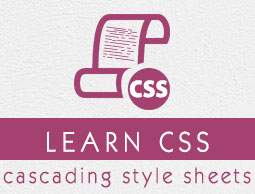
- CSS Tutorial
- CSS - Home
- CSS - Introduction
- CSS - Syntax
- CSS - Selectors
- CSS - Inclusion
- CSS - Measurement Units
- CSS - Colors
- CSS - Backgrounds
- CSS - Fonts
- CSS - Text
- CSS - Images
- CSS - Links
- CSS - Tables
- CSS - Borders
- CSS - Border Block
- CSS - Border Inline
- CSS - Margins
- CSS - Lists
- CSS - Padding
- CSS - Cursor
- CSS - Outlines
- CSS - Dimension
- CSS - Scrollbars
- CSS - Inline Block
- CSS - Dropdowns
- CSS - Visibility
- CSS - Overflow
- CSS - Clearfix
- CSS - Float
- CSS - Arrows
- CSS - Resize
- CSS - Quotes
- CSS - Order
- CSS - Position
- CSS - Hyphens
- CSS - Hover
- CSS - Display
- CSS - Focus
- CSS - Zoom
- CSS - Translate
- CSS - Height
- CSS - Hyphenate Character
- CSS - Width
- CSS - Opacity
- CSS - Z-Index
- CSS - Bottom
- CSS - Navbar
- CSS - Overlay
- CSS - Forms
- CSS - Align
- CSS - Icons
- CSS - Image Gallery
- CSS - Comments
- CSS - Loaders
- CSS - Attr Selectors
- CSS - Combinators
- CSS - Root
- CSS - Box Model
- CSS - Counters
- CSS - Clip
- CSS - Writing Mode
- CSS - Unicode-bidi
- CSS - min-content
- CSS - All
- CSS - Inset
- CSS - Isolation
- CSS - Overscroll
- CSS - Justify Items
- CSS - Justify Self
- CSS - Tab Size
- CSS - Pointer Events
- CSS - Place Content
- CSS - Place Items
- CSS - Place Self
- CSS - Max Block Size
- CSS - Min Block Size
- CSS - Mix Blend Mode
- CSS - Max Inline Size
- CSS - Min Inline Size
- CSS - Offset
- CSS - Accent Color
- CSS - User Select
- CSS Advanced
- CSS - Grid
- CSS - Grid Layout
- CSS - Flexbox
- CSS - Visibility
- CSS - Positioning
- CSS - Layers
- CSS - Pseudo Classes
- CSS - Pseudo Elements
- CSS - @ Rules
- CSS - Text Effects
- CSS - Paged Media
- CSS - Printing
- CSS - Layouts
- CSS - Validations
- CSS - Image Sprites
- CSS - Important
- CSS - Data Types
- CSS3 Tutorial
- CSS3 - Tutorial
- CSS - Rounded Corner
- CSS - Border Images
- CSS - Multi Background
- CSS - Color
- CSS - Gradients
- CSS - Box Shadow
- CSS - Box Decoration Break
- CSS - Caret Color
- CSS - Text Shadow
- CSS - Text
- CSS - 2d transform
- CSS - 3d transform
- CSS - Transition
- CSS - Animation
- CSS - Multi columns
- CSS - Box Sizing
- CSS - Tooltips
- CSS - Buttons
- CSS - Pagination
- CSS - Variables
- CSS - Media Queries
- CSS - Functions
- CSS - Math Functions
- CSS - Masking
- CSS - Shapes
- CSS - Style Images
- CSS - Specificity
- CSS - Custom Properties
- CSS Responsive
- CSS RWD - Introduction
- CSS RWD - Viewport
- CSS RWD - Grid View
- CSS RWD - Media Queries
- CSS RWD - Images
- CSS RWD - Videos
- CSS RWD - Frameworks
- CSS References
- CSS - Questions and Answers
- CSS - Quick Guide
- CSS - References
- CSS - Color References
- CSS - Web browser References
- CSS - Web safe fonts
- CSS - Units
- CSS - Animation
- CSS Resources
- CSS - Useful Resources
- CSS - Discussion
CSS - Selectors
CSS Selectors are used to select the HTML elements you want to style on a web page. They allow you to target specific elements or groups of elements to apply styles like colors, fonts, margins, and more.
The element or elements that are selected by the selector are referred to as subject of the selector.
Types of Selectors
CSS Universal Selector
Universal selector, denoted by an asterisk mark (*), is a special selector that matches all elements in an HTML document. These are generally used to add a same length margin and padding to all the elements in document.
Syntax
* { margin: 0; padding: 0; }
As per the above syntax, the universal selector is used to apply a margin and padding of 0 to all HTML elements.
Example
Following example demonstrates the use of a universal selector (*):
<html> <head> <style> * { margin: 0; padding: 0; background-color: peachpuff; color: darkgreen; font-size: 25px; } </style> </head> <body> <h1>Universal selector (*)</h1> <div> Parent element <p>Child paragraph 1</p> <p>Child paragraph 2</p> </div> <p>Paragraph 3</p> </body> </html>
CSS Element Selector
A element selector targets an HTML element, such as <h1>, <p>, etc. This is used when we want to apply similar style to all the <p> tags or <h1> tags in the document.
Syntax
/* Sets text color of all p tags to green */ p { color: green; } /* Add underline to all h1 tags in document */ h1 { text-decoration-line: underline; }
Example
Following example demonstrates the use of a element selector:
<html> <head> <style> div { border: 5px inset gold; width: 300px; text-align: center; } p { color: green; } h1 { text-decoration-line: underline; } </style> </head> <body> <div> <h1>Element selector</h1> <p>Div with border </p> <p>Text aligned to center</p> <p>Paragraph with green color</p> <p>h1 with an underline</p> </div> </body> </html>
CSS Class Selector
A class selector targets an element with a specific value for its class attribute to style it. A class in CSS is denoted by "." (period) symbol.
Syntax
.sideDiv { text-decoration-line: underline; } .topDiv { color: green; font-size: 25px; }
Example
Following example demonstrates the use of a class selector, where .style-div, .topDivs and .bottomDivs are class selectors:
<html> <head> <style> .style-div { border: 5px inset gold; width: 300px; text-align: center; } .topDivs{ font-weight: bold; font-size: 30px; } .bottomDivs{ color: green; font-size: 20px; } </style> </head> <body> <div class="style-div"> <div class="topDivs"> Hello World </div> <div class="topDivs"> Learn CSS </div> <div class="bottomDivs"> From </div> <div class="bottomDivs"> TutorialsPoint </div> </div> </body> </html>
CSS ID Selector
An ID selector targets single element with a particular value for id attribute to style it. An id in CSS is denoted by "#" (hash) symbol. Same class can be applied to multiple elements, but an id is unique for an element.
Syntax
#style-p { color: green; font-size: 25px; } #style-h1 { text-decoration-line: underline; color: red; }
Example
Following example demonstrates the use of an id selector, where #style-div, #tutorial and #stylePoint are the id selectors applied on the elements:
<html> <head> <style> #style-div { border: 5px inset gold; width: 300px; text-align: center; } #tutorial{ color: green; font-size: 20px; } #stylePoint{ color: black; font-size: 15px; font-weight: bold; } </style> </head> <body> <div id="style-div"> <div id="tutorial"> Tutorials <span id="stylePoint"> Point </span> </div> <p> Here we used ids to style different elements. </p> </div> </body> </html>
CSS Attribute Selector
An attribute selector targets an element based on a specific attribute or attribute values on an element.
For a detailed explanation of attribute selectors, refer this attribute selector article.
Syntax
/* Style all anchor tag with target attribute */ a[target] { background-color: peachpuff; } /* Style all anchor tag that links to tutorialspoint */ a[href="https://www.tutorialspoint.com"] { background-color: peachpuff; }
Example
Following example demonstrates the use of an attribute selector:
<html> <head> <style> a[href]{ font-size: 2em; } a[target] { background-color: peachpuff; color: blueviolet; } /* Attribute with value have more priority*/ /* Hence black background applied to CSS link*/ a[target="_self"] { background-color: black; } </style> </head> <body> <h2>Attribute selector</h2> <p> Styling applied to anchor element: </p> <a href="https://www.tutorialspoint.com/"> Tutorialspoint </a> <br><br> <a href="/html/index.htm" target="_blank"> HTML Tutorial </a> <br><br> <a href="/css/index.htm" target="_self"> CSS Tutorial </a> </body> </html>
CSS Group Selector
CSS group selector allow us to apply same style to multiple elements at a time. Name of elements can be separated by commas. This method is recommended as it keep CSS concise and avoid redundancy.
Syntax
/* Apply same background color for h1 and h2 */ h1, h2 { background-color: grey; }
Example
Following example shows how to use group selectors in CSS.
<html> <head> <style> /* This applies to both <h1> and <h2> elements */ h1, h2 { background-color: grey; padding: 4px; } /*Applies to all paragraphs, elements with class*/ /*'highlight', and element with ID 'hightlightSpan'*/ p, .highlight, #hightlightSpan { background-color: yellow; padding: 10px; } </style> </head> <body> <h1>CSS Selectors</h1> <h2>Group Selectors</h2> <p>This is a paragraph.</p> <div class="highlight"> This is div </div> <br> <span id="hightlightSpan"> This is span </span> </body> </html>
CSS Pseudo Class Selector
A pseudo-class selector is used to style a specific state of an element, such as :hover is used to style an element when hovered.
For a detailed list of pseudo-class selectors, refer this CSS Pseudo Classes tutorial.
Syntax
/* Change background color on hover */ a :hover { background-color: peachpuff; } /* Change background color on clicking button */ button:active { background-color: yellow; } /* Change border color on focusing input */ input:focus { border-color: blue; }
Example
Following example demonstrates the use of a pseudo-class selector:
<html> <head> <style> a:hover { background-color: peachpuff; color: green; font-size: 2em; } button:active { background-color: yellow; } </style> </head> <body> <h2>Pseudo-class selector</h2> <p> Styling applied to anchor element and button with a pseudo-class: </p> <a href="https://www.tutorialspoint.com"> Tutorialspoint </a> <br><br> <button>Click Me</button> </body> </html>
CSS Pseudo Element Selector
A pseudo-element selector is used to style a specific part of an element rather than the element itself.
For a detailed list of pseudo-element selectors, refer this CSS Pseudo elements tutorial.
Syntax
/* Define contents before paragraph */ a::before { content: " "; } /* Style first letter of paragraph */ p::first-letter { font-size: 2em; }
Example
Following example demonstrates the use of a pseudo-element selector (::before) and (::after):
<html> <head> <style> /* Add and style contents before paragraph */ p::before { content: "Note: "; font-weight: bold; color: red; } /* Add and style contents after paragraph */ p::after { content: " [Read more]"; font-style: italic; color: blue; } </style> </head> <body> <h2>Pseudo-element selector</h2> <p>This is a paragraph.</p> </body> </html>
CSS Descendant Selector
Descendant selector is used in css to style all the tags which are child of a particular specified tag. A single space between parent element and child element is used to mention as descendant.
Syntax
div p { color: blue; }
The above code set text color of paragraph tags that are inside div element to blue.
Example
The following example show how to use descendant selector in css.
<!DOCTYPE html> <html lang="en"> <head> <style> div{ border: 2px solid; } div p { color: blue; } </style> </head> <body> <div> <p> This paragraph is inside a div and will be blue. </p> <section> <p> This paragraph is inside a section which is inside a div and will also be blue. </p> </section> </div> <p> This paragraph is outside the div and will not be blue. </p> </body> </html>
CSS Child Selector
The child selector in css is used to target all the direct child of a particular element. This is denoted by '>' (Greater than) symbol.
Syntax
div > p { color: blue; }
The above code set text color of paragraph tags that are directly inside div element to blue.
Example
The following example shows how to use child selector in css.
<!DOCTYPE html> <html lang="en"> <head> <style> div{ border: 2px solid; } div > p { color: blue; } </style> </head> <body> <div> <p> This paragraph is inside a div and will be blue. </p> <section> <p> This paragraph is inside a section which is inside a div and will not be blue as this is not direct child </p> </section> </div> <p> This paragraph is outside the div and will not be blue. </p> </body> </html>
CSS Adjacent Sibling Selectors
In CSS, adjacent sibling selector is used to target an element that is immediately preceded by a specified element. A plus symbol ( "+" ) is used to denote adjacent sibling.
Syntax
h1 + p { margin-top: 0; }
The above code sets top margin of paragraph tag just after h1 tag to 0.
Example
The following example shows how to use adjacent sibling selector in css.
<!DOCTYPE html> <html lang="en"> <head> <style> div{ border: 4px solid; } div + p { color: blue; } </style> </head> <body> <p> This paragraph is above the div and will not be blue </p> <div> <p> This paragraph is inside a div and will not be blue. </p> </div> <p> This paragraph 1 after the div and will be blue. </p> <p>This paragraph 2 after the div and will not be blue. </p> </body> </html>
CSS General Sibling Selector
In CSS, general sibling selector is used to target all the element that is preceded by a specified element. A tilde symbol ( "~" ) is used to denote general sibling.
Syntax
h1 ~ p { color: gray; }
The above code sets text color of all the paragraph after h1 tag to gray.
Example
The following example shows how to use general sibling selector in css.
<!DOCTYPE html> <html lang="en"> <head> <style> div{ border: 4px solid; } div ~ p { color: blue; } </style> </head> <body> <p> This paragraph is above the div and will not be blue </p> <div> <p> This paragraph is inside a div and will not be blue. </p> </div> <p> This paragraph 1 after the div and will be blue. </p> <p>This paragraph 2 after the div and will be blue. </p> </body> </html>
Nested Selectors In CSS
CSS nesting allows to nest one style rule inside another rule, with the selector of the child rule relative to the selector of the parent rule.
Characteristics of CSS nesting Selectors
The nesting selector shows the relationship between the parent and child rules.
- When the nested selectors are parsed by the browser, it automatically adds a whitespace between the selectors, thus creating a new CSS selector rule.
- In situations where the nested rule needs to be attached to the parent rule (without any whitespace), like while using the pseudo-class or compound selectors, the & nesting selector must be prepended immediately to achieve the desired result.
- In order to reverse the context of rules, the & nesting selector can be appended.
- There can be multiple instances of & nesting selector.
Syntax
nav { & ul { list-style: none; & li { display: inline-block; & a { text-decoration: none; color: blue; &:hover { color: red; } } } } }
Example
Following example demonstrates the use of a & nesting selector (&):
<html> <head> <style> #sample { font-family: Verdana, Geneva, Tahoma, sans-serif; font-size: 1.5rem; & a { color: crimson; &:hover, &:focus { color: green; background-color: yellow; } } } </style> </head> <body> <h1>& nesting selector</h1> <p id="sample"> Hover <a href="#">over the link</a>. </p> </body> </html>