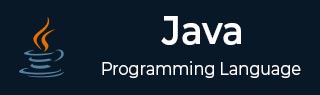
Java Tutorial
- Java - Home
- Java - Overview
- Java - History
- Java - Features
- Java vs C++
- Java Virtual Machine (JVM)
- Java - JDK vs JRE vs JVM
- Java - Hello World Program
- Java - Environment Setup
- Java - Basic Syntax
- Java - Variable Types
- Java - Data Types
- Java - Type Casting
- Java - Unicode System
- Java - Basic Operators
- Java - Comments
Java Control Statements
- Java - Loop Control
- Java - Decision Making
- Java - If-else
- Java - Switch
- Java - For Loops
- Java - For-Each Loops
- Java - While Loops
- Java - do-while Loops
- Java - Break
- Java - Continue
Object Oriented Programming
- Java - OOPs Concepts
- Java - Object & Classes
- Java - Class Attributes
- Java - Class Methods
- Java - Methods
- Java - Variables Scope
- Java - Constructors
- Java - Access Modifiers
- Java - Inheritance
- Java - Aggregation
- Java - Polymorphism
- Java - Overriding
- Java - Method Overloading
- Java - Dynamic Binding
- Java - Static Binding
- Java - Instance Initializer Block
- Java - Abstraction
- Java - Encapsulation
- Java - Interfaces
- Java - Packages
- Java - Inner Classes
- Java - Static Class
- Java - Anonymous Class
- Java - Singleton Class
- Java - Wrapper Classes
- Java - Enums
- Java - Enum Constructor
- Java - Enum Strings
Java Built-in Classes
Java File Handling
- Java - Files
- Java - Create a File
- Java - Write to File
- Java - Read Files
- Java - Delete Files
- Java - Directories
- Java - I/O Streams
Java Error & Exceptions
- Java - Exceptions
- Java - try-catch Block
- Java - try-with-resources
- Java - Multi-catch Block
- Java - Nested try Block
- Java - Finally Block
- Java - throw Exception
- Java - Exception Propagation
- Java - Built-in Exceptions
- Java - Custom Exception
Java Multithreading
- Java - Multithreading
- Java - Thread Life Cycle
- Java - Creating a Thread
- Java - Starting a Thread
- Java - Joining Threads
- Java - Naming Thread
- Java - Thread Scheduler
- Java - Thread Pools
- Java - Main Thread
- Java - Thread Priority
- Java - Daemon Threads
- Java - Thread Group
- Java - Shutdown Hook
Java Synchronization
- Java - Synchronization
- Java - Block Synchronization
- Java - Static Synchronization
- Java - Inter-thread Communication
- Java - Thread Deadlock
- Java - Interrupting a Thread
- Java - Thread Control
- Java - Reentrant Monitor
Java Networking
- Java - Networking
- Java - Socket Programming
- Java - URL Processing
- Java - URL Class
- Java - URLConnection Class
- Java - HttpURLConnection Class
- Java - Socket Class
- Java - Generics
Java Collections
Java Interfaces
- Java - List Interface
- Java - Queue Interface
- Java - Map Interface
- Java - SortedMap Interface
- Java - Set Interface
- Java - SortedSet Interface
Java Data Structures
Java Collections Algorithms
Advanced Java
- Java - Command-Line Arguments
- Java - Lambda Expressions
- Java - Sending Email
- Java - Applet Basics
- Java - Javadoc Comments
- Java - Autoboxing and Unboxing
- Java - File Mismatch Method
- Java - REPL (JShell)
- Java - Multi-Release Jar Files
- Java - Private Interface Methods
- Java - Inner Class Diamond Operator
- Java - Multiresolution Image API
- Java - Collection Factory Methods
- Java - Module System
- Java - Nashorn JavaScript
- Java - Optional Class
- Java - Method References
- Java - Functional Interfaces
- Java - Default Methods
- Java - Base64 Encode Decode
- Java - Switch Expressions
- Java - Teeing Collectors
- Java - Microbenchmark
- Java - Text Blocks
- Java - Dynamic CDS archive
- Java - Z Garbage Collector (ZGC)
- Java - Null Pointer Exception
- Java - Packaging Tools
- Java - Sealed Classes
- Java - Record Classes
- Java - Hidden Classes
- Java - Pattern Matching
- Java - Compact Number Formatting
- Java - Garbage Collection
- Java - JIT Compiler
Java Miscellaneous
- Java - Recursion
- Java - Regular Expressions
- Java - Serialization
- Java - Strings
- Java - Process API Improvements
- Java - Stream API Improvements
- Java - Enhanced @Deprecated Annotation
- Java - CompletableFuture API Improvements
- Java - Streams
- Java - Datetime Api
- Java 8 - New Features
- Java 9 - New Features
- Java 10 - New Features
- Java 11 - New Features
- Java 12 - New Features
- Java 13 - New Features
- Java 14 - New Features
- Java 15 - New Features
- Java 16 - New Features
Java APIs & Frameworks
Java Class References
- Java - Scanner Class
- Java - Arrays Class
- Java - Strings
- Java - Date & Time
- Java - ArrayList
- Java - Vector Class
- Java - Stack Class
- Java - PriorityQueue
- Java - LinkedList
- Java - ArrayDeque
- Java - HashMap
- Java - LinkedHashMap
- Java - WeakHashMap
- Java - EnumMap
- Java - TreeMap
- Java - The IdentityHashMap Class
- Java - HashSet
- Java - EnumSet
- Java - LinkedHashSet
- Java - TreeSet
- Java - BitSet Class
- Java - Dictionary
- Java - Hashtable
- Java - Properties
- Java - Collection Interface
- Java - Array Methods
Java Useful Resources
Java - JVM Shutdown Hook
JVM Shutdown
The following are the two different ways for JVM shutdown –
A controlled process: JVM starts to shut down its process if the System.exit() method is called, CTRL+C is pressed, or if the last non-daemon thread terminates.
An abrupt manner: JVM starts to shut down its process if it receives a kill signal, the Runtime.getRuntime().halt() method is called, or any kind of OS panic.
JVM Shutdown Hook
A shutdown hook is simply an initialized but unstarted thread. When the virtual machine begins its shutdown sequence it will start all registered shutdown hooks in some unspecified order and let them run concurrently. When all the hooks have finished it will then run all uninvoked finalizers if finalization-on-exit has been enabled. Finally, the virtual machine will halt. Note that daemon threads will continue to run during the shutdown sequence, as will non-daemon threads if shutdown was initiated by invoking the exit method.
JVM Shutdown Hook: The addShutdownHook(Thread hook) Method
The Runtime addShutdownHook(Thread hook) method registers a new virtual-machine shutdown hook.
Declaration
Following is the declaration for java.lang.Runtime.addShutdownHook() method
public void addShutdownHook(Thread hook)
Parameters
hook − An initialized but unstarted Thread object.
Return Value
This method does not return a value.
Exception
IllegalArgumentException − If the specified hook has already been registered, or if it can be determined that the hook is already running or has already been run.
IllegalStateException − If the virtual machine is already in the process of shutting down.
SecurityException − If a security manager is present and it denies RuntimePermission("shutdownHooks").
Example of JVM Shutdown Hook
In this example, we're creating a class CustomThread by extending Thread class. This thread object will be used as JVM Shutdown hook. CustomThread class has run() method implementation. In main class TestThread, we've added a shutdown hook using Runtime.getRuntime().addShutdownHook() method, by passing it a thread object. In output you can verify that CustomThread run() method is called when program is about to exit.
package com.tutorialspoint; class CustomThread extends Thread { public void run() { System.out.println("JVM is shutting down."); } } public class TestThread { public static void main(String args[]) throws InterruptedException { try { // register CustomThread as shutdown hook Runtime.getRuntime().addShutdownHook(new CustomThread()); // print the state of the program System.out.println("Program is starting..."); // cause thread to sleep for 3 seconds System.out.println("Waiting for 3 seconds..."); Thread.sleep(3000); // print that the program is closing System.out.println("Program is closing..."); } catch (Exception e) { e.printStackTrace(); } } }
Output
Program is starting... Waiting for 3 seconds... Program is closing... JVM is shutting down.
More Example of JVM Shutdown Hook
Example 1
In this example, we're creating a class CustomThread by implementing Runnable interface. This thread object will be used as JVM Shutdown hook. CustomThread class has run() method implementation. In main class TestThread, we've added a shutdown hook using Runtime.getRuntime().addShutdownHook() method, by passing it a thread object. In output you can verify that CustomThread run() method is called when program is about to exit.
package com.tutorialspoint; class CustomThread implements Runnable { public void run() { System.out.println("JVM is shutting down."); } } public class TestThread { public static void main(String args[]) throws InterruptedException { try { // register CustomThread as shutdown hook Runtime.getRuntime().addShutdownHook(new Thread(new CustomThread())); // print the state of the program System.out.println("Program is starting..."); // cause thread to sleep for 3 seconds System.out.println("Waiting for 3 seconds..."); Thread.sleep(3000); // print that the program is closing System.out.println("Program is closing..."); } catch (Exception e) { e.printStackTrace(); } } }
Output
Program is starting... Waiting for 3 seconds... Program is closing... JVM is shutting down.
Example 2
We can remove the shutdown hook as well using removeShutdownHook(). In this example, we're creating a class CustomThread by implementing Runnable interface. This thread object will be used as JVM Shutdown hook. CustomThread class has run() method implementation. In main class TestThread, we've added a shutdown hook using Runtime.getRuntime().addShutdownHook() method, by passing it a thread object. As last statement, we're removing the hook using Runtime.getRuntime().removeShutdownHook() method
package com.tutorialspoint; class CustomThread implements Runnable { public void run() { System.out.println("JVM is shutting down."); } } public class TestThread { public static void main(String args[]) throws InterruptedException { try { Thread hook = new Thread(new CustomThread()); // register Message as shutdown hook Runtime.getRuntime().addShutdownHook(hook); // print the state of the program System.out.println("Program is starting..."); // cause thread to sleep for 3 seconds System.out.println("Waiting for 3 seconds..."); Thread.sleep(3000); // print that the program is closing System.out.println("Program is closing..."); Runtime.getRuntime().removeShutdownHook(hook); } catch (Exception e) { e.printStackTrace(); } } }
Output
Program is starting... Waiting for 3 seconds... Program is closing...