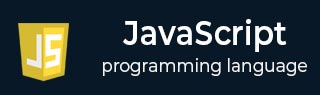
- Javascript Basics Tutorial
- Javascript - Home
- JavaScript - Overview
- JavaScript - Features
- JavaScript - Enabling
- JavaScript - Placement
- JavaScript - Syntax
- JavaScript - Hello World
- JavaScript - Console.log()
- JavaScript - Comments
- JavaScript - Variables
- JavaScript - let Statement
- JavaScript - Constants
- JavaScript - Data Types
- JavaScript - Type Conversions
- JavaScript - Strict Mode
- JavaScript - Reserved Keywords
- JavaScript Operators
- JavaScript - Operators
- JavaScript - Arithmetic Operators
- JavaScript - Comparison Operators
- JavaScript - Logical Operators
- JavaScript - Bitwise Operators
- JavaScript - Assignment Operators
- JavaScript - Conditional Operators
- JavaScript - typeof Operator
- JavaScript - Nullish Coalescing Operator
- JavaScript - Delete Operator
- JavaScript - Comma Operator
- JavaScript - Grouping Operator
- JavaScript - Yield Operator
- JavaScript - Spread Operator
- JavaScript - Exponentiation Operator
- JavaScript - Operator Precedence
- JavaScript Control Flow
- JavaScript - If...Else
- JavaScript - While Loop
- JavaScript - For Loop
- JavaScript - For...in
- Javascript - For...of
- JavaScript - Loop Control
- JavaScript - Break Statement
- JavaScript - Continue Statement
- JavaScript - Switch Case
- JavaScript - User Defined Iterators
- JavaScript Functions
- JavaScript - Functions
- JavaScript - Function Expressions
- JavaScript - Function Parameters
- JavaScript - Default Parameters
- JavaScript - Function() Constructor
- JavaScript - Function Hoisting
- JavaScript - Self-Invoking Functions
- JavaScript - Arrow Functions
- JavaScript - Function Invocation
- JavaScript - Function call()
- JavaScript - Function apply()
- JavaScript - Function bind()
- JavaScript - Closures
- JavaScript - Variable Scope
- JavaScript - Global Variables
- JavaScript - Smart Function Parameters
- JavaScript Objects
- JavaScript - Number
- JavaScript - Boolean
- JavaScript - Strings
- JavaScript - Arrays
- JavaScript - Date
- JavaScript - DataView
- JavaScript - Math
- JavaScript - RegExp
- JavaScript - Symbol
- JavaScript - Sets
- JavaScript - WeakSet
- JavaScript - Maps
- JavaScript - WeakMap
- JavaScript - Iterables
- JavaScript - Reflect
- JavaScript - TypedArray
- JavaScript - Template Literals
- JavaScript - Tagged Templates
- Object Oriented JavaScript
- JavaScript - Objects
- JavaScript - Classes
- JavaScript - Object Properties
- JavaScript - Object Methods
- JavaScript - Static Methods
- JavaScript - Display Objects
- JavaScript - Object Accessors
- JavaScript - Object Constructors
- JavaScript - Native Prototypes
- JavaScript - ES5 Object Methods
- JavaScript - Encapsulation
- JavaScript - Inheritance
- JavaScript - Abstraction
- JavaScript - Polymorphism
- JavaScript - Destructuring Assignment
- JavaScript - Object Destructuring
- JavaScript - Array Destructuring
- JavaScript - Nested Destructuring
- JavaScript - Optional Chaining
- JavaScript - Global Object
- JavaScript - Mixins
- JavaScript - Proxies
- JavaScript Versions
- JavaScript - History
- JavaScript - Versions
- JavaScript - ES5
- JavaScript - ES6
- ECMAScript 2016
- ECMAScript 2017
- ECMAScript 2018
- ECMAScript 2019
- ECMAScript 2020
- ECMAScript 2021
- ECMAScript 2022
- JavaScript Cookies
- JavaScript - Cookies
- JavaScript - Cookie Attributes
- JavaScript - Deleting Cookies
- JavaScript Browser BOM
- JavaScript - Browser Object Model
- JavaScript - Window Object
- JavaScript - Document Object
- JavaScript - Screen Object
- JavaScript - History Object
- JavaScript - Navigator Object
- JavaScript - Location Object
- JavaScript - Console Object
- JavaScript Web APIs
- JavaScript - Web API
- JavaScript - History API
- JavaScript - Storage API
- JavaScript - Forms API
- JavaScript - Worker API
- JavaScript - Fetch API
- JavaScript - Geolocation API
- JavaScript Events
- JavaScript - Events
- JavaScript - DOM Events
- JavaScript - addEventListener()
- JavaScript - Mouse Events
- JavaScript - Keyboard Events
- JavaScript - Form Events
- JavaScript - Window/Document Events
- JavaScript - Event Delegation
- JavaScript - Event Bubbling
- JavaScript - Event Capturing
- JavaScript - Custom Events
- JavaScript Error Handling
- JavaScript - Error Handling
- JavaScript - try...catch
- JavaScript - Debugging
- JavaScript - Custom Errors
- JavaScript - Extending Errors
- JavaScript Important Keywords
- JavaScript - this Keyword
- JavaScript - void Keyword
- JavaScript - new Keyword
- JavaScript - var Keyword
- JavaScript HTML DOM
- JavaScript - HTML DOM
- JavaScript - DOM Methods
- JavaScript - DOM Document
- JavaScript - DOM Elements
- JavaScript - DOM Forms
- JavaScript - Changing HTML
- JavaScript - Changing CSS
- JavaScript - DOM Animation
- JavaScript - DOM Navigation
- JavaScript - DOM Collections
- JavaScript - DOM Node Lists
- JavaScript Miscellaneous
- JavaScript - Ajax
- JavaScript - Async Iteration
- JavaScript - Atomics Objects
- JavaScript - Rest Parameter
- JavaScript - Page Redirect
- JavaScript - Dialog Boxes
- JavaScript - Page Printing
- JavaScript - Validations
- JavaScript - Animation
- JavaScript - Multimedia
- JavaScript - Image Map
- JavaScript - Browsers
- JavaScript - JSON
- JavaScript - Multiline Strings
- JavaScript - Date Formats
- JavaScript - Get Date Methods
- JavaScript - Set Date Methods
- JavaScript - Modules
- JavaScript - Dynamic Imports
- JavaScript - BigInt
- JavaScript - Blob
- JavaScript - Unicode
- JavaScript - Shallow Copy
- JavaScript - Call Stack
- JavaScript - Reference Type
- JavaScript - IndexedDB
- JavaScript - Clickjacking Attack
- JavaScript - Currying
- JavaScript - Graphics
- JavaScript - Canvas
- JavaScript - Debouncing
- JavaScript - Performance
- JavaScript - Style Guide
- JavaScript Useful Resources
- JavaScript - Questions And Answers
- JavaScript - Quick Guide
- JavaScript - Functions
- JavaScript - Resources
JavaScript - Deleting Cookies
In order to delete cookies with JavaScript, we can set the expires date to a past date. We can also delete a cookie using the max-age attribute. We can delete the cookies explicitly from the browser.
Deleting cookies with JavaScript removes small bits of data that websites store on a user's computer. Cookies are used to track a user's browsing activity and preferences.
It is important to note that deleting cookies can have unintended consequences. For example, if you delete a cookie that is used to authenticate you to a website, you will be logged out of the website. You should only delete cookies if you are sure that you want to do so.
Different Ways to Delete the Cookies
There are three different ways to delete the cookies −
Set the past expiry date to the 'expires' attribute.
Use the 'max-age' attribute.
Delete cookies explicitly from the browser.
Delete Cookies using 'expires' Attribute
When you set the past expiry date as a value of the 'expires' attribute, the browser automatically deletes the cookie.
Syntax
Follow the syntax below to delete a cookie by setting up the past expiry date as a value of the 'expires' attribute.
document.cookie = "data1=test1;expires=Tue, 22 Aug 2023 12:00:00 UTC;";
In the above syntax, we have set the past date as a value of the 'expires' attribute. You may set any past date.
Example
In the below code, you can click on the set cookies button to set cookies. After that, click on the get cookies button to observe the cookies.
Next, click the delete cookies button, and again get cookies to check whether the cookies are deleted.
Here, we delete the data1 cookie only.
<html> <body> <button onclick = "setCookies()"> Set Cookie </button> <button onclick = "deleteCookies()"> Delete Cookie </button> <button onclick="readCookies()"> Read Cookies </button> <p id = "output"> </p> <script> let output = document.getElementById("output"); function setCookies() { document.cookie = "data1=test1;"; document.cookie = "data2=test2;"; } function deleteCookies() { document.cookie = "data1=test1;expires=Tue, 22 Aug 2023 12:00:00 UTC;"; document.cookie = "data2=test2;expires=Mon, 22 Aug 2050 12:00:00 UTC;"; } function readCookies() { const allCookies = document.cookie.split("; "); output.innerHTML = "The cookie is : <br>"; for (const cookie of allCookies) { const [key, value] = cookie.split("="); if (key == "data1" || key == "data2") { output.innerHTML += `${key} : ${decodeURIComponent(value)} <br>`; } } } </script> </body> </html>
Delete Cookies using 'max-age' Attribute
The browser automatically deletes the cookie when you assign 0 or a negative value to the 'maxAge' attribute.
Syntax
Follow the syntax below to use the max-age attribute to delete the cookie.
document.cookie = "user1=sam;max-age=-60;";
In the above syntax, we have set a negative value to the 'max-age' attribute to delete the cookies.
Example
In the below code, we delete the user1 cookie only in the deleteCookies() function.
<html> <body> <button onclick = "setCookies()"> Set Cookie </button> <button onclick = "deleteCookies()"> Delete Cookie </button> <button onclick = "readCookies()"> Read Cookies </button> <p id = "output"> </p> <script> let output = document.getElementById("output"); function setCookies() { document.cookie = "user1=sam;"; document.cookie = "user2=virat;"; } function deleteCookies() { document.cookie = "user1=sam;max-age=-60;"; document.cookie = "user2=virat;max-age=5000;"; } function readCookies() { const allCookies = document.cookie.split("; "); output.innerHTML = "The cookie is : <br>"; for (const cookie of allCookies) { const [key, value] = cookie.split("="); if (key == "user1" || key == "user2") { output.innerHTML += `${key} : ${decodeURIComponent(value)} <br>`; } } } </script> </body> </html>
Delete Cookies Explicitly from the Browser
You can manually delete the cookies from the browser by following the steps below.
Step 1 − In your browser, click on the three verticle dots at the top right corner. After that, hover over the 'history’, and it will open the menu. In the menu, click on the 'history’.
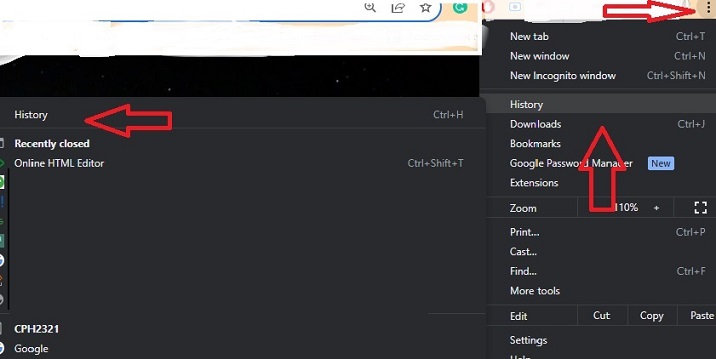
Step 2 − Here, click on the 'clear browsing data' tab.
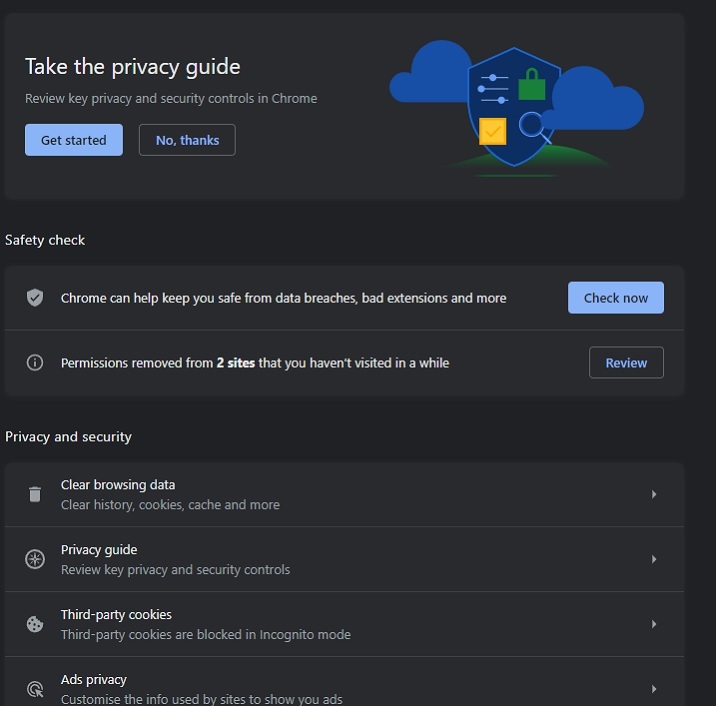
Step 3 − Check the cookies and other site data check boxes here. After that, click on the 'clear data' button.
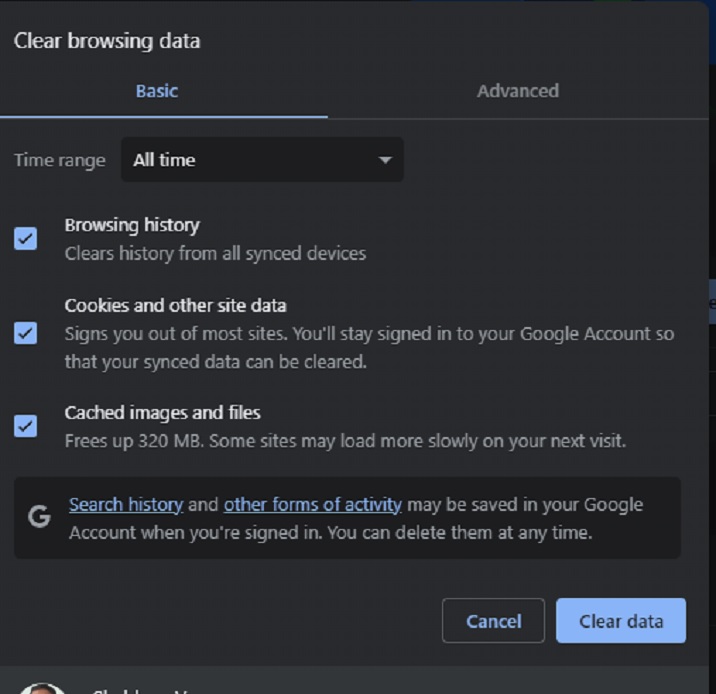
However, the steps might differ based on what browser you are using.
This way, you can use any way among the three to clear your browser's cookie.