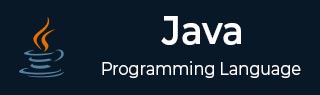
Java Tutorial
- Java - Home
- Java - Overview
- Java - History
- Java - Features
- Java vs C++
- Java Virtual Machine (JVM)
- Java - JDK vs JRE vs JVM
- Java - Hello World Program
- Java - Environment Setup
- Java - Basic Syntax
- Java - Variable Types
- Java - Data Types
- Java - Type Casting
- Java - Unicode System
- Java - Basic Operators
- Java - Comments
Java Control Statements
- Java - Loop Control
- Java - Decision Making
- Java - If-else
- Java - Switch
- Java - For Loops
- Java - For-Each Loops
- Java - While Loops
- Java - do-while Loops
- Java - Break
- Java - Continue
Object Oriented Programming
- Java - OOPs Concepts
- Java - Object & Classes
- Java - Class Attributes
- Java - Class Methods
- Java - Methods
- Java - Variables Scope
- Java - Constructors
- Java - Access Modifiers
- Java - Inheritance
- Java - Aggregation
- Java - Polymorphism
- Java - Overriding
- Java - Method Overloading
- Java - Dynamic Binding
- Java - Static Binding
- Java - Instance Initializer Block
- Java - Abstraction
- Java - Encapsulation
- Java - Interfaces
- Java - Packages
- Java - Inner Classes
- Java - Static Class
- Java - Anonymous Class
- Java - Singleton Class
- Java - Wrapper Classes
- Java - Enums
- Java - Enum Constructor
- Java - Enum Strings
Java Built-in Classes
Java File Handling
- Java - Files
- Java - Create a File
- Java - Write to File
- Java - Read Files
- Java - Delete Files
- Java - Directories
- Java - I/O Streams
Java Error & Exceptions
- Java - Exceptions
- Java - try-catch Block
- Java - try-with-resources
- Java - Multi-catch Block
- Java - Nested try Block
- Java - Finally Block
- Java - throw Exception
- Java - Exception Propagation
- Java - Built-in Exceptions
- Java - Custom Exception
Java Multithreading
- Java - Multithreading
- Java - Thread Life Cycle
- Java - Creating a Thread
- Java - Starting a Thread
- Java - Joining Threads
- Java - Naming Thread
- Java - Thread Scheduler
- Java - Thread Pools
- Java - Main Thread
- Java - Thread Priority
- Java - Daemon Threads
- Java - Thread Group
- Java - Shutdown Hook
Java Synchronization
- Java - Synchronization
- Java - Block Synchronization
- Java - Static Synchronization
- Java - Inter-thread Communication
- Java - Thread Deadlock
- Java - Interrupting a Thread
- Java - Thread Control
- Java - Reentrant Monitor
Java Networking
- Java - Networking
- Java - Socket Programming
- Java - URL Processing
- Java - URL Class
- Java - URLConnection Class
- Java - HttpURLConnection Class
- Java - Socket Class
- Java - Generics
Java Collections
Java Interfaces
- Java - List Interface
- Java - Queue Interface
- Java - Map Interface
- Java - SortedMap Interface
- Java - Set Interface
- Java - SortedSet Interface
Java Data Structures
Java Collections Algorithms
Advanced Java
- Java - Command-Line Arguments
- Java - Lambda Expressions
- Java - Sending Email
- Java - Applet Basics
- Java - Javadoc Comments
- Java - Autoboxing and Unboxing
- Java - File Mismatch Method
- Java - REPL (JShell)
- Java - Multi-Release Jar Files
- Java - Private Interface Methods
- Java - Inner Class Diamond Operator
- Java - Multiresolution Image API
- Java - Collection Factory Methods
- Java - Module System
- Java - Nashorn JavaScript
- Java - Optional Class
- Java - Method References
- Java - Functional Interfaces
- Java - Default Methods
- Java - Base64 Encode Decode
- Java - Switch Expressions
- Java - Teeing Collectors
- Java - Microbenchmark
- Java - Text Blocks
- Java - Dynamic CDS archive
- Java - Z Garbage Collector (ZGC)
- Java - Null Pointer Exception
- Java - Packaging Tools
- Java - Sealed Classes
- Java - Record Classes
- Java - Hidden Classes
- Java - Pattern Matching
- Java - Compact Number Formatting
- Java - Garbage Collection
- Java - JIT Compiler
Java Miscellaneous
- Java - Recursion
- Java - Regular Expressions
- Java - Serialization
- Java - Strings
- Java - Process API Improvements
- Java - Stream API Improvements
- Java - Enhanced @Deprecated Annotation
- Java - CompletableFuture API Improvements
- Java - Streams
- Java - Datetime Api
- Java 8 - New Features
- Java 9 - New Features
- Java 10 - New Features
- Java 11 - New Features
- Java 12 - New Features
- Java 13 - New Features
- Java 14 - New Features
- Java 15 - New Features
- Java 16 - New Features
Java APIs & Frameworks
Java Class References
- Java - Scanner Class
- Java - Arrays Class
- Java - Strings
- Java - Date & Time
- Java - ArrayList
- Java - Vector Class
- Java - Stack Class
- Java - PriorityQueue
- Java - LinkedList
- Java - ArrayDeque
- Java - HashMap
- Java - LinkedHashMap
- Java - WeakHashMap
- Java - EnumMap
- Java - TreeMap
- Java - The IdentityHashMap Class
- Java - HashSet
- Java - EnumSet
- Java - LinkedHashSet
- Java - TreeSet
- Java - BitSet Class
- Java - Dictionary
- Java - Hashtable
- Java - Properties
- Java - Collection Interface
- Java - Array Methods
Java Useful Resources
Java - Constructors
Java Constructors
Java constructors are special types of methods that are used to initialize an object when it is created. It has the same name as its class and is syntactically similar to a method. However, constructors have no explicit return type.
Typically, you will use a constructor to give initial values to the instance variables defined by the class or to perform any other start-up procedures required to create a fully formed object.
All classes have constructors, whether you define one or not because Java automatically provides a default constructor that initializes all member variables to zero. However, once you define your constructor, the default constructor is no longer used.
Rules for Creating Java Constructors
You must follow the below-given rules while creating Java constructors:
- The name of the constructors must be the same as the class name.
- Java constructors do not have a return type. Even do not use void as a return type.
- There can be multiple constructors in the same class, this concept is known as constructor overloading.
- The access modifiers can be used with the constructors, use if you want to change the visibility/accessibility of constructors.
- Java provides a default constructor that is invoked during the time of object creation. If you create any type of constructor, the default constructor (provided by Java) is not invoked.
Creating a Java Constructor
To create a constructor in Java, simply write the constructor's name (that is the same as the class name) followed by the brackets and then write the constructor's body inside the curly braces ({}).
Syntax
Following is the syntax of a constructor −
class ClassName { ClassName() { } }
Example to create a Java Constructor
The following example creates a simple constructor that will print "Hello world".
public class Main { // Creating a constructor Main() { System.out.println("Hello, World!"); } public static void main(String[] args) { System.out.println("The main() method."); // Creating a class's object // that will invoke the constructor Main obj_x = new Main(); } }
This program will print:
The main() method. Hello, World!
Types of Java Constructors
There are three different types of constructors in Java, we have listed them as follows:
- Default Constructor
- No-Args Constructor
- Parameterized Constructor

1. Default Constructor
If you do not create any constructor in the class, Java provides a default constructor that initializes the object.
Example: Default Constructor (A Class Without Any Constructor)
In this example, there is no constructor defined by us. The default constructor is there to initialize the object.
public class Main { int num1; int num2; public static void main(String[] args) { // We didn't created any structure // a default constructor will invoke here Main obj_x = new Main(); // Printing the values System.out.println("num1 : " + obj_x.num1); System.out.println("num2 : " + obj_x.num2); } }
Output
num1 : 0 num2 : 0
2. No-Args (No Argument) Constructor
As the name specifies, the No-argument constructor does not accept any argument. By using the No-Args constructor you can initialize the class data members and perform various activities that you want on object creation.
Example: No-Args Constructor
This example creates no-args constructor.
public class Main { int num1; int num2; // Creating no-args constructor Main() { num1 = -1; num2 = -1; } public static void main(String[] args) { // no-args constructor will invoke Main obj_x = new Main(); // Printing the values System.out.println("num1 : " + obj_x.num1); System.out.println("num2 : " + obj_x.num2); } }
Output
num1 : -1 num2 : -1
3. Parameterized Constructor
A constructor with one or more arguments is called a parameterized constructor.
Most often, you will need a constructor that accepts one or more parameters. Parameters are added to a constructor in the same way that they are added to a method, just declare them inside the parentheses after the constructor's name.
Example 1: Parameterized Constructor
This example creates a parameterized constructor.
public class Main { int num1; int num2; // Creating parameterized constructor Main(int a, int b) { num1 = a; num2 = b; } public static void main(String[] args) { // Creating two objects by passing the values // to initialize the attributes. // parameterized constructor will invoke Main obj_x = new Main(10, 20); Main obj_y = new Main(100, 200); // Printing the objects values System.out.println("obj_x"); System.out.println("num1 : " + obj_x.num1); System.out.println("num2 : " + obj_x.num2); System.out.println("obj_y"); System.out.println("num1 : " + obj_y.num1); System.out.println("num2 : " + obj_y.num2); } }
Output
obj_x num1 : 10 num2 : 20 obj_y num1 : 100 num2 : 200
Example 2: Parameterized Constructor
Here is a simple example that uses a constructor −
// A simple constructor. class MyClass { int x; // Following is the constructor MyClass(int i ) { x = i; } }
You would call constructor to initialize objects as follows −
public class ConsDemo { public static void main(String args[]) { MyClass t1 = new MyClass( 10 ); MyClass t2 = new MyClass( 20 ); System.out.println(t1.x + " " + t2.x); } }
Output
10 20
Constructor Overloading in Java
Constructor overloading means multiple constructors in a class. When you have multiple constructors with different parameters listed, then it will be known as constructor overloading.
Example: Constructor Overloading
In this example, we have more than one constructor.
// Example of Java Constructor Overloading // Creating a Student Class class Student { String name; int age; // no-args constructor Student() { this.name = "Unknown"; this.age = 0; } // parameterized constructor having one parameter Student(String name) { this.name = name; this.age = 0; } // parameterized constructor having both parameters Student(String name, int age) { this.name = name; this.age = age; } public void printDetails() { System.out.println("Name : " + this.name); System.out.println("Age : " + this.age); } } public class Main { public static void main(String[] args) { Student std1 = new Student(); // invokes no-args constructor Student std2 = new Student("Jordan"); // invokes parameterized constructor Student std3 = new Student("Paxton", 25); // invokes parameterized constructor // Printing details System.out.println("std1..."); std1.printDetails(); System.out.println("std2..."); std2.printDetails(); System.out.println("std3..."); std3.printDetails(); } }
Output
td1... Name : Unknown Age : 0 std2... Name : Jordan Age : 0 std3... Name : Paxton Age : 25