> <title> Iteration statement is optional </title> </head> <body> <p id = "output"> </p> <script> let output = document.getElementById("output"); let str = "Tutorialspoint"; var p = 0; for (; ;) { if (p >= str.length) { break; } output.innerHTML += "str[" + p + "] -> " + str[p] + "<br/>"; p++; } </script> </body> </html>Output
str[0] -> T str[1] -> u str[2] -> t str[3] -> o str[4] -> r str[5] -> i str[6] -> a str[7] -> l str[8] -> s str[9] -> p str[10] -> o str[11] -> i str[12] -> n str[13] -> t