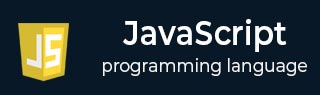
- Javascript Basics Tutorial
- Javascript - Home
- JavaScript - Overview
- JavaScript - Features
- JavaScript - Enabling
- JavaScript - Placement
- JavaScript - Syntax
- JavaScript - Hello World
- JavaScript - Console.log()
- JavaScript - Comments
- JavaScript - Variables
- JavaScript - let Statement
- JavaScript - Constants
- JavaScript - Data Types
- JavaScript - Type Conversions
- JavaScript - Strict Mode
- JavaScript - Reserved Keywords
- JavaScript Operators
- JavaScript - Operators
- JavaScript - Arithmetic Operators
- JavaScript - Comparison Operators
- JavaScript - Logical Operators
- JavaScript - Bitwise Operators
- JavaScript - Assignment Operators
- JavaScript - Conditional Operators
- JavaScript - typeof Operator
- JavaScript - Nullish Coalescing Operator
- JavaScript - Delete Operator
- JavaScript - Comma Operator
- JavaScript - Grouping Operator
- JavaScript - Yield Operator
- JavaScript - Spread Operator
- JavaScript - Exponentiation Operator
- JavaScript - Operator Precedence
- JavaScript Control Flow
- JavaScript - If...Else
- JavaScript - While Loop
- JavaScript - For Loop
- JavaScript - For...in
- Javascript - For...of
- JavaScript - Loop Control
- JavaScript - Break Statement
- JavaScript - Continue Statement
- JavaScript - Switch Case
- JavaScript - User Defined Iterators
- JavaScript Functions
- JavaScript - Functions
- JavaScript - Function Expressions
- JavaScript - Function Parameters
- JavaScript - Default Parameters
- JavaScript - Function() Constructor
- JavaScript - Function Hoisting
- JavaScript - Self-Invoking Functions
- JavaScript - Arrow Functions
- JavaScript - Function Invocation
- JavaScript - Function call()
- JavaScript - Function apply()
- JavaScript - Function bind()
- JavaScript - Closures
- JavaScript - Variable Scope
- JavaScript - Global Variables
- JavaScript - Smart Function Parameters
- JavaScript Objects
- JavaScript - Number
- JavaScript - Boolean
- JavaScript - Strings
- JavaScript - Arrays
- JavaScript - Date
- JavaScript - DataView
- JavaScript - Math
- JavaScript - RegExp
- JavaScript - Symbol
- JavaScript - Sets
- JavaScript - WeakSet
- JavaScript - Maps
- JavaScript - WeakMap
- JavaScript - Iterables
- JavaScript - Reflect
- JavaScript - TypedArray
- JavaScript - Template Literals
- JavaScript - Tagged Templates
- Object Oriented JavaScript
- JavaScript - Objects
- JavaScript - Classes
- JavaScript - Object Properties
- JavaScript - Object Methods
- JavaScript - Static Methods
- JavaScript - Display Objects
- JavaScript - Object Accessors
- JavaScript - Object Constructors
- JavaScript - Native Prototypes
- JavaScript - ES5 Object Methods
- JavaScript - Encapsulation
- JavaScript - Inheritance
- JavaScript - Abstraction
- JavaScript - Polymorphism
- JavaScript - Destructuring Assignment
- JavaScript - Object Destructuring
- JavaScript - Array Destructuring
- JavaScript - Nested Destructuring
- JavaScript - Optional Chaining
- JavaScript - Global Object
- JavaScript - Mixins
- JavaScript - Proxies
- JavaScript Versions
- JavaScript - History
- JavaScript - Versions
- JavaScript - ES5
- JavaScript - ES6
- ECMAScript 2016
- ECMAScript 2017
- ECMAScript 2018
- ECMAScript 2019
- ECMAScript 2020
- ECMAScript 2021
- ECMAScript 2022
- JavaScript Cookies
- JavaScript - Cookies
- JavaScript - Cookie Attributes
- JavaScript - Deleting Cookies
- JavaScript Browser BOM
- JavaScript - Browser Object Model
- JavaScript - Window Object
- JavaScript - Document Object
- JavaScript - Screen Object
- JavaScript - History Object
- JavaScript - Navigator Object
- JavaScript - Location Object
- JavaScript - Console Object
- JavaScript Web APIs
- JavaScript - Web API
- JavaScript - History API
- JavaScript - Storage API
- JavaScript - Forms API
- JavaScript - Worker API
- JavaScript - Fetch API
- JavaScript - Geolocation API
- JavaScript Events
- JavaScript - Events
- JavaScript - DOM Events
- JavaScript - addEventListener()
- JavaScript - Mouse Events
- JavaScript - Keyboard Events
- JavaScript - Form Events
- JavaScript - Window/Document Events
- JavaScript - Event Delegation
- JavaScript - Event Bubbling
- JavaScript - Event Capturing
- JavaScript - Custom Events
- JavaScript Error Handling
- JavaScript - Error Handling
- JavaScript - try...catch
- JavaScript - Debugging
- JavaScript - Custom Errors
- JavaScript - Extending Errors
- JavaScript Important Keywords
- JavaScript - this Keyword
- JavaScript - void Keyword
- JavaScript - new Keyword
- JavaScript - var Keyword
- JavaScript HTML DOM
- JavaScript - HTML DOM
- JavaScript - DOM Methods
- JavaScript - DOM Document
- JavaScript - DOM Elements
- JavaScript - DOM Forms
- JavaScript - Changing HTML
- JavaScript - Changing CSS
- JavaScript - DOM Animation
- JavaScript - DOM Navigation
- JavaScript - DOM Collections
- JavaScript - DOM Node Lists
- JavaScript Miscellaneous
- JavaScript - Ajax
- JavaScript - Async Iteration
- JavaScript - Atomics Objects
- JavaScript - Rest Parameter
- JavaScript - Page Redirect
- JavaScript - Dialog Boxes
- JavaScript - Page Printing
- JavaScript - Validations
- JavaScript - Animation
- JavaScript - Multimedia
- JavaScript - Image Map
- JavaScript - Browsers
- JavaScript - JSON
- JavaScript - Multiline Strings
- JavaScript - Date Formats
- JavaScript - Get Date Methods
- JavaScript - Set Date Methods
- JavaScript - Modules
- JavaScript - Dynamic Imports
- JavaScript - BigInt
- JavaScript - Blob
- JavaScript - Unicode
- JavaScript - Shallow Copy
- JavaScript - Call Stack
- JavaScript - Reference Type
- JavaScript - IndexedDB
- JavaScript - Clickjacking Attack
- JavaScript - Currying
- JavaScript - Graphics
- JavaScript - Canvas
- JavaScript - Debouncing
- JavaScript - Performance
- JavaScript - Style Guide
- JavaScript Useful Resources
- JavaScript - Questions And Answers
- JavaScript - Quick Guide
- JavaScript - Functions
- JavaScript - Resources
JavaScript - Delete Operator
JavaScript Delete Operator
The JavaScript delete operator deletes/ removes a property from an object. It removes the property as well as value of the property from the object. It works only with the objects not with the variables or functions.
In JavaScript, an array is an object, so you can use the 'delete' operator to delete an element from the particular index. However, there are methods like pop(), slice(), or shift() available to delete the element from the array.
Syntax
Follow the below syntax to delete the object property using the 'delete' operator.
delete obj.property; OR delete obj["property"];
Return value − The 'delete' operator returns true if the operand (specified property) is deleted successfully, otherwise returns false if the property is not deleted.
If you try to delete a property that doesn't exist, it will return true but will not affect the object.
Follow the below syntax to delete the array element using the 'delete' operator.
delete arr[index];
Deleting Object Properties
The JavaScript delete operator can be used to delete a property of an object. To delete the property we write the delelte operator followed by the property of the object.
delete obj.propertyName; or delete obj["propertyNmae"];
In the above syntas, object property named propertyName is being deleted from the object called obj.
Example: Deleting an Object Property
The 'obj' object in the example below contains the product, price, and color properties. We used the ‘delete' operator to delete the price property from the object.
<html> <body> <div id="output"></div> <script> const obj = { product: "Mobile", price: 20000, color: "Blue", } delete obj.price; // deleting price document.getElementById("output").innerHTML = "The Mobile price is " + obj.price + " and color is " + obj.color; </script> </body> </html>
It will produce the following result −
The Mobile price is undefined and color is Blue
Notice that when we access the deleted property, it returns undefined.
Example: Deleting a Nonexistent object Property
Try to delete a property that doesn't exit. It will return true but doesn't affect the original object.
<html> <body> <div id="output"></div> <script> const obj = { product: "Mobile", price: 20000 } document.getElementById("output").innerHTML = delete obj.color; </script> </body> </html>
The above program will produce the following result −
true
Deleting Array Elements
We can use the delete operator to remove or delete an element from an array. To delete an element, we use delete keyword followed by array element. We can use square brackets ([]) to access the elements from the array.
Example
The below code contains the array of numbers. We used the 'delete' operator to delete the element from the 1st index of the array. In the output, you can observe that element from the array gets deleted, but the position of the other elements remains as it is. The array length also remains the same.
<html> <body> <div id="output"></div> <script> const arr = [10, 20, 30, 40, 50, 60]; delete arr[1]; // deleting 2nd element from array document.getElementById("output").innerHTML = arr + "<br>" + arr[1]; </script> </body> </html>
It will produce the following result −
10,,30,40,50,60 undefined
Deleting Predefined Object
The JavaScript 'delete' operator can delete the predifiend object such as Math, Date, etc. It is not advisable to delete predefined objects. Once deleted, you can't access the properties of these objects.
Example: Deleting Built-in Math Object
In the example below, we try delete Math object so we get the above error.
<html> <body> <div id="output"></div> <script> var x = 10 var fun = function(){return 20;}; document.getElementById("output").innerHTML = "delete Math.PI :" + delete Math.PI + "<br>" + "delete Math :" + delete Math + "<br>"; try{ document.getElementById("output").innerHTML += Math.PI; } catch(e){ document.getElementById("output").innerHTML += e; } </script> </body> </html>
It will produce the following output −
delete Math.PI :false delete Math :true ReferenceError: Math is not defined
Can't Delete Variables and Functions
The delete operator can't delete the variables or functions.
<html> <body> <div id="output1"></div> <div id="output2"></div> <script> var x = 10 var fun = function(){return 20;}; document.getElementById("output1").innerHTML = delete x; document.getElementById("output2").innerHTML = delete fun; </script> </body> </html>
It will produce the following result −
false false
The variable defined without var, let or const can be deleted. Such a variable is treated as property of window object.
<html> <body> <div id="output1"></div> <div id="output2"></div> <script> try{ x = 10 document.getElementById("output1").innerHTML = delete x; document.getElementById("output2").innerHTML = x; }catch(e){ document.getElementById("output2").innerHTML = e; } </script> </body> </html>
It will produce the following result −
true ReferenceError: x is not defined