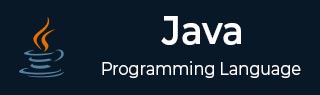
Java Tutorial
- Java - Home
- Java - Overview
- Java - History
- Java - Features
- Java vs C++
- Java Virtual Machine (JVM)
- Java - JDK vs JRE vs JVM
- Java - Hello World Program
- Java - Environment Setup
- Java - Basic Syntax
- Java - Variable Types
- Java - Data Types
- Java - Type Casting
- Java - Unicode System
- Java - Basic Operators
- Java - Comments
Java Control Statements
- Java - Loop Control
- Java - Decision Making
- Java - If-else
- Java - Switch
- Java - For Loops
- Java - For-Each Loops
- Java - While Loops
- Java - do-while Loops
- Java - Break
- Java - Continue
Object Oriented Programming
- Java - OOPs Concepts
- Java - Object & Classes
- Java - Class Attributes
- Java - Class Methods
- Java - Methods
- Java - Variables Scope
- Java - Constructors
- Java - Access Modifiers
- Java - Inheritance
- Java - Aggregation
- Java - Polymorphism
- Java - Overriding
- Java - Method Overloading
- Java - Dynamic Binding
- Java - Static Binding
- Java - Instance Initializer Block
- Java - Abstraction
- Java - Encapsulation
- Java - Interfaces
- Java - Packages
- Java - Inner Classes
- Java - Static Class
- Java - Anonymous Class
- Java - Singleton Class
- Java - Wrapper Classes
- Java - Enums
- Java - Enum Constructor
- Java - Enum Strings
Java Built-in Classes
Java File Handling
- Java - Files
- Java - Create a File
- Java - Write to File
- Java - Read Files
- Java - Delete Files
- Java - Directories
- Java - I/O Streams
Java Error & Exceptions
- Java - Exceptions
- Java - try-catch Block
- Java - try-with-resources
- Java - Multi-catch Block
- Java - Nested try Block
- Java - Finally Block
- Java - throw Exception
- Java - Exception Propagation
- Java - Built-in Exceptions
- Java - Custom Exception
Java Multithreading
- Java - Multithreading
- Java - Thread Life Cycle
- Java - Creating a Thread
- Java - Starting a Thread
- Java - Joining Threads
- Java - Naming Thread
- Java - Thread Scheduler
- Java - Thread Pools
- Java - Main Thread
- Java - Thread Priority
- Java - Daemon Threads
- Java - Thread Group
- Java - Shutdown Hook
Java Synchronization
- Java - Synchronization
- Java - Block Synchronization
- Java - Static Synchronization
- Java - Inter-thread Communication
- Java - Thread Deadlock
- Java - Interrupting a Thread
- Java - Thread Control
- Java - Reentrant Monitor
Java Networking
- Java - Networking
- Java - Socket Programming
- Java - URL Processing
- Java - URL Class
- Java - URLConnection Class
- Java - HttpURLConnection Class
- Java - Socket Class
- Java - Generics
Java Collections
Java Interfaces
- Java - List Interface
- Java - Queue Interface
- Java - Map Interface
- Java - SortedMap Interface
- Java - Set Interface
- Java - SortedSet Interface
Java Data Structures
Java Collections Algorithms
Advanced Java
- Java - Command-Line Arguments
- Java - Lambda Expressions
- Java - Sending Email
- Java - Applet Basics
- Java - Javadoc Comments
- Java - Autoboxing and Unboxing
- Java - File Mismatch Method
- Java - REPL (JShell)
- Java - Multi-Release Jar Files
- Java - Private Interface Methods
- Java - Inner Class Diamond Operator
- Java - Multiresolution Image API
- Java - Collection Factory Methods
- Java - Module System
- Java - Nashorn JavaScript
- Java - Optional Class
- Java - Method References
- Java - Functional Interfaces
- Java - Default Methods
- Java - Base64 Encode Decode
- Java - Switch Expressions
- Java - Teeing Collectors
- Java - Microbenchmark
- Java - Text Blocks
- Java - Dynamic CDS archive
- Java - Z Garbage Collector (ZGC)
- Java - Null Pointer Exception
- Java - Packaging Tools
- Java - Sealed Classes
- Java - Record Classes
- Java - Hidden Classes
- Java - Pattern Matching
- Java - Compact Number Formatting
- Java - Garbage Collection
- Java - JIT Compiler
Java Miscellaneous
- Java - Recursion
- Java - Regular Expressions
- Java - Serialization
- Java - Strings
- Java - Process API Improvements
- Java - Stream API Improvements
- Java - Enhanced @Deprecated Annotation
- Java - CompletableFuture API Improvements
- Java - Streams
- Java - Datetime Api
- Java 8 - New Features
- Java 9 - New Features
- Java 10 - New Features
- Java 11 - New Features
- Java 12 - New Features
- Java 13 - New Features
- Java 14 - New Features
- Java 15 - New Features
- Java 16 - New Features
Java APIs & Frameworks
Java Class References
- Java - Scanner Class
- Java - Arrays Class
- Java - Strings
- Java - Date & Time
- Java - ArrayList
- Java - Vector Class
- Java - Stack Class
- Java - PriorityQueue
- Java - LinkedList
- Java - ArrayDeque
- Java - HashMap
- Java - LinkedHashMap
- Java - WeakHashMap
- Java - EnumMap
- Java - TreeMap
- Java - The IdentityHashMap Class
- Java - HashSet
- Java - EnumSet
- Java - LinkedHashSet
- Java - TreeSet
- Java - BitSet Class
- Java - Dictionary
- Java - Hashtable
- Java - Properties
- Java - Collection Interface
- Java - Array Methods
Java Useful Resources
Java - List Interface
List Interface
The List interface extends Collection and declares the behavior of a collection that stores a sequence of elements.
Elements can be inserted or accessed by their position in the list, using a zero-based index.
A list may contain duplicate elements.
In addition to the methods defined by Collection, List defines some of its own, which are summarized in the following table.
Several of the list methods will throw an UnsupportedOperationException if the collection cannot be modified, and a ClassCastException is generated when one object is incompatible with another.
Declaration of Java List Interface
The following is the declaration of a List interface in Java:
public interface List<E> extends Collection<E>;
Creating a Java list
A Java list is created using the List interface. The syntax is as follows -
List<Obj> list = new ArrayList<Obj> ();
Example of List Interface
The following example demonstrates an example of List interface in Java -
import java.util.*; // The Main class public class Main { public static void main(String[] args) { // Creating two lists using List interface List < Integer > list1 = new ArrayList < Integer > (); List < Integer > list2 = new ArrayList < Integer > (); // Adding elements to list 1 list1.add(0, 10); list1.add(1, 20); // Printing list 1 System.out.println("list1 : " + list1); // Adding elements to list 2 list2.add(10); list2.add(20); list2.add(30); // Adding all elements of list 2 in list 1 list1.addAll(1, list2); // Printing list 2 System.out.println("list2 : " + list2); // Removes an element from list 1 (from index 1) list1.remove(1); // Printing list 1 System.out.println("list1 after removing an element: " + list1); // get() method System.out.println("list1 using get() : " + list1.get(2)); // Replacing element list1.set(0, 50); // Printing the list 1 System.out.println("list1 : " + list1); } }
Output
list1 : [10, 20] list2 : [10, 20, 30] list1 after removing an element: [10, 20, 30, 20] list1 using get() : 30 list1 : [50, 20, 30, 20]
List Interface Methods
The following are the methods of List Interface in Java -
Sr.No. | Method & Description |
---|---|
1 | void add(int index, Object obj) Inserts obj into the invoking list at the index passed in the index. Any pre-existing elements at or beyond the point of insertion are shifted up. Thus, no elements are overwritten. |
2 | boolean addAll(int index, Collection c) Inserts all elements of c into the invoking list at the index passed in the index. Any pre-existing elements at or beyond the point of insertion are shifted up. Thus, no elements are overwritten. Returns true if the invoking list changes and returns false otherwise. |
3 | Object get(int index) Returns the object stored at the specified index within the invoking collection. |
4 | int indexOf(Object obj) Returns the index of the first instance of obj in the invoking list. If obj is not an element of the list, .1 is returned. |
5 | int lastIndexOf(Object obj) Returns the index of the last instance of obj in the invoking list. If obj is not an element of the list, .1 is returned. |
6 | ListIterator listIterator( ) Returns an iterator to the start of the invoking list. |
7 | ListIterator listIterator(int index) Returns an iterator to the invoking list that begins at the specified index. |
8 | Object remove(int index) Removes the element at position index from the invoking list and returns the deleted element. The resulting list is compacted. That is, the indexes of subsequent elements are decremented by one. |
9 | Object set(int index, Object obj) Assigns obj to the location specified by index within the invoking list. |
10 | List subList(int start, int end) Returns a list that includes elements from start to end.1 in the invoking list. Elements in the returned list are also referenced by the invoking object. |
More Examples of List Interface
Example: Java List using ArrayList
The above interface has been implemented using ArrayList. Following is the example to explain few methods from various class implementation of the above collection methods −
import java.util.ArrayList; import java.util.List; public class CollectionsDemo { public static void main(String[] args) { List<String> a1 = new ArrayList<>(); a1.add("Zara"); a1.add("Mahnaz"); a1.add("Ayan"); System.out.println(" ArrayList Elements"); System.out.print("\t" + a1); } }
Output
ArrayList Elements [Zara, Mahnaz, Ayan]
Example: Java List using LinkedList
The above interface has been implemented using LinkedList. Following is the example to explain few methods from various class implementation of the above collection methods −
import java.util.LinkedList; import java.util.List; public class CollectionsDemo { public static void main(String[] args) { List<String> a1 = new LinkedList<>(); a1.add("Zara"); a1.add("Mahnaz"); a1.add("Ayan"); System.out.println(" LinkedList Elements"); System.out.print("\t" + a1); } }
Output
LinkedList Elements [Zara, Mahnaz, Ayan]
Example: Adding Element to Java List
The above interface has been implemented using ArrayList. Following is another example to explain few methods from various class implementation of the above collection methods −
import java.util.ArrayList; import java.util.List; public class CollectionsDemo { public static void main(String[] args) { List<String> a1 = new ArrayList<>(); a1.add("Zara"); a1.add("Mahnaz"); a1.add("Ayan"); System.out.println(" ArrayList Elements"); System.out.print("\t" + a1); // remove second element a1.remove(1); System.out.println("\n ArrayList Elements"); System.out.print("\t" + a1); } }
Output
ArrayList Elements [Zara, Mahnaz, Ayan] ArrayList Elements [Zara, Ayan]