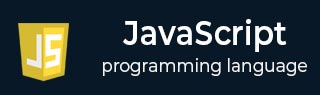
- Javascript Basics Tutorial
- Javascript - Home
- JavaScript - Overview
- JavaScript - Features
- JavaScript - Enabling
- JavaScript - Placement
- JavaScript - Syntax
- JavaScript - Hello World
- JavaScript - Console.log()
- JavaScript - Comments
- JavaScript - Variables
- JavaScript - let Statement
- JavaScript - Constants
- JavaScript - Data Types
- JavaScript - Type Conversions
- JavaScript - Strict Mode
- JavaScript - Reserved Keywords
- JavaScript Operators
- JavaScript - Operators
- JavaScript - Arithmetic Operators
- JavaScript - Comparison Operators
- JavaScript - Logical Operators
- JavaScript - Bitwise Operators
- JavaScript - Assignment Operators
- JavaScript - Conditional Operators
- JavaScript - typeof Operator
- JavaScript - Nullish Coalescing Operator
- JavaScript - Delete Operator
- JavaScript - Comma Operator
- JavaScript - Grouping Operator
- JavaScript - Yield Operator
- JavaScript - Spread Operator
- JavaScript - Exponentiation Operator
- JavaScript - Operator Precedence
- JavaScript Control Flow
- JavaScript - If...Else
- JavaScript - While Loop
- JavaScript - For Loop
- JavaScript - For...in
- Javascript - For...of
- JavaScript - Loop Control
- JavaScript - Break Statement
- JavaScript - Continue Statement
- JavaScript - Switch Case
- JavaScript - User Defined Iterators
- JavaScript Functions
- JavaScript - Functions
- JavaScript - Function Expressions
- JavaScript - Function Parameters
- JavaScript - Default Parameters
- JavaScript - Function() Constructor
- JavaScript - Function Hoisting
- JavaScript - Self-Invoking Functions
- JavaScript - Arrow Functions
- JavaScript - Function Invocation
- JavaScript - Function call()
- JavaScript - Function apply()
- JavaScript - Function bind()
- JavaScript - Closures
- JavaScript - Variable Scope
- JavaScript - Global Variables
- JavaScript - Smart Function Parameters
- JavaScript Objects
- JavaScript - Number
- JavaScript - Boolean
- JavaScript - Strings
- JavaScript - Arrays
- JavaScript - Date
- JavaScript - DataView
- JavaScript - Math
- JavaScript - RegExp
- JavaScript - Symbol
- JavaScript - Sets
- JavaScript - WeakSet
- JavaScript - Maps
- JavaScript - WeakMap
- JavaScript - Iterables
- JavaScript - Reflect
- JavaScript - TypedArray
- JavaScript - Template Literals
- JavaScript - Tagged Templates
- Object Oriented JavaScript
- JavaScript - Objects
- JavaScript - Classes
- JavaScript - Object Properties
- JavaScript - Object Methods
- JavaScript - Static Methods
- JavaScript - Display Objects
- JavaScript - Object Accessors
- JavaScript - Object Constructors
- JavaScript - Native Prototypes
- JavaScript - ES5 Object Methods
- JavaScript - Encapsulation
- JavaScript - Inheritance
- JavaScript - Abstraction
- JavaScript - Polymorphism
- JavaScript - Destructuring Assignment
- JavaScript - Object Destructuring
- JavaScript - Array Destructuring
- JavaScript - Nested Destructuring
- JavaScript - Optional Chaining
- JavaScript - Global Object
- JavaScript - Mixins
- JavaScript - Proxies
- JavaScript Versions
- JavaScript - History
- JavaScript - Versions
- JavaScript - ES5
- JavaScript - ES6
- ECMAScript 2016
- ECMAScript 2017
- ECMAScript 2018
- ECMAScript 2019
- ECMAScript 2020
- ECMAScript 2021
- ECMAScript 2022
- JavaScript Cookies
- JavaScript - Cookies
- JavaScript - Cookie Attributes
- JavaScript - Deleting Cookies
- JavaScript Browser BOM
- JavaScript - Browser Object Model
- JavaScript - Window Object
- JavaScript - Document Object
- JavaScript - Screen Object
- JavaScript - History Object
- JavaScript - Navigator Object
- JavaScript - Location Object
- JavaScript - Console Object
- JavaScript Web APIs
- JavaScript - Web API
- JavaScript - History API
- JavaScript - Storage API
- JavaScript - Forms API
- JavaScript - Worker API
- JavaScript - Fetch API
- JavaScript - Geolocation API
- JavaScript Events
- JavaScript - Events
- JavaScript - DOM Events
- JavaScript - addEventListener()
- JavaScript - Mouse Events
- JavaScript - Keyboard Events
- JavaScript - Form Events
- JavaScript - Window/Document Events
- JavaScript - Event Delegation
- JavaScript - Event Bubbling
- JavaScript - Event Capturing
- JavaScript - Custom Events
- JavaScript Error Handling
- JavaScript - Error Handling
- JavaScript - try...catch
- JavaScript - Debugging
- JavaScript - Custom Errors
- JavaScript - Extending Errors
- JavaScript Important Keywords
- JavaScript - this Keyword
- JavaScript - void Keyword
- JavaScript - new Keyword
- JavaScript - var Keyword
- JavaScript HTML DOM
- JavaScript - HTML DOM
- JavaScript - DOM Methods
- JavaScript - DOM Document
- JavaScript - DOM Elements
- JavaScript - DOM Forms
- JavaScript - Changing HTML
- JavaScript - Changing CSS
- JavaScript - DOM Animation
- JavaScript - DOM Navigation
- JavaScript - DOM Collections
- JavaScript - DOM Node Lists
- JavaScript Miscellaneous
- JavaScript - Ajax
- JavaScript - Async Iteration
- JavaScript - Atomics Objects
- JavaScript - Rest Parameter
- JavaScript - Page Redirect
- JavaScript - Dialog Boxes
- JavaScript - Page Printing
- JavaScript - Validations
- JavaScript - Animation
- JavaScript - Multimedia
- JavaScript - Image Map
- JavaScript - Browsers
- JavaScript - JSON
- JavaScript - Multiline Strings
- JavaScript - Date Formats
- JavaScript - Get Date Methods
- JavaScript - Set Date Methods
- JavaScript - Modules
- JavaScript - Dynamic Imports
- JavaScript - BigInt
- JavaScript - Blob
- JavaScript - Unicode
- JavaScript - Shallow Copy
- JavaScript - Call Stack
- JavaScript - Reference Type
- JavaScript - IndexedDB
- JavaScript - Clickjacking Attack
- JavaScript - Currying
- JavaScript - Graphics
- JavaScript - Canvas
- JavaScript - Debouncing
- JavaScript - Performance
- JavaScript - Style Guide
- JavaScript Useful Resources
- JavaScript - Questions And Answers
- JavaScript - Quick Guide
- JavaScript - Functions
- JavaScript - Resources
JavaScript - Extending Errors
The custom errors in JavaScript are errors that you create yourself, as opposed to the built-in errors that JavaScript throws. You can create custom errors to handle specific types of errors that may occur in your code.
To create a custom error, you can use the Error constructor. The Error constructor takes a string as its argument, which will be the message of the error.
Extending the Error Class: Creating Custom Errors
The best way to create custom errors is by creating a new class and extending it using the 'extends' keyword. It uses the concept of inheritance, and the custom error class inherits the properties of the Error class.
In the constructor() function, you can initialize the properties of the custom error class.
Syntax
You can follow the syntax below to create custom errors by extending the Error class.
class customError extends Error { constructor(message) { super(message) // Initialize properties } }
In the above code, we call the parent class constructor using the super() method.
You can also initialize the properties of the customError class in the constructor function.
Example
In the code below, we take the input from the user. When a user clicks the check age button, it calls the checkAge() function.
We have defined the ageError class in JavaScript code and extended it with the Error class. In the ageError class, we have added the constructor() function to initialize the properties.
In the constructor() function, we used the super() method to initialize the message property of the Error class. Also, we have initialized the 'name' and 'age' properties in the constructor function.
In the checkAge() function, we throw the error if the age is less than 18, and in the catch{} block, we print the error message and age.
<html> <body> <p>Enter your age: <input type = "number" id = "age" /> </p> <button onclick = "checkAge()"> Check Age </button> <p id = "demo"> </p> <script> const output = document.getElementById("demo"); class ageError extends Error { constructor(message, age) { super(message); this.name = "ageError"; this.age = age // Custom property } } function checkAge() { const ageValue = document.getElementById('age').value; try { if (ageValue < 18) { // Throw error when age is less than 18 throw new ageError("You are too young", ageValue); } else { output.innerHTML = "You are old enough"; } } catch (e) { output.innerHTML = "Error: " + e.message + ". <br>"; output.innerHTML += "Age: " + e.age + "<br>"; } } </script> </body> </html>
Output
Enter your age: 5 Check Age Error: You are too young. Age: 5
If you want to create multiple new classes for the custom errors only to provide the clarified error type and message and don't want to change the properties of the Error class, you can use the syntax below.
class InputError extends Error {};
Let's learn it via the example below.
Example
In the code below, we have created 3 different custom classes and extended them with the Error class to create custom errors.
In the try{} block, we throw the StringError.
In the catch{} block, we used the instanceOf operator to check the type of the error object and print the error message accordingly.
<html> <body> <div id = "demo"> </div> <script> const output = document.getElementById("demo"); class StringError extends Error { }; class NumberError extends Error { }; class BooleanError extends Error { }; try { throw new StringError("This is a string error"); } catch (e) { if (e instanceof StringError) { output.innerHTML = "String Error"; } else if (e instanceof NumberError) { output.innerHTML = "Number Error"; } else if (e instanceof BooleanError) { output.innerHTML = "Boolean Error"; } else { output.innerHTML = "Unknown Error"; } } </script> </body> </html>
Output
String Error
Multilevel Inheritance
You can create a general custom error by extending it with the Error class. After that, you can extend the custom error class to create a more generalized error class.
Let's understand it via the example below.
Example
In the code below, we have defined the 'NotFound' class and extended it using the Error class.
After that, we defined the 'propertyNotFound' and 'valueNotFound' classes and extended them with the 'NotFound' class. Here, we have done the multilevel inheritance.
In the try block, we throw a valueNotFound error if the array doesn't contain 6.
In the catch block, we print the error.
<html> <body> <div id = "output"> </div> <script> const output = document.getElementById("output"); class NotFound extends Error { constructor(message) { super(message); this.name = "NotFound"; } } // Further Inheritance class propertyNotFound extends NotFound { constructor(message) { super(message); this.name = "propertyNotFound"; } } // Further Inheritance class ElementNotFound extends NotFound { constructor(message) { super(message); this.name = "ElementNotFound"; } } try { let arr = [1, 2, 3, 4, 5]; // Throw an error if array doesn't contain 6 if (!arr.includes(6)) { throw new propertyNotFound("Array doesn't contain 6"); } } catch (e) { output.innerHTML = e.name + ": " + e.message; } </script> </body> </html>
Output
propertyNotFound: Array doesn't contain 6
You can also throw the propertyNotFound error if any object doesn't contain the particular property.