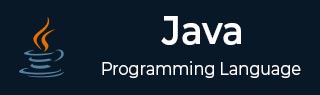
Java Tutorial
- Java - Home
- Java - Overview
- Java - History
- Java - Features
- Java vs C++
- Java Virtual Machine (JVM)
- Java - JDK vs JRE vs JVM
- Java - Hello World Program
- Java - Environment Setup
- Java - Basic Syntax
- Java - Variable Types
- Java - Data Types
- Java - Type Casting
- Java - Unicode System
- Java - Basic Operators
- Java - Comments
Java Control Statements
- Java - Loop Control
- Java - Decision Making
- Java - If-else
- Java - Switch
- Java - For Loops
- Java - For-Each Loops
- Java - While Loops
- Java - do-while Loops
- Java - Break
- Java - Continue
Object Oriented Programming
- Java - OOPs Concepts
- Java - Object & Classes
- Java - Class Attributes
- Java - Class Methods
- Java - Methods
- Java - Variables Scope
- Java - Constructors
- Java - Access Modifiers
- Java - Inheritance
- Java - Aggregation
- Java - Polymorphism
- Java - Overriding
- Java - Method Overloading
- Java - Dynamic Binding
- Java - Static Binding
- Java - Instance Initializer Block
- Java - Abstraction
- Java - Encapsulation
- Java - Interfaces
- Java - Packages
- Java - Inner Classes
- Java - Static Class
- Java - Anonymous Class
- Java - Singleton Class
- Java - Wrapper Classes
- Java - Enums
- Java - Enum Constructor
- Java - Enum Strings
Java Built-in Classes
Java File Handling
- Java - Files
- Java - Create a File
- Java - Write to File
- Java - Read Files
- Java - Delete Files
- Java - Directories
- Java - I/O Streams
Java Error & Exceptions
- Java - Exceptions
- Java - try-catch Block
- Java - try-with-resources
- Java - Multi-catch Block
- Java - Nested try Block
- Java - Finally Block
- Java - throw Exception
- Java - Exception Propagation
- Java - Built-in Exceptions
- Java - Custom Exception
Java Multithreading
- Java - Multithreading
- Java - Thread Life Cycle
- Java - Creating a Thread
- Java - Starting a Thread
- Java - Joining Threads
- Java - Naming Thread
- Java - Thread Scheduler
- Java - Thread Pools
- Java - Main Thread
- Java - Thread Priority
- Java - Daemon Threads
- Java - Thread Group
- Java - Shutdown Hook
Java Synchronization
- Java - Synchronization
- Java - Block Synchronization
- Java - Static Synchronization
- Java - Inter-thread Communication
- Java - Thread Deadlock
- Java - Interrupting a Thread
- Java - Thread Control
- Java - Reentrant Monitor
Java Networking
- Java - Networking
- Java - Socket Programming
- Java - URL Processing
- Java - URL Class
- Java - URLConnection Class
- Java - HttpURLConnection Class
- Java - Socket Class
- Java - Generics
Java Collections
Java Interfaces
- Java - List Interface
- Java - Queue Interface
- Java - Map Interface
- Java - SortedMap Interface
- Java - Set Interface
- Java - SortedSet Interface
Java Data Structures
Java Collections Algorithms
Advanced Java
- Java - Command-Line Arguments
- Java - Lambda Expressions
- Java - Sending Email
- Java - Applet Basics
- Java - Javadoc Comments
- Java - Autoboxing and Unboxing
- Java - File Mismatch Method
- Java - REPL (JShell)
- Java - Multi-Release Jar Files
- Java - Private Interface Methods
- Java - Inner Class Diamond Operator
- Java - Multiresolution Image API
- Java - Collection Factory Methods
- Java - Module System
- Java - Nashorn JavaScript
- Java - Optional Class
- Java - Method References
- Java - Functional Interfaces
- Java - Default Methods
- Java - Base64 Encode Decode
- Java - Switch Expressions
- Java - Teeing Collectors
- Java - Microbenchmark
- Java - Text Blocks
- Java - Dynamic CDS archive
- Java - Z Garbage Collector (ZGC)
- Java - Null Pointer Exception
- Java - Packaging Tools
- Java - Sealed Classes
- Java - Record Classes
- Java - Hidden Classes
- Java - Pattern Matching
- Java - Compact Number Formatting
- Java - Garbage Collection
- Java - JIT Compiler
Java Miscellaneous
- Java - Recursion
- Java - Regular Expressions
- Java - Serialization
- Java - Strings
- Java - Process API Improvements
- Java - Stream API Improvements
- Java - Enhanced @Deprecated Annotation
- Java - CompletableFuture API Improvements
- Java - Streams
- Java - Datetime Api
- Java 8 - New Features
- Java 9 - New Features
- Java 10 - New Features
- Java 11 - New Features
- Java 12 - New Features
- Java 13 - New Features
- Java 14 - New Features
- Java 15 - New Features
- Java 16 - New Features
Java APIs & Frameworks
Java Class References
- Java - Scanner Class
- Java - Arrays Class
- Java - Strings
- Java - Date & Time
- Java - ArrayList
- Java - Vector Class
- Java - Stack Class
- Java - PriorityQueue
- Java - LinkedList
- Java - ArrayDeque
- Java - HashMap
- Java - LinkedHashMap
- Java - WeakHashMap
- Java - EnumMap
- Java - TreeMap
- Java - The IdentityHashMap Class
- Java - HashSet
- Java - EnumSet
- Java - LinkedHashSet
- Java - TreeSet
- Java - BitSet Class
- Java - Dictionary
- Java - Hashtable
- Java - Properties
- Java - Collection Interface
- Java - Array Methods
Java Useful Resources
Java - URL Class
What is a URL?
URL stands for Uniform Resource Locator and represents a resource on the World Wide Web, such as a Webpage or FTP directory.
This section shows you how to write Java programs that communicate with a URL. A URL can be broken down into parts, as follows −
protocol://host:port/path?query#ref
Examples of protocols include HTTP, HTTPS, FTP, and File. The path is also referred to as the filename, and the host is also called the authority.
Example
The following is a URL to a web page whose protocol is HTTP −
https://www.amrood.com/index.htm?language=en#j2se
Notice that this URL does not specify a port, in which case the default port for the protocol is used. With HTTP, the default port is 80.
Java URL Class
The URL class is a part of the java.net package. URL class represents a Uniform Resource Locator (URL). Where, URLs are used for identifying online resources (for example: webpages, images used in the webpages, videos, files, etc.)
The URL class provides several constructors and methods for creating, parsing, and manipulating URLs (or, URL objects).
URL Class Declaration
public final class URL extends Object implements Serializable
URL Class Constructors
The java.net.URL class represents a URL and has a complete set of methods to manipulate URL in Java.
The URL class has several constructors for creating URLs, including the following −
Sr.No. | Constructors & Description |
---|---|
1 | public URL(String protocol, String host, int port, String file) throws MalformedURLException Creates a URL by putting together the given parts. |
2 | public URL(String protocol, String host, int port, String file, URLStreamHandler handler) throws MalformedURLException Creates a URL by putting together the given parts with the specified handler within a specified context. |
3 | public URL(String protocol, String host, String file) throws MalformedURLException Identical to the previous constructor, except that the default port for the given protocol is used. |
4 | public URL(String url) throws MalformedURLException Creates a URL from the given String. |
5 | public URL(URL context, String url) throws MalformedURLException Creates a URL by parsing together the URL and String arguments. |
6 | public URL(URL context, String url, URLStreamHandler handler) throws MalformedURLException Creates a URL by parsing together the URL and String arguments with the specified handler within a specified context. |
URL Class Methods
The URL class contains many methods for accessing the various parts of the URL being represented. Some of the methods in the URL class include the following −
Sr.No. | Method & Description |
---|---|
1 |
This method compares this URL for equality with another object. |
2 |
This method returns the authority of the URL. |
3 |
This method returns the contents of this URL. |
4 | public Object getContent(Class<?>[] classes) This method returns the contents of this URL. |
5 |
This method returns the default port for the protocol of the URL. |
6 |
This method returns the filename of the URL. |
7 |
This method returns the host of the URL. |
8 |
This method returns the path of the URL. |
9 |
This method returns the port of the URL. |
10 |
This method returns the protocol of the URL. |
11 |
This method returns the query part of the URL. |
12 |
This method returns the reference part of the URL. |
13 |
This method returns the userInfo part of the URL. |
14 |
This method creates and return an integer suitable for hash table indexing. |
15 | public URLConnection openConnection() This method returns a URLConnection instance that represents a connection to the remote object referred to by the URL. |
16 | public URLConnection openConnection(Proxy proxy) This method acts as openConnection(), except that the connection will be made through the specified proxy; Protocol handlers that do not support proxing will ignore the proxy parameter and make a normal connection. |
17 | public InputStream openStream() This method opens a connection to this URL and returns an InputStream for reading from that connection. |
18 | public boolean sameFile(URL other) This method compares two URLs, excluding the fragment component. |
19 | public static void setURLStreamHandlerFactory(URLStreamHandlerFactory fac) This method sets an application's URLStreamHandlerFactory. |
20 | public String toExternalForm() This method constructs and return a string representation of this URL. |
21 |
This method constructs and return a string representation of this URL. |
22 |
This method returns a URI equivalent to this URL. |
java.lang.Object
Example of URL Class
The following URLDemo program demonstrates the various parts of a URL. A URL is entered on the command line, and the URLDemo program outputs each part of the given URL.
// File Name : URLDemo.java import java.io.IOException; import java.net.URL; public class URLDemo { public static void main(String [] args) { try { URL url = new URL("https://www.tutorialspoint.com/index.htm?language=en#j2se"); System.out.println("URL is " + url.toString()); System.out.println("protocol is " + url.getProtocol()); System.out.println("authority is " + url.getAuthority()); System.out.println("file name is " + url.getFile()); System.out.println("host is " + url.getHost()); System.out.println("path is " + url.getPath()); System.out.println("port is " + url.getPort()); System.out.println("default port is " + url.getDefaultPort()); System.out.println("query is " + url.getQuery()); System.out.println("ref is " + url.getRef()); } catch (IOException e) { e.printStackTrace(); } } }
A sample run of the this program will produce the following result −
Output
URL is https://www.tutorialspoint.com/index.htm?language=en#j2se protocol is https authority is www.tutorialspoint.com file name is /index.htm?language=en host is www.tutorialspoint.com path is /index.htm port is -1 default port is 443 query is language=en ref is j2se