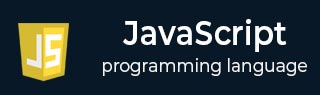
- Javascript Basics Tutorial
- Javascript - Home
- JavaScript - Overview
- JavaScript - Features
- JavaScript - Enabling
- JavaScript - Placement
- JavaScript - Syntax
- JavaScript - Hello World
- JavaScript - Console.log()
- JavaScript - Comments
- JavaScript - Variables
- JavaScript - let Statement
- JavaScript - Constants
- JavaScript - Data Types
- JavaScript - Type Conversions
- JavaScript - Strict Mode
- JavaScript - Reserved Keywords
- JavaScript Operators
- JavaScript - Operators
- JavaScript - Arithmetic Operators
- JavaScript - Comparison Operators
- JavaScript - Logical Operators
- JavaScript - Bitwise Operators
- JavaScript - Assignment Operators
- JavaScript - Conditional Operators
- JavaScript - typeof Operator
- JavaScript - Nullish Coalescing Operator
- JavaScript - Delete Operator
- JavaScript - Comma Operator
- JavaScript - Grouping Operator
- JavaScript - Yield Operator
- JavaScript - Spread Operator
- JavaScript - Exponentiation Operator
- JavaScript - Operator Precedence
- JavaScript Control Flow
- JavaScript - If...Else
- JavaScript - While Loop
- JavaScript - For Loop
- JavaScript - For...in
- Javascript - For...of
- JavaScript - Loop Control
- JavaScript - Break Statement
- JavaScript - Continue Statement
- JavaScript - Switch Case
- JavaScript - User Defined Iterators
- JavaScript Functions
- JavaScript - Functions
- JavaScript - Function Expressions
- JavaScript - Function Parameters
- JavaScript - Default Parameters
- JavaScript - Function() Constructor
- JavaScript - Function Hoisting
- JavaScript - Self-Invoking Functions
- JavaScript - Arrow Functions
- JavaScript - Function Invocation
- JavaScript - Function call()
- JavaScript - Function apply()
- JavaScript - Function bind()
- JavaScript - Closures
- JavaScript - Variable Scope
- JavaScript - Global Variables
- JavaScript - Smart Function Parameters
- JavaScript Objects
- JavaScript - Number
- JavaScript - Boolean
- JavaScript - Strings
- JavaScript - Arrays
- JavaScript - Date
- JavaScript - DataView
- JavaScript - Math
- JavaScript - RegExp
- JavaScript - Symbol
- JavaScript - Sets
- JavaScript - WeakSet
- JavaScript - Maps
- JavaScript - WeakMap
- JavaScript - Iterables
- JavaScript - Reflect
- JavaScript - TypedArray
- JavaScript - Template Literals
- JavaScript - Tagged Templates
- Object Oriented JavaScript
- JavaScript - Objects
- JavaScript - Classes
- JavaScript - Object Properties
- JavaScript - Object Methods
- JavaScript - Static Methods
- JavaScript - Display Objects
- JavaScript - Object Accessors
- JavaScript - Object Constructors
- JavaScript - Native Prototypes
- JavaScript - ES5 Object Methods
- JavaScript - Encapsulation
- JavaScript - Inheritance
- JavaScript - Abstraction
- JavaScript - Polymorphism
- JavaScript - Destructuring Assignment
- JavaScript - Object Destructuring
- JavaScript - Array Destructuring
- JavaScript - Nested Destructuring
- JavaScript - Optional Chaining
- JavaScript - Global Object
- JavaScript - Mixins
- JavaScript - Proxies
- JavaScript Versions
- JavaScript - History
- JavaScript - Versions
- JavaScript - ES5
- JavaScript - ES6
- ECMAScript 2016
- ECMAScript 2017
- ECMAScript 2018
- ECMAScript 2019
- ECMAScript 2020
- ECMAScript 2021
- ECMAScript 2022
- JavaScript Cookies
- JavaScript - Cookies
- JavaScript - Cookie Attributes
- JavaScript - Deleting Cookies
- JavaScript Browser BOM
- JavaScript - Browser Object Model
- JavaScript - Window Object
- JavaScript - Document Object
- JavaScript - Screen Object
- JavaScript - History Object
- JavaScript - Navigator Object
- JavaScript - Location Object
- JavaScript - Console Object
- JavaScript Web APIs
- JavaScript - Web API
- JavaScript - History API
- JavaScript - Storage API
- JavaScript - Forms API
- JavaScript - Worker API
- JavaScript - Fetch API
- JavaScript - Geolocation API
- JavaScript Events
- JavaScript - Events
- JavaScript - DOM Events
- JavaScript - addEventListener()
- JavaScript - Mouse Events
- JavaScript - Keyboard Events
- JavaScript - Form Events
- JavaScript - Window/Document Events
- JavaScript - Event Delegation
- JavaScript - Event Bubbling
- JavaScript - Event Capturing
- JavaScript - Custom Events
- JavaScript Error Handling
- JavaScript - Error Handling
- JavaScript - try...catch
- JavaScript - Debugging
- JavaScript - Custom Errors
- JavaScript - Extending Errors
- JavaScript Important Keywords
- JavaScript - this Keyword
- JavaScript - void Keyword
- JavaScript - new Keyword
- JavaScript - var Keyword
- JavaScript HTML DOM
- JavaScript - HTML DOM
- JavaScript - DOM Methods
- JavaScript - DOM Document
- JavaScript - DOM Elements
- JavaScript - DOM Forms
- JavaScript - Changing HTML
- JavaScript - Changing CSS
- JavaScript - DOM Animation
- JavaScript - DOM Navigation
- JavaScript - DOM Collections
- JavaScript - DOM Node Lists
- JavaScript Miscellaneous
- JavaScript - Ajax
- JavaScript - Async Iteration
- JavaScript - Atomics Objects
- JavaScript - Rest Parameter
- JavaScript - Page Redirect
- JavaScript - Dialog Boxes
- JavaScript - Page Printing
- JavaScript - Validations
- JavaScript - Animation
- JavaScript - Multimedia
- JavaScript - Image Map
- JavaScript - Browsers
- JavaScript - JSON
- JavaScript - Multiline Strings
- JavaScript - Date Formats
- JavaScript - Get Date Methods
- JavaScript - Set Date Methods
- JavaScript - Modules
- JavaScript - Dynamic Imports
- JavaScript - BigInt
- JavaScript - Blob
- JavaScript - Unicode
- JavaScript - Shallow Copy
- JavaScript - Call Stack
- JavaScript - Reference Type
- JavaScript - IndexedDB
- JavaScript - Clickjacking Attack
- JavaScript - Currying
- JavaScript - Graphics
- JavaScript - Canvas
- JavaScript - Debouncing
- JavaScript - Performance
- JavaScript - Style Guide
- JavaScript Useful Resources
- JavaScript - Questions And Answers
- JavaScript - Quick Guide
- JavaScript - Functions
- JavaScript - Resources
JavaScript - Changing HTML
Changing HTML with JavaScript
The HTML DOM allows us to change the HTML elements with JavaScript. You can customize or dynamically update the HTML elements using the various methods and properties. For example, you can change the content of the HTML element, remove or add new HTML elements to the web page, change a particular element's attribute's value, etc.
In JavaScript, we can access the HTML elements using id, attribute, tag name, class name, etc. After accessing the elements, we can change, manipulate them using the properties such as innerHTML, outerHTML, etc. and methods such as replaceWith(), appendChild() etc.
Changing HTML Using innerHTML Property
The innerHTML property of the HTML property is used to replace the HTML content of the element or add new HTML elements as children of the current element.
Syntax
Follow the syntax below to use the innerHTML property to change the HTML.
element.innerHTML = HTML_str;
In the above syntax, 'element' is an HTML element accessed in JavaScript, and HTML_str is an HTML in the string format.
Example
In the example below, we replace the HTML content of the div element using the innerHTML property. You can click the button to replace the HTML content in the output.
<html> <body> <div id = "text"> <p> One </p> <p> Two </p> </div> <button onclick = "changeHTML()"> Change HTML </button> <script> function changeHTML() { let text = document.getElementById('text'); text.innerHTML = `<div> JavaScript </div> <div> HTML </div> <div> CSS </div>`; } </script> </body> </html>
Changing HTML Using outerHTML Property
The outerHTML property of the HTML element replaces the HTML of the element, including the tags.
Syntax
Follow the syntax below to use the outerHTML property.
element.outerHTML = HTML_str;
The HTML_str is an HTML content in the string format.
Example
In the below code, we replace the <div> element with the <img> element when users click the button using the outerHTML property.
<html> <body> <div id = "text"> <p> Paragraph One </p> <p> Paragraph Two </p> </div> <p></p> <button onclick = "changeHTML()"> Change HTML </button> <script> function changeHTML() { let text = document.getElementById('text'); text.outerHTML = `<img src="https://www.tutorialspoint.com/static/images/logo.png?v3" alt="Logo">`; } </script> </body> </html>
Replacing HTML Element Using replaceWith() Method
The replaceWIth() method replaces the particular HTML element with a new element.
Syntax
Follow the syntax below to use the replaceWith() method to change the HTML.
Old_lement.replaceChild(new_ele);
You need to take the existing element as a reference of the replaceChild() method and pass the new element as an argument.
Example
In the below code, we used the createElement() method to create a new <p> element. After that, we added the HTML into that.
Next, we have replaced the div element with the new element in the changeHTML() function.
<html> <body> <div id = "text">Hello World!</div> <button onclick = "changeHTML()"> Change HTML </button> <script> function changeHTML() { const text = document.getElementById('text'); const textNode = document.createElement('p'); textNode.innerHTML = "Welcome to the Tutorialspoint!"; // Using the replaceWith() method text.replaceWith(textNode); } </script> </body> </html>
Changing Value of HTML Element's Attribute
You can access the HTML element and set its value in JavaScript.
Syntax
Follow the syntax below to change the value of the HTML element's attribute.
element.attribute = new_value;
Here, 'attribute' is needed to replace the HTML attribute. The 'new_value' is a new value of the HTML attribute.
Example
In the below code, we change the value of the 'src' attribute of the <img> element. When you click the button, it will change the image.
<html> <body> <img src = "https://www.tutorialspoint.com/static/images/logo.png?v3" width = "300px" id = "logoImg" alt = "logo"> <p></p> <button onclick="changeURL()"> Change URL of Image </button> <script> function changeURL() { document.getElementById('logoImg').src = "https://www.tutorialspoint.com/static/images/simply-easy-learning.png"; } </script> </body> </html>
Adding a New Element to the HTML Element
You can use the appendChild() method to add a new HTML child to the particular HTML element.
Syntax
Follow the syntax below to add a new element using the appendCHild() method.
element.appendChild(new_ele);
You need to use the parent element as a reference of the appendChild() method and pass the new element as an argument.
Example
In the below code, we append the <p> Apple <p> as a child of the <div> element.
<html> <body> <div id = "fruits"> <p> Banana </p> <p> Watermelon </p> </div> <button onclick = "AddFruit()"> Add Apple </button> <script> function AddFruit() { const fruits = document.getElementById("fruits"); const p = document.createElement("p"); p.innerHTML = "Apple"; fruits.appendChild(p); // Using the appendChild() method } </script> </body> </html>
Removing a Child Element from the HTML Element
You can use the removeChild() method to remove the child element from the particular HTML element.
Syntax
Follow the syntax below to use the removeChild() method.
element.removeChild(child_ele)
You need to use the HTML element as a reference of the removeChild() method when you need to remove the child element and pass the child element as an argument.
Example
In the below code, we remove the <p> Apple <p> from the <div> element.
<html> <body> <div id = "fruits"> <p> Banana </p> <p> Watermelon </p> <p> Apple </p> </div> <button onclick = "removeFruit()"> Remove Apple </button> <script> function removeFruit() { const fruits = document.getElementById("fruits"); const apple = fruits.children[2]; fruits.removeChild(apple); } </script> </body> </html>
Using the document.write() Method
The document.write() method replaces the whole content of the web page and writes a new HTML.
Syntax
Follow the syntax below to use the document.write() method.
document.write(HTML);
The document.write() method takes an HTML in the string format as a parameter.
Example
In the below code, we replace the content of the whole web page using the document.write() method.
<html> <body> <div id = "fruits"> <p> Banana </p> <p> WaterMealon </p> <p> Apple </p> </div> <button onclick="replaceContent()"> Replace content </button> <script> function replaceContent() { document.write("Hello World"); } </script> </body> </html>
For more practice, you may try to change the first child element, last child element, other attributes, etc. of the HTML elements.