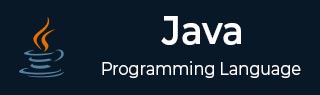
Java Tutorial
- Java - Home
- Java - Overview
- Java - History
- Java - Features
- Java vs C++
- Java Virtual Machine (JVM)
- Java - JDK vs JRE vs JVM
- Java - Hello World Program
- Java - Environment Setup
- Java - Basic Syntax
- Java - Variable Types
- Java - Data Types
- Java - Type Casting
- Java - Unicode System
- Java - Basic Operators
- Java - Comments
Java Control Statements
- Java - Loop Control
- Java - Decision Making
- Java - If-else
- Java - Switch
- Java - For Loops
- Java - For-Each Loops
- Java - While Loops
- Java - do-while Loops
- Java - Break
- Java - Continue
Object Oriented Programming
- Java - OOPs Concepts
- Java - Object & Classes
- Java - Class Attributes
- Java - Class Methods
- Java - Methods
- Java - Variables Scope
- Java - Constructors
- Java - Access Modifiers
- Java - Inheritance
- Java - Aggregation
- Java - Polymorphism
- Java - Overriding
- Java - Method Overloading
- Java - Dynamic Binding
- Java - Static Binding
- Java - Instance Initializer Block
- Java - Abstraction
- Java - Encapsulation
- Java - Interfaces
- Java - Packages
- Java - Inner Classes
- Java - Static Class
- Java - Anonymous Class
- Java - Singleton Class
- Java - Wrapper Classes
- Java - Enums
- Java - Enum Constructor
- Java - Enum Strings
Java Built-in Classes
Java File Handling
- Java - Files
- Java - Create a File
- Java - Write to File
- Java - Read Files
- Java - Delete Files
- Java - Directories
- Java - I/O Streams
Java Error & Exceptions
- Java - Exceptions
- Java - try-catch Block
- Java - try-with-resources
- Java - Multi-catch Block
- Java - Nested try Block
- Java - Finally Block
- Java - throw Exception
- Java - Exception Propagation
- Java - Built-in Exceptions
- Java - Custom Exception
Java Multithreading
- Java - Multithreading
- Java - Thread Life Cycle
- Java - Creating a Thread
- Java - Starting a Thread
- Java - Joining Threads
- Java - Naming Thread
- Java - Thread Scheduler
- Java - Thread Pools
- Java - Main Thread
- Java - Thread Priority
- Java - Daemon Threads
- Java - Thread Group
- Java - Shutdown Hook
Java Synchronization
- Java - Synchronization
- Java - Block Synchronization
- Java - Static Synchronization
- Java - Inter-thread Communication
- Java - Thread Deadlock
- Java - Interrupting a Thread
- Java - Thread Control
- Java - Reentrant Monitor
Java Networking
- Java - Networking
- Java - Socket Programming
- Java - URL Processing
- Java - URL Class
- Java - URLConnection Class
- Java - HttpURLConnection Class
- Java - Socket Class
- Java - Generics
Java Collections
Java Interfaces
- Java - List Interface
- Java - Queue Interface
- Java - Map Interface
- Java - SortedMap Interface
- Java - Set Interface
- Java - SortedSet Interface
Java Data Structures
Java Collections Algorithms
Advanced Java
- Java - Command-Line Arguments
- Java - Lambda Expressions
- Java - Sending Email
- Java - Applet Basics
- Java - Javadoc Comments
- Java - Autoboxing and Unboxing
- Java - File Mismatch Method
- Java - REPL (JShell)
- Java - Multi-Release Jar Files
- Java - Private Interface Methods
- Java - Inner Class Diamond Operator
- Java - Multiresolution Image API
- Java - Collection Factory Methods
- Java - Module System
- Java - Nashorn JavaScript
- Java - Optional Class
- Java - Method References
- Java - Functional Interfaces
- Java - Default Methods
- Java - Base64 Encode Decode
- Java - Switch Expressions
- Java - Teeing Collectors
- Java - Microbenchmark
- Java - Text Blocks
- Java - Dynamic CDS archive
- Java - Z Garbage Collector (ZGC)
- Java - Null Pointer Exception
- Java - Packaging Tools
- Java - Sealed Classes
- Java - Record Classes
- Java - Hidden Classes
- Java - Pattern Matching
- Java - Compact Number Formatting
- Java - Garbage Collection
- Java - JIT Compiler
Java Miscellaneous
- Java - Recursion
- Java - Regular Expressions
- Java - Serialization
- Java - Strings
- Java - Process API Improvements
- Java - Stream API Improvements
- Java - Enhanced @Deprecated Annotation
- Java - CompletableFuture API Improvements
- Java - Streams
- Java - Datetime Api
- Java 8 - New Features
- Java 9 - New Features
- Java 10 - New Features
- Java 11 - New Features
- Java 12 - New Features
- Java 13 - New Features
- Java 14 - New Features
- Java 15 - New Features
- Java 16 - New Features
Java APIs & Frameworks
Java Class References
- Java - Scanner Class
- Java - Arrays Class
- Java - Strings
- Java - Date & Time
- Java - ArrayList
- Java - Vector Class
- Java - Stack Class
- Java - PriorityQueue
- Java - LinkedList
- Java - ArrayDeque
- Java - HashMap
- Java - LinkedHashMap
- Java - WeakHashMap
- Java - EnumMap
- Java - TreeMap
- Java - The IdentityHashMap Class
- Java - HashSet
- Java - EnumSet
- Java - LinkedHashSet
- Java - TreeSet
- Java - BitSet Class
- Java - Dictionary
- Java - Hashtable
- Java - Properties
- Java - Collection Interface
- Java - Array Methods
Java Useful Resources
Java - Aggregation
Java Aggregation
An aggregation is a relationship between two classes where one class contains an instance of another class. For example, when an object A contains a reference to another object B or we can say Object A has a HAS-A relationship with Object B, then it is termed as Aggregation in Java Programming.
Use of Java Aggregation
Aggregation in Java helps in reusing the code. Object B can have utility methods and which can be utilized by multiple objects. Whichever class has object B then it can utilize its methods.
Java Aggregation Examples
Example 1
In this example, we're creating few classes like Vehicle, Speed. A Van class is defined which extends Vehicle class and has a Speed class object. Van class inherits properties from Vehicle class and Speed being its property, we're passing it from caller object. In output, we're printing the details of Van object.
package com.tutorialspoint; class Vehicle{ private String vin; public String getVin() { return vin; } public void setVin(String vin) { this.vin = vin; } } class Speed{ private double max; public double getMax() { return max; } public void setMax(double max) { this.max = max; } } class Van extends Vehicle { private Speed speed; public Speed getSpeed() { return speed; } public void setSpeed(Speed speed) { this.speed = speed; } public void print() { System.out.println("Vin: " +this.getVin() + ", Max Speed: " + speed.getMax() ); } } public class Tester { public static void main(String[] args) { Speed speed = new Speed(); speed.setMax(120); Van van = new Van(); van.setVin("abcd1233"); van.setSpeed(speed); van.print(); } }
Output
Vin: abcd1233, Max Speed: 120.0
Example 2
In this example, we're creating few classes like Student, Address. A student can has a address. So we've defined an address as an instance variable in Student class. In output, we're printing the student details.
package com.tutorialspoint; class Address { int strNum; String city; String state; String country; Address(int street, String c, String st, String country) { this.strNum = street; this.city = c; this.state = st; this.country = country; } } class Student { int rno; String stName; Address stAddr; Student(int roll, String name, Address address){ this.rno = roll; this.stName = name; this.stAddr = address; } } public class Tester { public static void main(String[] args) { Address ad= new Address(10, "Bareilly", "UP", "India"); Student st= new Student(1, "Aashi", ad); System.out.println("Roll no: "+ st.rno); System.out.println("Name: "+ st.stName); System.out.println("Street: "+ st.stAddr.strNum); System.out.println("City: "+ st.stAddr.city); System.out.println("State: "+ st.stAddr.state); System.out.println("Country: "+ st.stAddr.country); } }
Output
Roll no: 1 Name: Aashi Street: 10 City: Bareilly State: UP Country: India
In a unique sense, this is a type of association. Aggregation is a one way directed relationship that precisely expresses HAS-A relationship between classes. Additionally, when two classes are aggregated, terminating one of them has no effect on the other. When compared to composition, it is frequently designated as a weak relationship. In comparison, the parent owns the child entity, which means the child entity cannot be accessed directly and cannot exist without the parent object. Contrarily, in an association, the parent and child entities may both exist in their own right.
HAS-A Relationship
These relationships are mainly based on the usage. This determines whether a certain class HAS-A certain thing. This relationship helps to reduce duplication of code as well as bugs.
Example
public class Vehicle{} public class Speed{} public class Van extends Vehicle { private Speed sp; }
This shows that class Van HAS-A Speed. By having a separate class for Speed, we do not have to put the entire code that belongs to speed inside the Van class, which makes it possible to reuse the Speed class in multiple applications.
In Object-Oriented feature, the users do not need to bother about which object is doing the real work. To achieve this, the Van class hides the implementation details from the users of the Van class. So, basically what happens is the users would ask the Van class to do a certain action and the Van class will either do the work by itself or ask another class to perform the action.