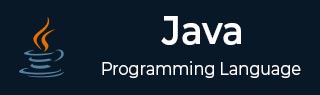
Java Tutorial
- Java - Home
- Java - Overview
- Java - History
- Java - Features
- Java vs C++
- Java Virtual Machine (JVM)
- Java - JDK vs JRE vs JVM
- Java - Hello World Program
- Java - Environment Setup
- Java - Basic Syntax
- Java - Variable Types
- Java - Data Types
- Java - Type Casting
- Java - Unicode System
- Java - Basic Operators
- Java - Comments
Java Control Statements
- Java - Loop Control
- Java - Decision Making
- Java - If-else
- Java - Switch
- Java - For Loops
- Java - For-Each Loops
- Java - While Loops
- Java - do-while Loops
- Java - Break
- Java - Continue
Object Oriented Programming
- Java - OOPs Concepts
- Java - Object & Classes
- Java - Class Attributes
- Java - Class Methods
- Java - Methods
- Java - Variables Scope
- Java - Constructors
- Java - Access Modifiers
- Java - Inheritance
- Java - Aggregation
- Java - Polymorphism
- Java - Overriding
- Java - Method Overloading
- Java - Dynamic Binding
- Java - Static Binding
- Java - Instance Initializer Block
- Java - Abstraction
- Java - Encapsulation
- Java - Interfaces
- Java - Packages
- Java - Inner Classes
- Java - Static Class
- Java - Anonymous Class
- Java - Singleton Class
- Java - Wrapper Classes
- Java - Enums
- Java - Enum Constructor
- Java - Enum Strings
Java Built-in Classes
Java File Handling
- Java - Files
- Java - Create a File
- Java - Write to File
- Java - Read Files
- Java - Delete Files
- Java - Directories
- Java - I/O Streams
Java Error & Exceptions
- Java - Exceptions
- Java - try-catch Block
- Java - try-with-resources
- Java - Multi-catch Block
- Java - Nested try Block
- Java - Finally Block
- Java - throw Exception
- Java - Exception Propagation
- Java - Built-in Exceptions
- Java - Custom Exception
Java Multithreading
- Java - Multithreading
- Java - Thread Life Cycle
- Java - Creating a Thread
- Java - Starting a Thread
- Java - Joining Threads
- Java - Naming Thread
- Java - Thread Scheduler
- Java - Thread Pools
- Java - Main Thread
- Java - Thread Priority
- Java - Daemon Threads
- Java - Thread Group
- Java - Shutdown Hook
Java Synchronization
- Java - Synchronization
- Java - Block Synchronization
- Java - Static Synchronization
- Java - Inter-thread Communication
- Java - Thread Deadlock
- Java - Interrupting a Thread
- Java - Thread Control
- Java - Reentrant Monitor
Java Networking
- Java - Networking
- Java - Socket Programming
- Java - URL Processing
- Java - URL Class
- Java - URLConnection Class
- Java - HttpURLConnection Class
- Java - Socket Class
- Java - Generics
Java Collections
Java Interfaces
- Java - List Interface
- Java - Queue Interface
- Java - Map Interface
- Java - SortedMap Interface
- Java - Set Interface
- Java - SortedSet Interface
Java Data Structures
Java Collections Algorithms
Advanced Java
- Java - Command-Line Arguments
- Java - Lambda Expressions
- Java - Sending Email
- Java - Applet Basics
- Java - Javadoc Comments
- Java - Autoboxing and Unboxing
- Java - File Mismatch Method
- Java - REPL (JShell)
- Java - Multi-Release Jar Files
- Java - Private Interface Methods
- Java - Inner Class Diamond Operator
- Java - Multiresolution Image API
- Java - Collection Factory Methods
- Java - Module System
- Java - Nashorn JavaScript
- Java - Optional Class
- Java - Method References
- Java - Functional Interfaces
- Java - Default Methods
- Java - Base64 Encode Decode
- Java - Switch Expressions
- Java - Teeing Collectors
- Java - Microbenchmark
- Java - Text Blocks
- Java - Dynamic CDS archive
- Java - Z Garbage Collector (ZGC)
- Java - Null Pointer Exception
- Java - Packaging Tools
- Java - Sealed Classes
- Java - Record Classes
- Java - Hidden Classes
- Java - Pattern Matching
- Java - Compact Number Formatting
- Java - Garbage Collection
- Java - JIT Compiler
Java Miscellaneous
- Java - Recursion
- Java - Regular Expressions
- Java - Serialization
- Java - Strings
- Java - Process API Improvements
- Java - Stream API Improvements
- Java - Enhanced @Deprecated Annotation
- Java - CompletableFuture API Improvements
- Java - Streams
- Java - Datetime Api
- Java 8 - New Features
- Java 9 - New Features
- Java 10 - New Features
- Java 11 - New Features
- Java 12 - New Features
- Java 13 - New Features
- Java 14 - New Features
- Java 15 - New Features
- Java 16 - New Features
Java APIs & Frameworks
Java Class References
- Java - Scanner Class
- Java - Arrays Class
- Java - Strings
- Java - Date & Time
- Java - ArrayList
- Java - Vector Class
- Java - Stack Class
- Java - PriorityQueue
- Java - LinkedList
- Java - ArrayDeque
- Java - HashMap
- Java - LinkedHashMap
- Java - WeakHashMap
- Java - EnumMap
- Java - TreeMap
- Java - The IdentityHashMap Class
- Java - HashSet
- Java - EnumSet
- Java - LinkedHashSet
- Java - TreeSet
- Java - BitSet Class
- Java - Dictionary
- Java - Hashtable
- Java - Properties
- Java - Collection Interface
- Java - Array Methods
Java Useful Resources
Java - ThreadGroup Class
ThreadGroup Class
The Java ThreadGroup class represents a set of threads. It can also include other thread groups. The thread groups form a tree in which every thread group except the initial thread group has a parent.
ThreadGroup Class Declaration
Following is the declaration for java.lang.ThreadGroup class −
public class ThreadGroup extends Object implements Thread.UncaughtExceptionHandler
ThreadGroup Class Constructors
Sr.No. | Constructor & Description |
---|---|
1 |
ThreadGroup(String name) This constructs a new thread group. |
2 |
ThreadGroup(ThreadGroup parent, String name) This creates a new thread group. |
ThreadGroup Class Methods
Sr.No. | Method & Description |
---|---|
1 | int activeCount()
This method returns an estimate of the number of active threads in this thread group. |
2 | int activeGroupCount()
This method returns an estimate of the number of active groups in this thread group. |
3 | void checkAccess()
This method determines if the currently running thread has permission to modify this thread group. |
4 | void destroy()
This method Destroys this thread group and all of its subgroups. |
5 | int enumerate(Thread[] list)
This method copies into the specified array every active thread in this thread group and its subgroups. |
6 | int enumerate(Thread[] list, boolean recurse)
This method copies into the specified array every active thread in this thread group. |
7 | int enumerate(ThreadGroup[] list)
This method copies into the specified array references to every active subgroup in this thread group. |
8 | int enumerate(ThreadGroup[] list, boolean recurse)
This method copies into the specified array references to every active subgroup in this thread group. |
9 | int getMaxPriority()
This method returns the maximum priority of this thread group. |
10 | String getName()
This method returns the name of this thread group. |
11 | ThreadGroup getParent()
This method returns the parent of this thread group. |
12 | void interrupt()
This method interrupts all threads in this thread group. |
13 | boolean isDaemon()
This method Tests if this thread group is a daemon thread group. |
14 | boolean isDestroyed()
This method tests if this thread group has been destroyed. |
15 | void list()
This method prints information about this thread group to the standard output. |
16 | boolean parentOf(ThreadGroup g)
This method tests if this thread group is either the thread group argument or one of its ancestor thread groups. |
17 | void setDaemon(boolean daemon)
This method changes the daemon status of this thread group. |
18 | void setMaxPriority(int pri)
This method sets the maximum priority of the group. |
19 | String toString()
This method returns a string representation of this Thread group. |
20 | void uncaughtException(Thread t, Throwable e)
This method called by the Java Virtual Machine when a thread in this thread group stops because of an uncaught exception, and the thread does not have a specific Thread.UncaughtExceptionHandler installed. |
Methods Inherited
This class inherits methods from the following classes −
- java.lang.Object
Example of ThreadGroup Class in Java
Following example showcases the usage of ThreadGroup in a multithreaded program.
package com.tutorialspoint; public class ThreadGroupDemo implements Runnable { public static void main(String[] args) { ThreadGroupDemo tg = new ThreadGroupDemo(); tg.func(); } public void func() { try { // create a parent ThreadGroup ThreadGroup pGroup = new ThreadGroup("Parent ThreadGroup"); // create a child ThreadGroup for parent ThreadGroup ThreadGroup cGroup = new ThreadGroup(pGroup, "Child ThreadGroup"); // create a thread Thread t1 = new Thread(pGroup, this); System.out.println("Starting " + t1.getName() + "..."); t1.start(); // create another thread Thread t2 = new Thread(cGroup, this); System.out.println("Starting " + t2.getName() + "..."); t2.start(); // display the number of active threads System.out.println("Active threads in \"" + pGroup.getName() + "\" = " + pGroup.activeCount()); // block until the other threads finish t1.join(); t2.join(); } catch (InterruptedException ex) { System.out.println(ex.toString()); } } // implements run() public void run() { for(int i = 0;i < 1000;i++) { i++; } System.out.println(Thread.currentThread().getName() + " finished executing."); } }
Output
Let us compile and run the above program, this will produce the following result −
Starting Thread-0... Starting Thread-1... Thread-0 finished executing. Active threads in "Parent ThreadGroup" = 1 Thread-1 finished executing.